Do you want to merge two maps in Typescript? In this tutorial, I explain everything about Typescript merge maps. We will explore various methods of merging maps in TypeScript with complete code.
To merge maps in TypeScript, you can use the spread operator, the forEach method, or custom merge functions. The spread operator is best for simple merges, forEach offers more control for updates, and custom functions are ideal for complex scenarios requiring conflict resolution or specific merging logic. Each method caters to different needs, ensuring efficient and tailored data management in your TypeScript projects.
Maps in TypeScript
A Map in Typescript is a collection of keyed data items, just like an Object. However, unlike objects, maps allow keys of any type. TypeScript offers strong typing for these collections, enhancing code reliability.
Typescript merge two maps
In this section, I will show you three methods of how to merge two maps in Typescript.
1. Merge maps in Typescript using the Spread Operator
The Typescript spread operator (…) is a convenient way to merge maps. It’s a modern JavaScript feature that TypeScript also supports.
Here is a complete code:
let map1 = new Map([[1, 'one'], [2, 'two']]);
let map2 = new Map([[3, 'three'], [4, 'four']]);
let mergedMap = new Map([...map1, ...map2]);
console.log(mergedMap);
Output:
Map(4) { 1 => 'one', 2 => 'two', 3 => 'three', 4 => 'four' }
You can see the screenshot below for the output; I ran the code using Visual Studio code:
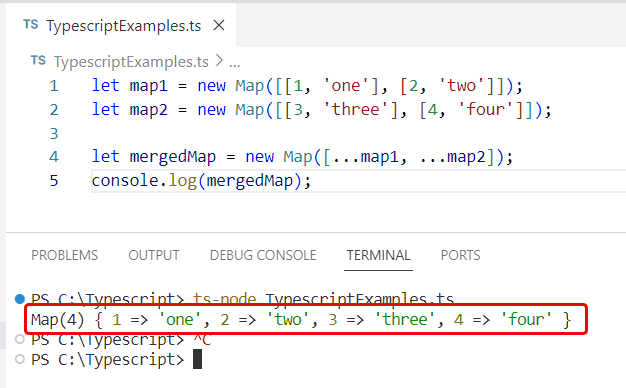
2. Typescript combine two maps using the forEach Method
You can also use the foreach() method to combine two maps in Typescript. Here is the complete code:
let map1 = new Map([[1, 'one'], [2, 'two']]);
let map2 = new Map([[3, 'three'], [2, 'updated two']]);
map2.forEach((value, key) => {
map1.set(key, value);
});
console.log(map1);
Output:
Map(3) { 1 => 'one', 2 => 'updated two', 3 => 'three' }
You can also see the output in the screenshot below:
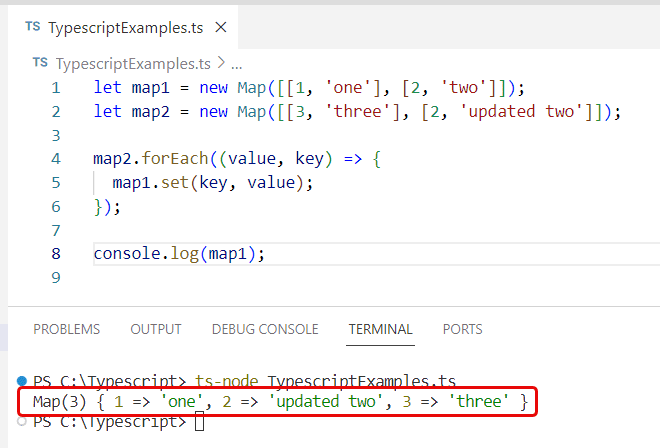
3. Typescript merge two maps using custom merge functions
As a Typescript developer, you can also write a custom merge function to merge two maps in Typescript. Here is the complete code to merge two maps using custom merge functions in Typescript:
function mergeMaps(map1: Map<number, string>, map2: Map<number, string>): Map<number, string> {
let result = new Map(map1);
map2.forEach((value, key) => {
if(result.has(key)) {
result.set(key, `${result.get(key)} and ${value}`);
} else {
result.set(key, value);
}
});
return result;
}
let map1 = new Map([[1, 'one'], [2, 'two']]);
let map2 = new Map([[2, 'second'], [3, 'three']]);
let mergedMap = mergeMaps(map1, map2);
console.log(mergedMap);
Once you run the code, you will the merged map like the below screenshot.
Map(3) { 1 => 'one', 2 => 'two and second', 3 => 'three' }
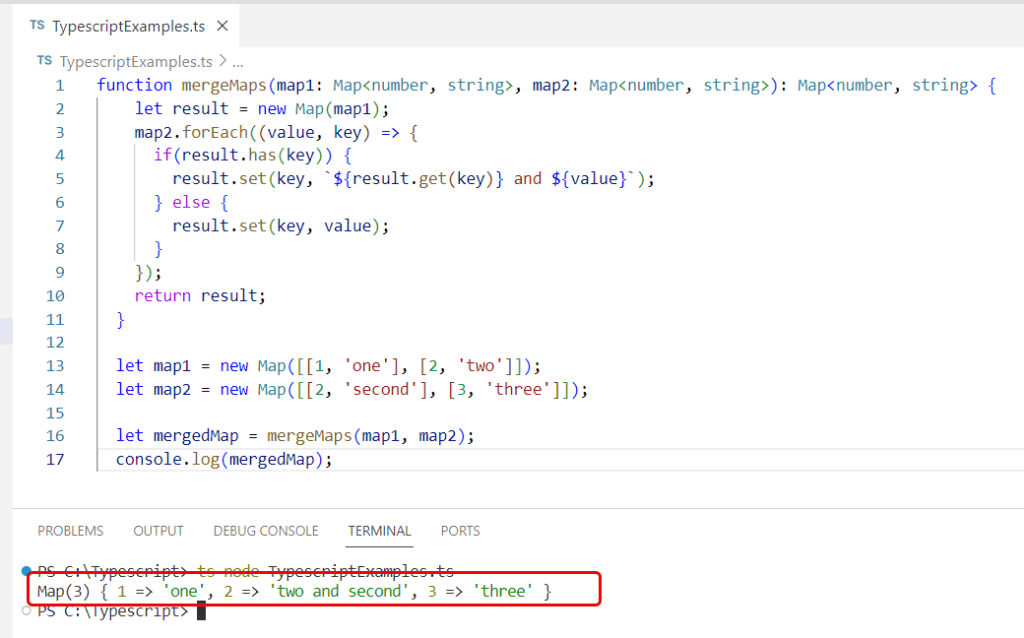
Conclusion
In this Typescript tutorial, I have explained various ways to merge or combine two maps in Typescript.
- Merge maps in Typescript using the Spread Operator
- Typescript combine two maps using the forEach Method
- Typescript merge two maps using custom merge functions
You may also like:
- Compare two string dates in typescript
- How to get last element of an array in typescript
- Typescript array concatenation
- Convert Typescript Dictionary to String
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com