In this Typescript tutorial, we will learn how to use the typescript array find() method with a few examples. Here are the examples are listed below:
- Typescript array find
- Typescript array find vs filter
- Typescript array find vs some
- Typescript array find vs includes
- Typescript array find first
- Typescript array find object by property
- Typescript array find object is possibly undefined
- Typescript array find and update
- Typescript array find all matches
- Typescript array find by id
- Typescript array find by condition
- Typescript array find object
- Typescript array find string
If you are new to typescript, check out, Typescript hello world program for beginners
Typescript array find
The find() method in typescript returns the first entry in the specified array that meets the provided testing function. Undefined is returned if no values meet the testing function.
The syntax of the find method in typescript:
// Arrow function
find((elem) => { /* … */ })
find((elem, index) => { /* … */ })
find((elem, index, array) => { /* … */ })
// Callback function
find(callbackFn)
find(callbackFn, thisArg)
// Inline callback function
find(function (elem) { /* … */ })
find(function (elem, index) { /* … */ })
find(function (elem, index, array) { /* … */ })
find(function (elem, index, array) { /* … */ }, thisArg)
Example of find method in typescript:
Let’s say we have an array of numbers, and we will find the number from an array using the find() method. In the app.ts file write the below code:
const num1=[12, 14, 18, 22, 24];
const findNum= num1.find(elem=> elem ===12);
console.log(findNum);
To compile the code and run the below command and you can see the result in the console:
ts-node app.ts
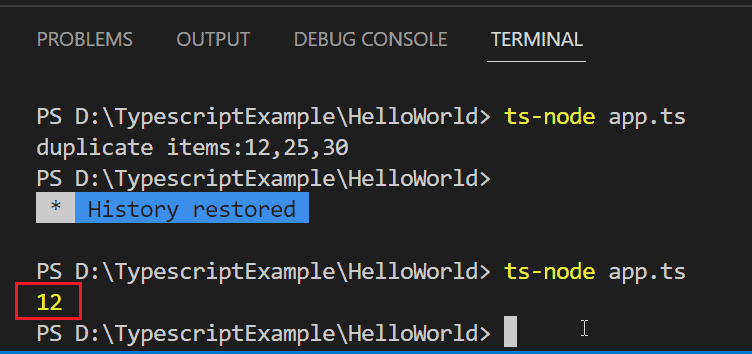
This is what the typescript array find method is, and how we can use it.
Typescript array find vs filter
Here we will see the difference between find and filter methods in typescript.
As there are several methods to get the values from an array, for this we are going to use the find and filter method in typescript.
Find() in typescript:
The find() method returns the very first matching value from the collection. It will not test the other values in the array collection once it satisfies the value in findings.
Example of find method in typescript
In typescript find method, we will see how we can get an item from an array. In the app.ts file write the below code:
var state_details = [{
Name: 'California',
Capital: 'Sacramento'
}, {
Name: 'Texas',
Capital: 'Austin'
}, {
Name: 'Alaska',
Capital: 'Juneau'
},
{
Name: 'Alaska',
Capital: 'Juneau'
}, {
Name: 'Georgia',
Capital: 'Atlanta'
}];
const findState= state_details.find(function(item) {
return item.Name == "Alaska"
});
console.log(findState)
To compile the code, run the below command and you can see the result in the console.
ts-node app.ts
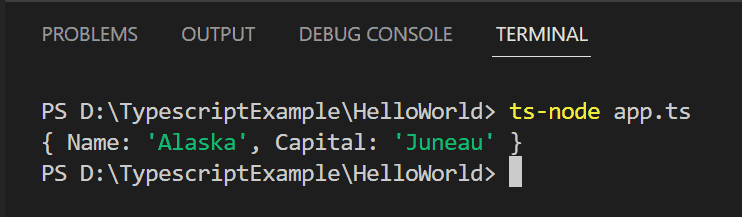
Filter method in typescript
The filter() in the typescript will return all the matched items from an array. Whereas it will check all items in an array.
