In this Typescript tutorial, I will explain to you how to get the month, day, and year from a date in TypeScript. When working with dates in TypeScript, it’s essential to know how to work with various components like month, day, year, etc.
Typescript get month day year from date
In Typescript, you can get the current date and time by using the Typescript Date object. Here is the code:
let currentDate = new Date();
console.log(currentDate);
Get Year
To get the year from a date object in Typescript, you can use the getFullYear() method. This method returns the year of the specified date according to local time.
Here is the Typescript code to get the year from the date in Typescript.
let year = currentDate.getFullYear();
console.log(`The year is: ${year}`);
The output will come here as:
The year is: 2023
Get Month
Like JavaScript, Typescript counts months from 0 to 11. January is 0, February is 1, and so on. To get the month from the Typescript date object, you use the getMonth() method and add 1 to adjust the month number to the familiar 1-12 range.
Here is the complete code to get the month from the Typescript date:
let month = currentDate.getMonth() + 1;
console.log(`The month is: ${month}`);
Get Day of the Month
In Typescript, you can use the getDate() method is used to get the day of the month from a date object. Here is a code example:
let day = currentDate.getDate();
console.log(`The day of the month is: ${day}`);
Get month, day, year from the date in Typescript Example
Below is a TypeScript program that demonstrates how to retrieve the current year, month, and day from a Date object in Typescript, and then formats these components into a single string.
// Define a function to format a date into a string of 'month/day/year'
function formatDate(date: Date): string {
// Get the year from the date object
const year = date.getFullYear();
// Get the month from the date object, adjust for indexing from 0
const month = date.getMonth() + 1;
// Get the day of the month from the date object
const day = date.getDate();
// Format the date string in 'MM/DD/YYYY' format
return `${month}/${day}/${year}`;
}
// Example usage:
const currentDate = new Date(); // Create a new Date object for the current date and time
const formattedDate = formatDate(currentDate); // Format the current date
// Output the results to the console
console.log(`The year is: ${currentDate.getFullYear()}`);
console.log(`The month is: ${currentDate.getMonth() + 1}`); // Remember to add 1 to the month
console.log(`The day of the month is: ${currentDate.getDate()}`);
console.log(`The formatted date is: ${formattedDate}`);
After executing the code using Visual Studio Code, the output will come like below.
The year is: 2023
The month is: 11
The day of the month is: 10
The formatted date is: 11/10/2023
Below is the screenshot.
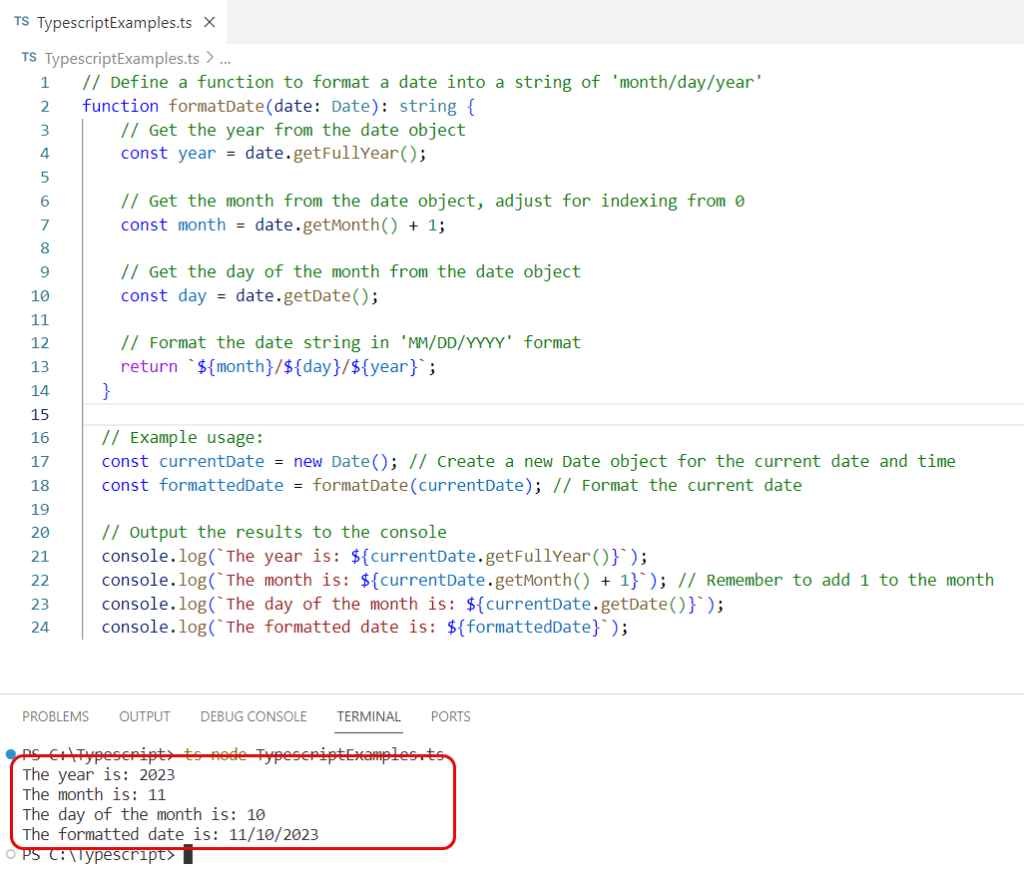
Conclusion
I hope you got to know the Typescript Date object and its methods, such as getFullYear(), getMonth(), and getDate(). You can use these methods to extract the year, month, and day in Typescript. This is how to get month day year from date in Typescript.
You may also like:
- Get Week Number from Date in TypeScript
- How to create date from year month day in Typescript?
- Get First and Last Day of Week in Typescript
- TypeScript Date Add Days
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com