Do you want to concat array of objects in Typescript? In this Typescript tutorial, I will explain how to merge arrays in Typescript. We will see three examples related to merge two array of objects in Typescript.
To merge two arrays of objects in TypeScript, you can use the spread operator (...
) for a simple concatenation. If you need to ensure uniqueness based on a specific property, use a Map
to filter out duplicates. For more complex scenarios where you want to combine properties of objects with the same identifier, iterate over the arrays and merge properties of matching objects using the spread operator within a Map
.
Typescript merge arrays
Here’s a complete TypeScript example for merging two arrays. This example will demonstrate how to merge two arrays of numbers, but the same logic can be applied to arrays of any type.
function mergeArrays<T>(arr1: T[], arr2: T[]): T[] {
return [...arr1, ...arr2];
}
// Example arrays
const array1: number[] = [1, 2, 3];
const array2: number[] = [4, 5, 6];
// Merging arrays
const mergedArray: number[] = mergeArrays(array1, array2);
// Output the result
console.log(mergedArray);
In this example, mergeArrays
is a generic function that can merge arrays of any type (T
). It uses the spread operator (...
) to create a new array containing all elements from both arr1
and arr2
. The spread operator is a concise and effective way to merge arrays in TypeScript.
Here is the screenshot for the output after I ran the code using VS code.
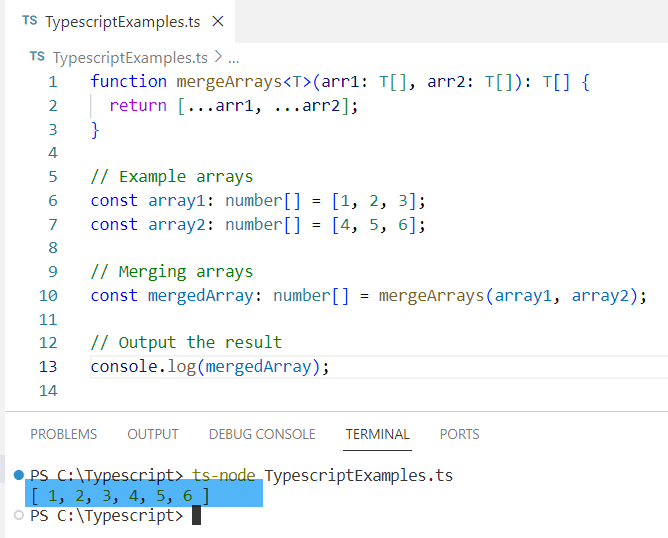
Typescript merge two arrays of objects
Let us explore how to merge two arrays of objects in TypeScript, ensuring that the result is an array containing the elements from both arrays.
For this example, Consider two arrays of objects, each representing a set of user data. Our goal is to merge these arrays into a single array.
interface User {
id: number;
name: string;
email: string;
}
const array1: User[] = [
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' }
];
const array2: User[] = [
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
{ id: 4, name: 'David', email: 'david@example.com' }
];
Method 1: Simple Merge
The simplest way to merge two arrays in TypeScript is by using the spread operator (...
). This method is suitable when you just need to concatenate the arrays without any additional logic.
Here is the code to merge two arrays in Typescript.
const mergedArray: User[] = [...array1, ...array2];
console.log(mergedArray);
This code will output a new array containing all the elements from array1
followed by all the elements from array2
.
Here is the complete code:
interface User {
id: number;
name: string;
email: string;
}
const array1: User[] = [
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' }
];
const array2: User[] = [
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
{ id: 4, name: 'David', email: 'david@example.com' }
];
const mergedArray: User[] = [...array1, ...array2];
console.log(mergedArray);
Once you run the code using VS code, you can see the output of the typescript concatenate arrays like the screenshot below.