Example of filter method in typescript
In typescript the filter method, we will see how we can filter the matching value from an array. In the app.ts file write the below code:
var state_details = [{
Name: 'California',
Capital: 'Sacramento'
}, {
Name: 'Texas',
Capital: 'Austin'
}, {
Name: 'Alaska',
Capital: 'Juneau'
},
{
Name: 'Alaska',
Capital: 'Juneau'
}, {
Name: 'Georgia',
Capital: 'Atlanta'
}];
const findState= state_details.filter(function(item) {
return item.Name == "Alaska"
});
console.log(findState)
To compile the code, run the below command and you can see the output in the console:
ts-node app.ts
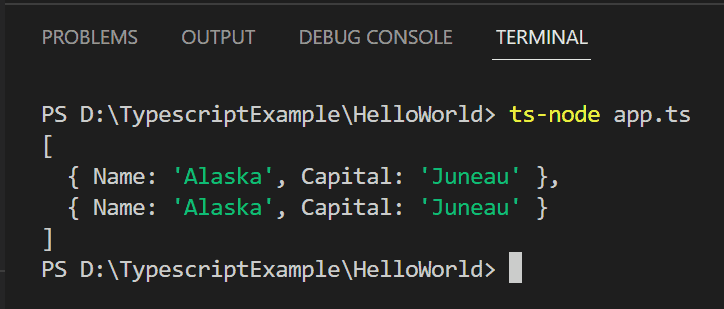
This is the difference between array find and filter in typescript.
Read TypeScript Date
Typescript array find vs some
Here we will see the difference between find method and some method in typescript.
Let’s see the difference between some method vs find method.
Some () in typescript
This method in typescript is allows us to determine whether one or more items of array correspond to what you are looking for.
Example of some() in typescript.
We will have a list of array, if the item is available in the array, some () will return true else return false. In the app.ts file write the below code:
var arr = ['hello', 'a', 'bc'];
var result = arr.some(item => item === 'bc');
console.log(result)
To compile the code and run the below command and you can see the result in the console.
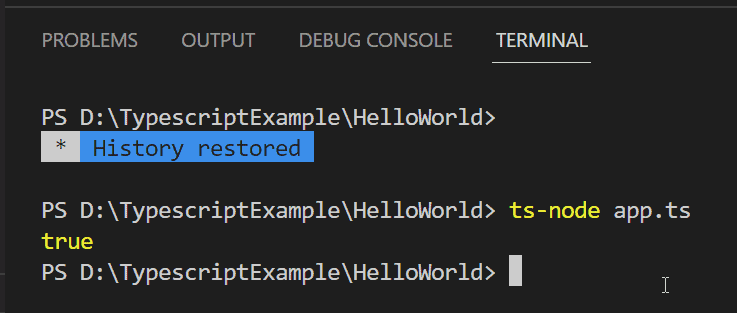
Find() in typescript
This method in typescript, will return the first value based on the condition.
Example of Find method in typescript
We will have a list of array, if the item is available in the array, find () will return true else return false. In the app.ts file write the below code:
var arr = ['hello', 'a', 'bc'];
//find()
var result = arr.find(item => item === 'bc');
console.log(result);
To compile the code run the below command and you can see the result in the console.
ts-node app.ts
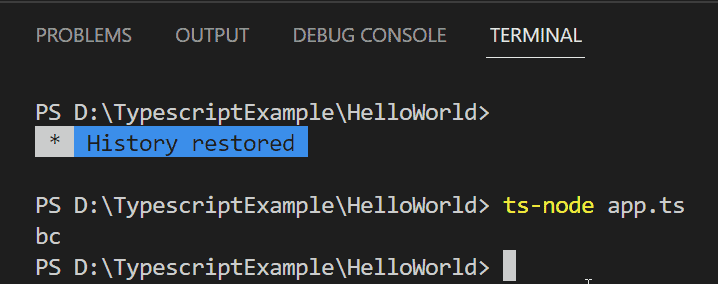
This is the difference between array find and some method in typescript.
Read TypeScript Map
Typescript array find vs includes
Here we will see the difference between find vs includes method in typescript.
Let’s see the difference between includes() and find () in typescript.
Include () in Typescript
This method will check whether array contains the item or not, ifit contains it returns true else false.
Example of Include() in typescript
We have array of string, the array contains ‘bc’ string, then the include method will return true or else false.
In the app.ts file write the below code:
var arr = ['hello', 'a', 'bc'];
var result = arr.includes('bc');
console.log(result);
To compile the code run the below command and you can see the output in the console.
ts-node app.ts
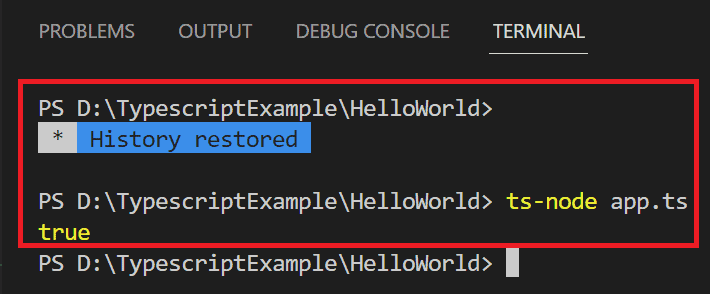
Find() in typescript
This method in typescript, will return the first value based on the condition.
