Do you want to split a string into an array in Typescript? In this Typescript tutorial, I will explain how to split a string into an array in Typescript using various methods.
To split a string into an array in TypeScript, use the split()
method. This method separates a string into an array of substrings based on a specified delimiter, like a comma or a space. For instance, const array = 'hello,world'.split(',')
splits the string ‘hello,world’ into [‘hello’, ‘world’]. This is a useful technique for text data parsing and manipulation in TypeScript.
What is the Typescript split() Method?
The split() method in TypeScript is used to divide a string into an ordered list of substrates, creating an array. This method takes a separator as an argument, which determines where the split should occur. If no separator is provided, the entire string is returned as the sole element of an array.
The syntax of the split() method in Typescript is straightforward:
string.split(separator, limit);
- separator (optional): The character or regular expression to use for splitting the string.
- limit (optional): A value to limit the number of splits. The array will contain a maximum of limit elements.
split string to array in Typescript
Let us take an example and try to understand how to split a string into an array in Typescript.
Suppose there is a string.
const cities = "New York,Los Angeles,Chicago,Houston,Phoenix";
To split this string into an array, we use the split() method with a comma (,) as the separator; below is the code:
const cityArray = cities.split(',');
console.log(cityArray);
Here is the complete code:
const cities = "New York,Los Angeles,Chicago,Houston,Phoenix";
const cityArray = cities.split(',');
console.log(cityArray);
Output:
Once you run the code using Visual Studio Code, you can see the output in the below screenshot.
[ 'New York', 'Los Angeles', 'Chicago', 'Houston', 'Phoenix' ]
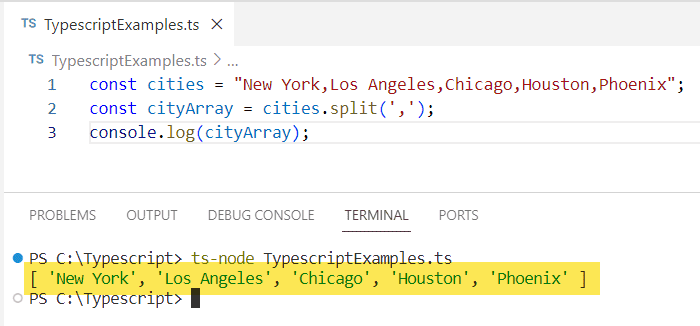
Here are a few other examples related to Typescript split a string into an array.
Typescript split string into array with limit
You can also limit the number of elements in the resulting array in Typescript. For example, to get only the first three elements, you can use the below code:
const cities = "New York,Los Angeles,Chicago,Houston,Phoenix";
const limitedCities = cities.split(',', 3);
console.log(limitedCities);
Output:
Once you run the code, you can see the output in the screenshot below:
[ 'New York', 'Los Angeles', 'Chicago' ]
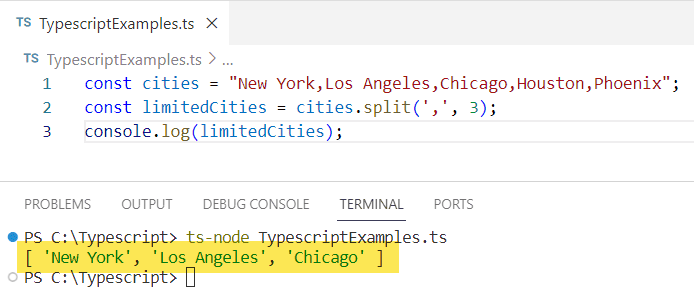
Split string into array in Typescript using Regular Expressions
Now, let us see another example where I will show you how to split a string into an array in Typescript using regular expressions.
Here is the complete code:
const mixedString = "NewYorkLosAngelesChicago";
const splitByCapital = mixedString.split(/(?=[A-Z])/);
console.log(splitByCapital);
The split() method in Typescript can also take a regular expression as a separator. For example, if we want to split a string at every capital letter:
Output:
[ 'New', 'York', 'Los', 'Angeles', 'Chicago' ]
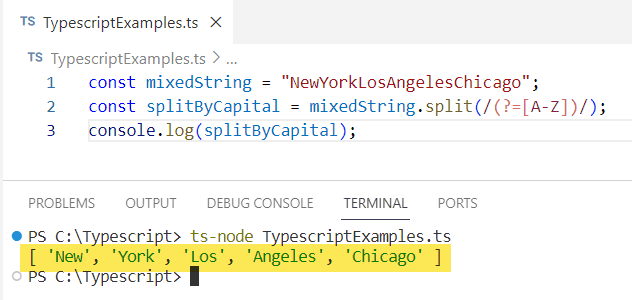
Conclusion
The split()
method is a versatile tool in TypeScript for string manipulation. Whether you’re handling simple comma-separated data or complex patterns, it can easily transform strings into manageable arrays. This functionality is particularly beneficial for data parsing, allowing developers to easily extract and manipulate specific elements from strings.
In this Typescript tutorial, I have explained how to split a string into an array in Typescript using the Typescript split() method.
You may also like:
- Split String by Regex in TypeScript
- Typescript Get Last Element of Array
- Typescript merge two arrays of objects
- Typescript sort array by date
- Typescript reverse array
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com