Do you want to get the first or last day of week in Typescript? In this Typescript tutorial, I will explain how to get the first and last day of the week in Typescript.
To get the first or last day of the week in TypeScript, you can use the native Date object or libraries like Moment.js or Day.js. The native method involves manipulating the date to add the remaining days of the week, while libraries offer functions like endOf(‘week’) to directly get the last day. Choose the method that best fits your project’s requirements for efficient and precise date handling.
Get the First Day of Week in Typescript
JavaScript (and by extension, TypeScript) counts days of the week starting from Sunday (0) to Saturday (6).
1. Using Date Object
The most straightforward approach involves the native Date object in Typescript. Here’s how you can get the first day of the week in Typescript.
function getFirstDayOfWeek(date: Date): Date {
const day = date.getDay();
const firstDay = new Date(date);
firstDay.setDate(date.getDate() - day);
return firstDay;
}
// Usage
const today = new Date();
const firstDay = getFirstDayOfWeek(today);
console.log(firstDay);
This function takes a Date object, finds the current day of the week, and subtracts that number of days to reach Sunday, the first day of the week.
You can see the output in the screenshot below.
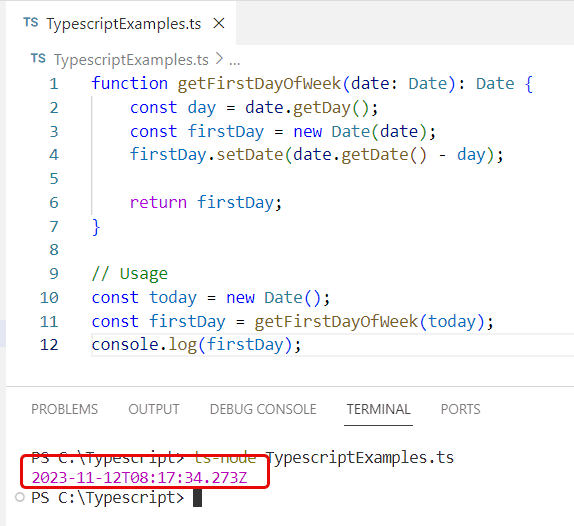
2. Using Moment.js
For projects already using Moment.js, getting the first day of the week becomes simpler. Moment.js is a powerful, versatile library for date manipulation. Here is the complete code:
import moment from 'moment';
function getFirstDayOfWeekUsingMoment(date: Date): Date {
return moment(date).startOf('week').toDate();
}
// Usage
const today = new Date();
const firstDay = getFirstDayOfWeekUsingMoment(today);
console.log(firstDay);
The startOf(‘week’) method in Moment.js adjusts the date to the beginning of the week.
3. Using Day.js
Day.js is a lightweight alternative to Moment.js, offering similar functionality. Here is the complete code for “typescript date get last day of week” using Day.js
import dayjs from 'dayjs';
function getFirstDayOfWeekUsingDayjs(date: Date): Date {
return dayjs(date).startOf('week').toDate();
}
// Usage
const today = new Date();
const firstDay = getFirstDayOfWeekUsingDayjs(today);
console.log(firstDay);
Like Moment.js, Day.js has a startOf(‘week’) method, which is used to get the first day of the week.
Get Last Day of Week in Typescript
Now, let us see how to get the last day of week in Typescript using various methods.
1. Using the Date Object
The simplest way to find the last day of the week in Typescript is by using JavaScript’s native Date object.
function getLastDayOfWeek(date: Date): Date {
const lastDay = new Date(date);
lastDay.setDate(date.getDate() + (6 - date.getDay()));
return lastDay;
}
// Usage
const today = new Date();
const lastDay = getLastDayOfWeek(today);
console.log(lastDay);
You can see the output in the screenshot below.
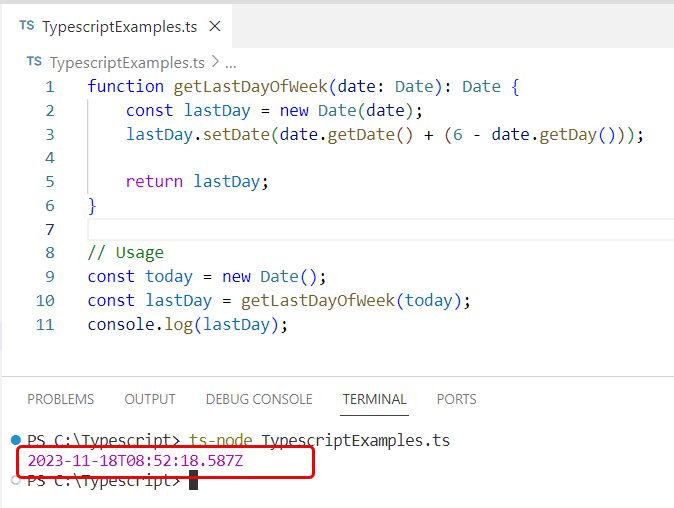
2. Moment.js
You can also use Moment.js to get the last day of the week in Typescript. The endOf(‘week’) method in Moment.js is used to get the end (Saturday) of the week.
Here is the complete code:
import moment from 'moment';
function getLastDayOfWeekUsingMoment(date: Date): Date {
return moment(date).endOf('week').toDate();
}
// Usage
const today = new Date();
const lastDay = getLastDayOfWeekUsingMoment(today);
console.log(lastDay);
3. Day.js
Day.js is a minimalistic library similar to Moment.js and can be used for getting the last day of week in Typescript. Day.js also provides an endOf(‘week’) method, similar to Moment.js, for reaching the end of the week.
Here is the complete code:
import dayjs from 'dayjs';
function getLastDayOfWeekUsingDayjs(date: Date): Date {
return dayjs(date).endOf('week').toDate();
}
// Usage
const today = new Date();
const lastDay = getLastDayOfWeekUsingDayjs(today);
console.log(lastDay);
Conclusion
TypeScript provides multiple ways to get the first day of the week from a given date. Whether using native JavaScript methods or libraries like Moment.js or Day.js. The same methods you can also use to get the last day of week from a given date in Typescript.
I hope you have a complete idea of how to get the first and last days of the week in Typescript using various methods.
You may also like:
- How to Get First and Last Day of Week in Typescript?
- How to create date from year month day in Typescript?
- How to Remove Time from Date in TypeScript?
- How to Calculate Date Difference in Days in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com