In this Typescript tutorial, I will explain everything about “typescript enum reverse mapping”, like what reverse mapping is, how it works in TypeScript, and various methods to utilize it effectively.
To reverse map a TypeScript enum, access the enum member using its numeric value. For example, with enum StatusCode { Success = 1, Failure }
, you can get the name ‘Success’ using StatusCode[1]
. Note that automatic reverse mapping works only with numeric enums; string enums require a custom implementation.
What is Typescript Enum Reverse Mapping?
Enum (enumeration) in TypeScript gives more friendly names to sets of numeric values. Reverse mapping is an automatic feature of TypeScript enums, where it creates a bidirectional map, allowing you to access the enum member both by its name and its value.
For example:
enum StatusCode {
Success = 1,
Failure,
Unknown
}
let statusName: string = StatusCode[1];
let statusCode: number = StatusCode.Success;
In the above code, StatusCode[1]
returns the name of the enum member whose value is 1, which is ‘Success’.
Typescript enum reverse mapping
Here are more advanced and practical uses of reverse mapping in Typescript.
Method 1: Using Reverse Mapping for Error Codes
Consider an application where you need to handle various error codes and display corresponding messages.
enum ErrorCode {
NotFound = 404,
Forbidden = 403,
BadRequest = 400
}
function getErrorMessage(code: ErrorCode): string {
return `Error ${code}: ${ErrorCode[code]}`;
}
let message = getErrorMessage(ErrorCode.NotFound);
Method 2: Enums with String Values
Enums don’t just have to be numeric. You can also use strings, but this changes how reverse mapping works.
enum FileExtension {
JSON = 'json',
CSV = 'csv'
}
let ext: string = FileExtension.JSON; // 'json'
let extEnum: string = FileExtension['json'];
For string enums, TypeScript does not create a reverse map. You have to implement it manually if needed.
You can see after I ran the code using VS code, it gave me an error as “error TS2551: Property ‘json’ does not exist on type ‘typeof FileExtension’. Did you mean ‘JSON’?”.
Check out the screenshot below:
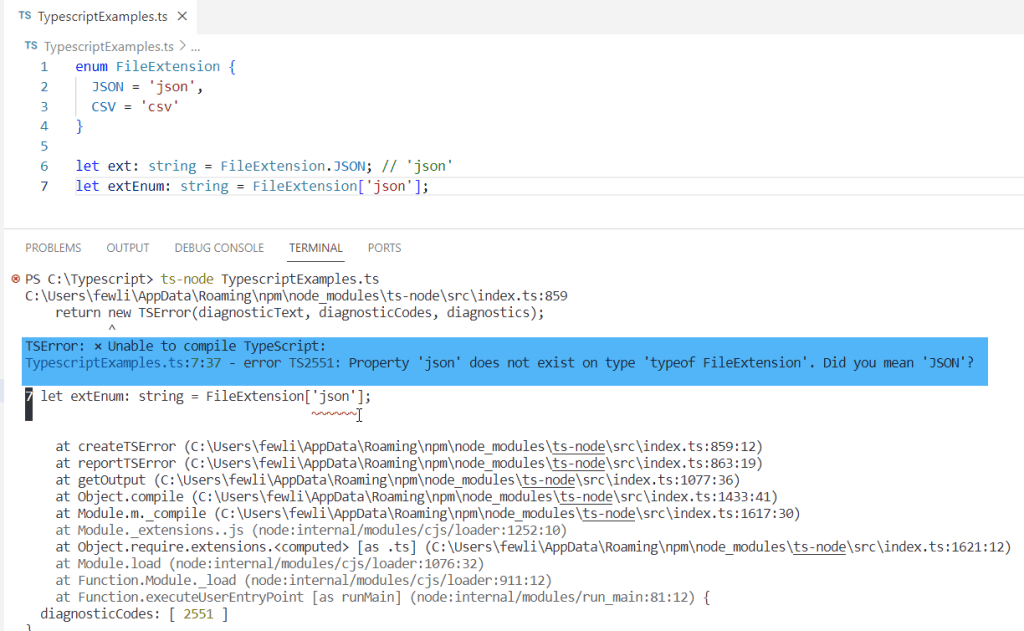
Method 3: Manual Reverse Mapping for String Enums
You can create a function to manually reverse-map string enums in Typescript. Here is a complete code:
enum FileExtension {
JSON = 'json',
CSV = 'csv'
}
function reverseMap(enumObj: any, value: string) {
return Object.keys(enumObj).find(key => enumObj[key] === value);
}
let extension = reverseMap(FileExtension, 'json');
console.log(extension);
Once you run the code, you can see the output in the screenshot below:
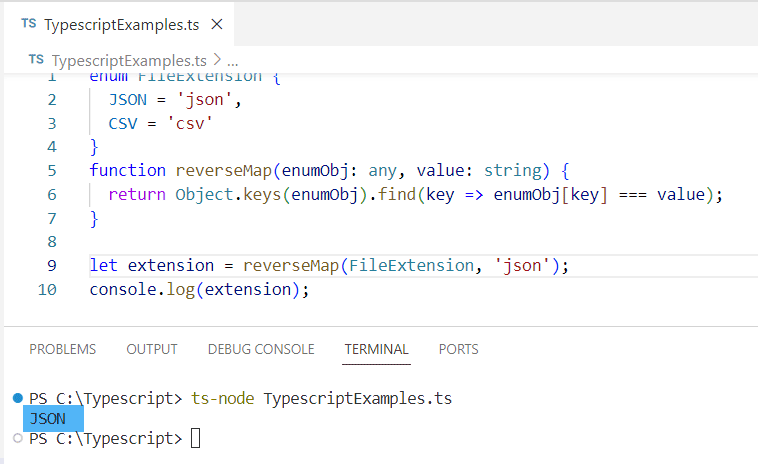
Method 4: Enums as Flags
Enums can also be used as flags, where you can combine multiple values using bitwise operators.
enum Permissions {
Read = 1,
Write = 2,
Execute = 4
}
let myPermissions = Permissions.Read | Permissions.Write;
let canExecute = (myPermissions & Permissions.Execute) !== 0; // false
Reverse mapping also works here, but it becomes a bit more complex due to the bitwise operations.
Conclusion
TypeScript enums are a robust feature, and reverse mapping adds an extra layer of utility, especially in scenarios where you need to map values back to names. It’s particularly useful in error handling, logging, or when working with sets of constants. However, remember that string enums don’t support automatic reverse mapping, so you might have to implement your own solution in such cases. In this Typescript tutorial, I have explained different methods to implement reverse mapping in Typescript.
You may also like:
- Split String by Regex in TypeScript
- How to get string after character in Typescript
- How to get string between 2 characters in Typescript
- How to push an object into an array in Typescript
- How to Get Key by Value from enum String in Typescript
- TypeScript Switch Case Examples
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com