[
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' },
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
{ id: 4, name: 'David', email: 'david@example.com' }
]
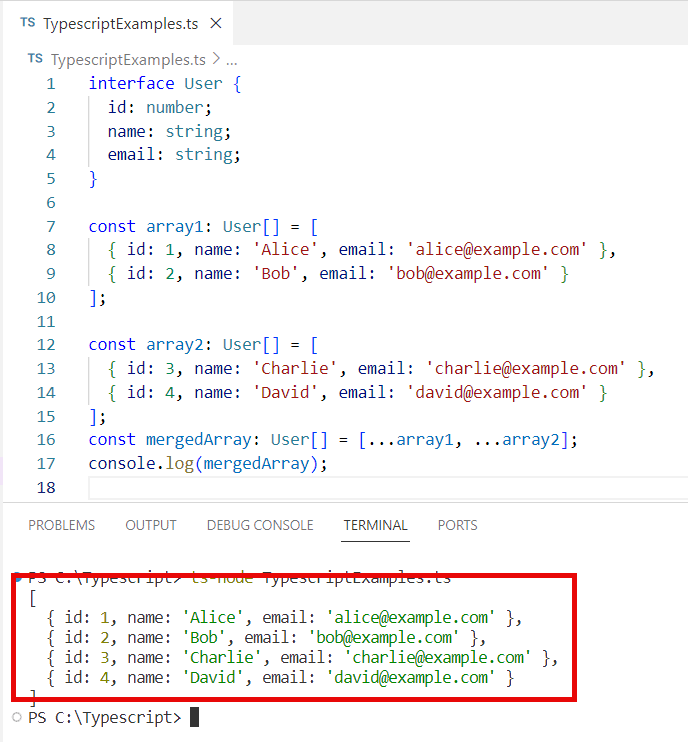
Method 2: Merge two arrays with unique objects
If you need to ensure that the merged array only contains unique objects (based on a specific property like id
) in Typescript, you can use the below code:
const mergeUnique = (arr1: User[], arr2: User[]): User[] => {
const tempMap = new Map<number, User>();
[...arr1, ...arr2].forEach(user => {
tempMap.set(user.id, user);
});
return Array.from(tempMap.values());
};
const uniqueMergedArray = mergeUnique(array1, array2);
console.log(uniqueMergedArray);
This function uses a Map
to track unique users based on their id
. If a user with the same id
is encountered, it overwrites the existing entry, ensuring uniqueness.
Here is the complete Typescript code to concat array of objects.
interface User {
id: number;
name: string;
email: string;
}
const array1: User[] = [
{ id: 1, name: 'Alice', email: 'alice@example.com' },
{ id: 2, name: 'Bob', email: 'bob@example.com' }
];
const array2: User[] = [
{ id: 3, name: 'Charlie', email: 'charlie@example.com' },
{ id: 4, name: 'David', email: 'david@example.com' }
];
const mergeUnique = (arr1: User[], arr2: User[]): User[] => {
const tempMap = new Map<number, User>();
[...arr1, ...arr2].forEach(user => {
tempMap.set(user.id, user);
});
return Array.from(tempMap.values());
};
const uniqueMergedArray = mergeUnique(array1, array2);
console.log(uniqueMergedArray);
You can see the output in the screenshot below:
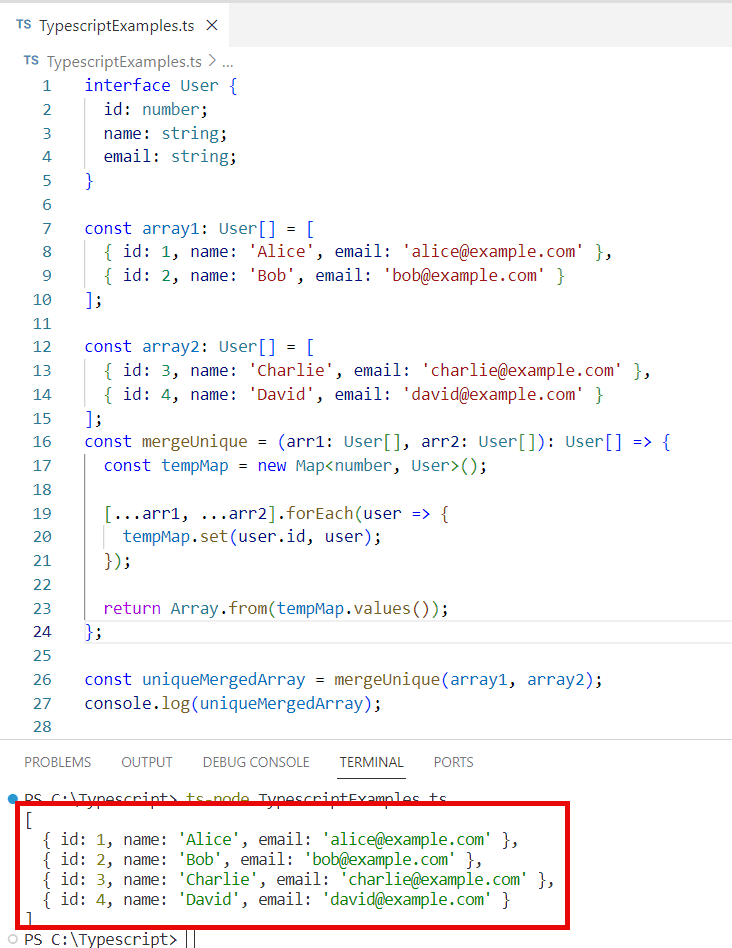
Method 3: Merging and Combining Properties
In some cases, you may want to merge arrays in Typescript and combine the properties of objects with the same identifier. This can be achieved by iterating over the arrays and merging the properties of matching objects.