Example of Find method in typescript
We will have a list of array, if the item is available in the array, find () will return true else return false. In the app.ts file write the below code:
var arr = ['hello', 'a', 'bc'];
//find()
var result = arr.find(item => item === 'bc');
console.log(result);
To compile the code run the below command and you can see the result in the console.
ts-node app.ts
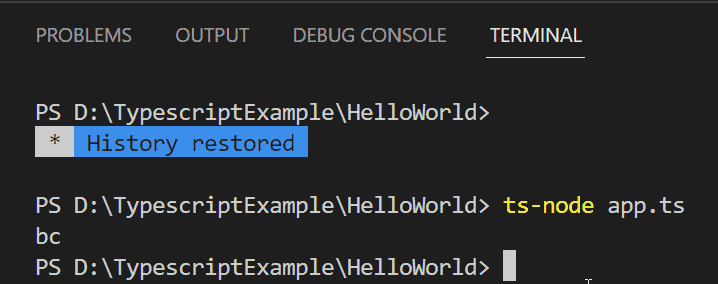
This is the difference between array find and includes method in typescript.
Typescript array find object by property
Here we will see an example of how to get an object by property from an array in typescript
For example, we have an array of objects so we will find the state name ‘Alaska’ is available or not. In the app.ts file, write the below code:
var state_details = [{
Name: 'California',
Capital: 'Sacramento'
}, {
Name: 'Texas',
Capital: 'Austin'
}, {
Name: 'Alaska',
Capital: 'Juneau'
},
{
Name: 'Alaska',
Capital: 'Juneau'
}, {
Name: 'Georgia',
Capital: 'Atlanta'
}];
function isAlaska(state_details:any) {
return state_details.Name == "Alaska";
}
console.log(state_details.find(isAlaska));
To compile the code, run the below command, and you can see the result in the console.
ts-node app.ts
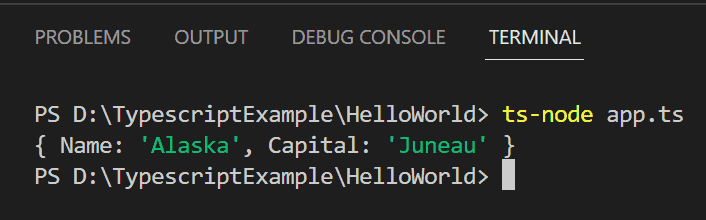
This is an example of a Typescript array find object by property.
Read TypeScript Set and Weakset
Typescript array find first
Here we will see how to use the find a method to get the first match item from an array in typescript.
For example, we have an array of employee details, which contains employee names and departments. So in this array, we have some details containing the same employee names with different departments, so here we will filter an array using find method based on the employee name, then it will return the first match details from employee details.
Let’s say we need to find the employee name ‘Adam’, so it will return you the first match from an array. In the app.ts file, write the below code:
var emp_details = [{
Name: 'Adam',
Department: 'IT'
}, {
Name: 'Adam',
Department: 'HR'
}, {
Name: 'Alex',
Department: 'Accounts'
},
{
Name: 'James',
Department: 'HR'
}, {
Name: 'John',
Capital: 'Accounts'
}];
function getEmp(emp_details:any) {
return emp_details.Name == "Adam";
}
console.log(emp_details.find(getEmp));
To compile the code, run the below command and see the result in the console.
ts-node app.ts
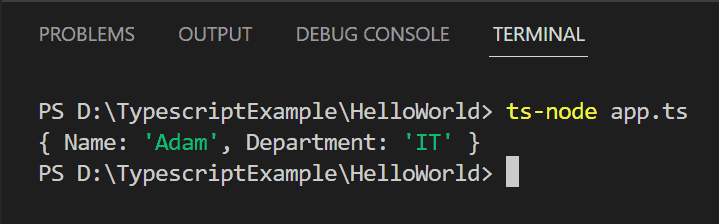
This is an example of Typescript array find first
Typescript array find object is possibly undefined
Here we will see an example of how to solve the ‘undefined’ results from the find() method in TypeScript.
In TypeScript, the Array.find() usually returns an undefined value only when the condition applied in the callback code is never satisfied. Moreover, it will also return the undefined value when we do not return any value from the callback function.
