Do you want to reverse a Typescript array? In this tutorial, I will explain three methods for Typescript reverse array with complete code and examples.
To reverse an array in TypeScript, you can use the reverse() method, which directly mutates the original array. If you need to preserve the original order, create a copy of the array and then apply reverse(). For more control, manually reverse the array using a loop, which allows you to keep the original array unchanged and offers flexibility in handling the reversal process.
Typescript reverse array
Let us explore different methods to reverse an array in Typescript.
- Using the reverse() Method
- Reversing Without Mutation
- Using a Loop
1. Typescript reverse array using reverse() method
Let us first see how to reverse an array using the reverse() method in typescript. The reverse() method mutates the original array and reverses its elements.
Here is an example:
let cities: string[] = ["New York", "Los Angeles", "Chicago", "Houston", "Phoenix"];
cities.reverse();
console.log(cities);
Output:
[ 'Phoenix', 'Houston', 'Chicago', 'Los Angeles', 'New York' ]
Once you run the code using Visual Studio Code, you can see the output in the screenshot below:
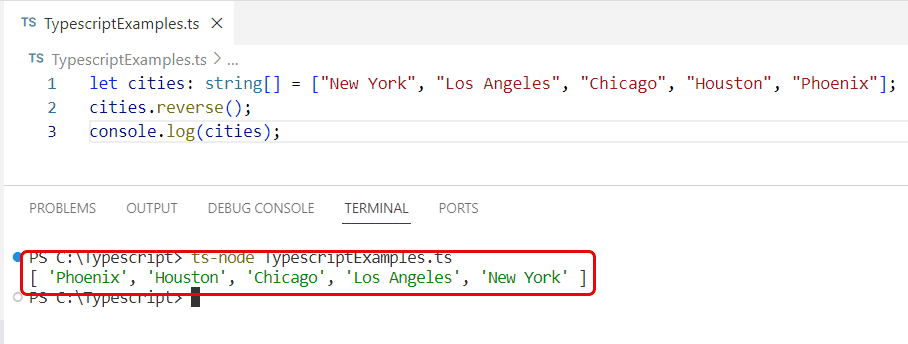
Pros:
- Easy and quick to use.
- Directly alters the original array, which can be useful if you don’t need the original order anymore.
Cons:
- Mutates the original array, which is not ideal if you need to preserve the original order.
2. Reverse array in Typescript using Reversing Without Mutation
If you need to reverse an array in Typescript but also keep the original order, you can first create a copy of the array and then apply the reverse() method.
Here is a complete code:
let cities: string[] = ["Miami", "Atlanta", "Seattle", "Denver", "Boston"];
let reversedCities = [...cities].reverse();
console.log(reversedCities);
console.log(cities);
Output:
[ 'Boston', 'Denver', 'Seattle', 'Atlanta', 'Miami' ]
[ 'Miami', 'Atlanta', 'Seattle', 'Denver', 'Boston' ]
After executing the code using VS code, you can see the output in the screenshot below:
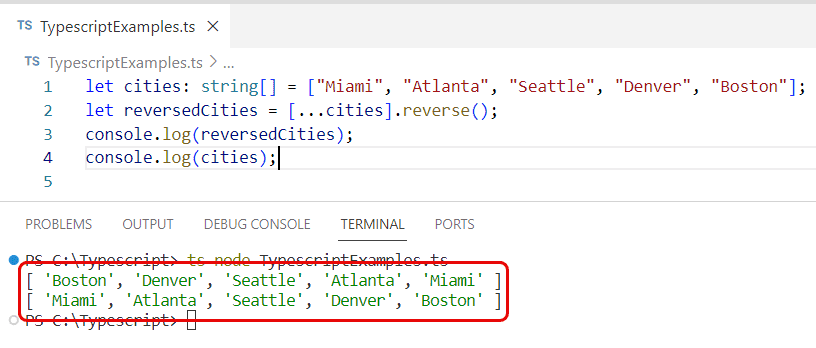
Pros:
- Keeps the original array intact.
- Flexible and easy to understand.
Cons:
- Creates a new array, which can be a downside in terms of memory usage, especially with large arrays.
3. Typescript reverse array using a loop
You can reverse an array using a loop in Typescript. Here is the complete code:
let cities: string[] = ["San Francisco", "Las Vegas", "Washington D.C.", "Orlando", "Dallas"];
let reversedCities: string[] = [];
for (let i = cities.length - 1; i >= 0; i--) {
reversedCities.push(cities[i]);
}
console.log(reversedCities);
Output:
[
'Dallas',
'Orlando',
'Washington D.C.',
'Las Vegas',
'San Francisco'
]
Once you run the code, you can see the output in the screenshot below.
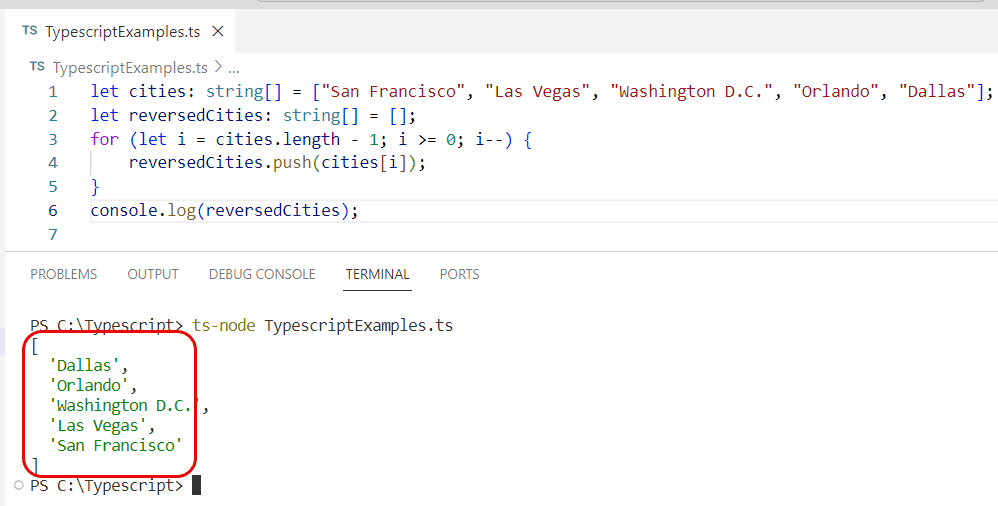
Pros:
- Offers full control over the reversing process.
- Does not mutate the original array.
Cons:
- More verbose and less intuitive.
- Slightly slower for large arrays.
Conclusion
Reversing an array in TypeScript can be done in various ways, each with it’s pros and cons. The method you choose will depend on your specific requirements, such as whether you need to preserve the original order of the array or if memory efficiency is a concern. In this Typescript tutorial, you learned how to reverse an array in Typescript using different methods like reverse() method, looping, etc.
You may also like:
- Typescript array concatenation
- Typescript sort array
- Typescript array find
- Typescript filter array of objects
- TypeScript Array filter() Method
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com