const mergeAndCombine = (arr1: User[], arr2: User[]): User[] => {
const combinedMap = new Map<number, User>();
[...arr1, ...arr2].forEach(user => {
if (!combinedMap.has(user.id)) {
combinedMap.set(user.id, user);
} else {
combinedMap.set(user.id, { ...combinedMap.get(user.id), ...user });
}
});
return Array.from(combinedMap.values());
};
const combinedMergedArray = mergeAndCombine(array1, array2);
console.log(combinedMergedArray);
In this approach, if two objects have the same id
, their properties are combined using the spread operator.
Typescript concat array of strings
Here’s a complete TypeScript example demonstrating how to concatenate two arrays of strings in Typescript:
function concatArrays(arr1: string[], arr2: string[]): string[] {
return arr1.concat(arr2);
}
// Example arrays
const array1: string[] = ['Hello', 'World'];
const array2: string[] = ['TypeScript', 'is', 'awesome'];
// Concatenating arrays
const concatenatedArray: string[] = concatArrays(array1, array2);
// Output the result
console.log(concatenatedArray);
Output:
[ 'Hello', 'World', 'TypeScript', 'is', 'awesome' ]
Once you run the code using Visual Studio code, you can see the output in the screenshot below:
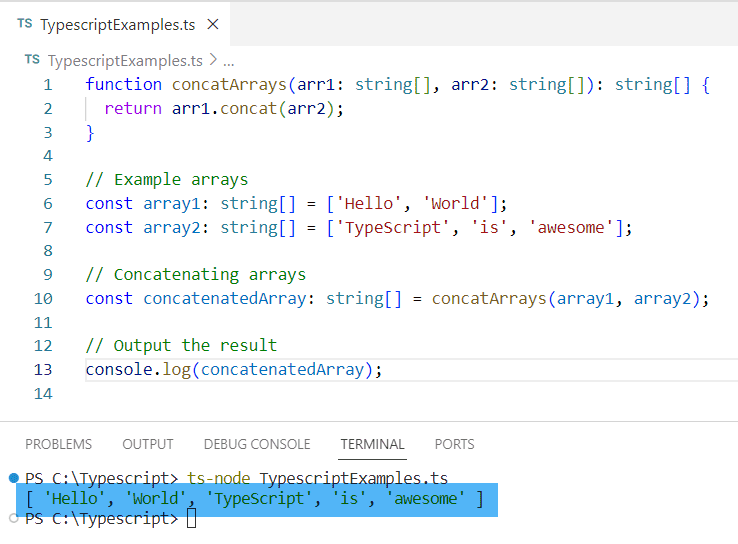
In this example, the concatArrays
function takes two arrays of strings as input and returns a new array formed by concatenating arr2
to the end of arr1
. The .concat()
method is used, which merges two or more arrays by returning a new array without modifying the original arrays.
Conclusion
Merging arrays of objects in TypeScript can be done in several ways, depending on the requirements of your application. Whether you need a simple concatenation or a more complex merge that ensures uniqueness or combines properties, TypeScript provides the flexibility and power to accomplish these tasks. In this Typescript tutorial, I have explained different examples of Typescript merge arrays and Typescript merge two arrays of objects.
You may also like:
- Typescript array find
- Typescript sort array by date
- Typescript reverse array
- Typescript filter array of objects
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com