Let us look at an example when the Array.find() will return an undefined value.
//code which returns undefined value
const emp_details = [
{ emp_id: 101, name: 'Harry' },
{ emp_id: 102, name: 'Ron' },
{ emp_id: 103, name: 'Alex' },
];
const result = emp_details.find((element) => {
return element.emp_id === 103;
});
console.log(result.name);
To compile the code, run the below command and you will come across the error” ‘result’ is possibly ‘undefined'”.
ts-node app.ts

To solve the above error, we will use the optional chaining operator ‘?’, which is used to access the object property or calls a function. Now, change the app.ts code with the below code:
const emp_details = [
{ emp_id: 101, name: 'Harry' },
{ emp_id: 102, name: 'Ron' },
{ emp_id: 103, name: 'Alex' },
];
const result = emp_details.find((element) => {
return element.emp_id === 103;
});
console.log(result?.name);
Now run the below command and you can see the output in the console:
ts-node app.ts
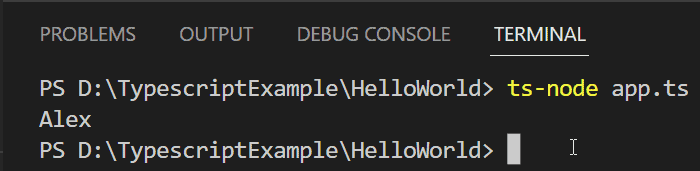
This is an example of Typescript array find object is possibly undefined.
Read TypeScript Enum
The typescript array finds all matches
Here we will see how to find all matches from an array in Typescript.
As we know find() method, will return you the first match item from an array in typescript. To get the all matches items from an array in typescript, we can use the filter method in typescript .
Typescript array find by condition
Here we will see how to find the first element from an array that matches the condition.
To find the first item from an array using typescript:
- Iterate through the array using the Array.find() function.
- Check to see whether each value meets the requirement.
- The first array item that meets the requirement is returned by the find function.
Now, let’s see an example, of how we can find the first item from an array by condition using the find method. In the app.ts file write the below code:
const num = [11, 2, 3, 4, 15,14,18,19];
const result = num.find(elem => {
return elem > 4;
});
console.log(result);
To compile the code run the below command and you can see the result in the console.
ts-node app.ts
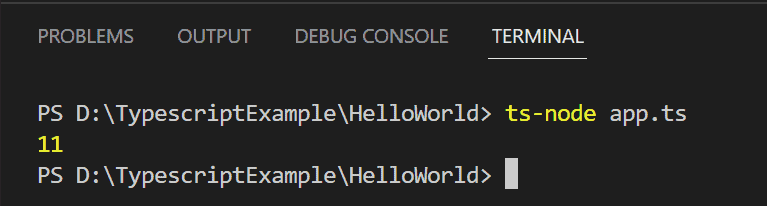
This is an example Typescript array find by condition.
Read Typescript Identifiers and Keywords
Typescript array find by id
Here we will see how we can find an object from an array by id in typescript.
With the find method, we will get the first match of the object from an array, which fits the conditions in the callback functions. If none of the objects fit the supplied condition, this function returns undefined.
Let’s see an example of getting an object from an array using find() in a typescript. In the app.ts file write the below code:
var empEligibilityDetails = [
{
id:1,
"name": "Rock",
"age": 30,
"isManager": false
},
{
id:2,
"name": "Ammy",
"age": 31,
"isManager": false,
},
{
id:3,
"name": "Alex",
"age": 32,
"isManager": true,
},
{
id:4,
"name": "Ron",
"age": 36,
"isManager": true,
}
];
const objFound= empEligibilityDetails.find(obj => obj.id === 3);
console.log(objFound);
To compile the code, run the below command and you can see the result in the console:
ts-node app.ts
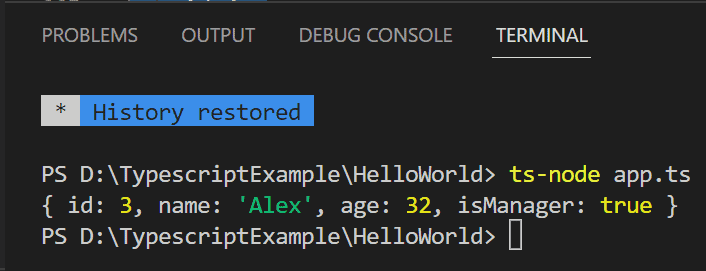
This is an example of Typescript array find by Id.
Read Typescript type annotation
Typescript array find and update
Here we will see an example of how to find and update an object in an array using typescript.
For example, we will see how we can find the first employee info from an array using find method, whose age is greater than 31, and we will set the ‘isManager’ property value as false.
In the app.ts file write the below code:
var empEligibilityInfo = [
{
id:1,
"name": "Rock",
"age": 30,
"isManager": false
},
{
id:2,
"name": "Ammy",
"age": 31,
"isManager": false,
},
{
id:3,
"name": "Alex",
"age": 32,
"isManager": true,
},
{
id:4,
"name": "Ron",
"age": 36,
"isManager": true,
}
];
var empData = empEligibilityInfo.find(value => value.age > 31)
empData!.isManager = false;
console.log(empEligibilityInfo);
To compile the code, run the below command, and you can see the result in the console:
ts-node app.ts
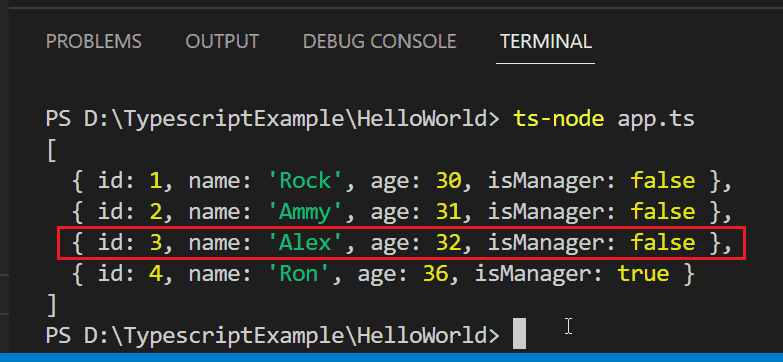
This is an example of Typescript array find and update.
Read Typescript array
Typescript array finds an object
Here we will see an example of how we can find objects from an array in typescript.
For example, we will use the find method to find an object id= 33, from an array in typescript. In the app.ts file, write the below code:
const arrCountry = [
{ id: 12, country: 'Mexico' },
{ id: 20, country: 'Germany' },
{ id: 33, country: 'Brazil' },
];
// ✅ Find first object that matches condition
const result = arrCountry.find((obj) => {
return obj.id === 33;
});
// 👇️ { id: 33, country: 'Brazil' }
console.log(result);
To compile the code, run the below command and you can see the result in the console.
ts-node app.ts
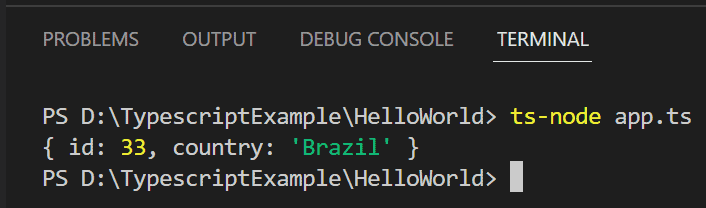
This is an example of Typescript array finds an object
Typescript array finds string
Here we will see how we can check the array contains a string in a typescript using the find method.
For example, we will use the find method, which will return the first occurrence of the required item, if it finds, then you can get the result in the console, else you can see undefined in the console.
In the app.ts file write the below code:
var countryArray : string[] = ['USA', 'Africa', 'UK', 'Russia'];
if(countryArray.find(e => e === 'UK')){
console.log('UK is present in country list');
To compile the code, run the below command and you can see the result in the console:
ts-node app.ts
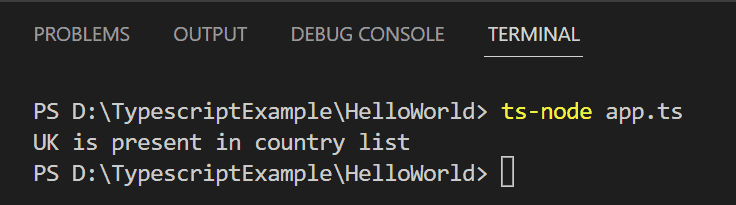
This is an example of array finds string in typescript
Conclusion
In this typescript tutorial, we learned all about the find method in typescript. Also, we saw different examples to use the find method in typescript. Here are the examples we cover:
- Typescript array find
- Typescript array find vs filter
- Typescript array find vs some
- Typescript array find vs includes
- Typescript array find first
- Typescript array find object by property
- Typescript array find object is possibly undefined
- Typescript array find and update
- Typescript array find all matches
- Typescript array find by id
- Typescript array find by condition
- Typescript array find distinct
- Typescript array finds object
- Typescript array find string
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com