Many times, you might required to remove time from date in Typesctipt. In this Typescript, we show how to remove time from date in Typescript using various methods.
Remove Time from Date in TypeScript
Let us check out various methods to remove time from date in Typescript. Below are the three methods:
- Using toDateString()
- Setting Time to Zero
- Using Intl.DateTimeFormat
Remove time from date in Typescript using toDateString()
The toDateString() method is a straightforward way to extract only the date part from a Date object in TypeScript. This method converts the date to a more readable format without the time component. Here is the complete code to remove time from date in Typescript using toDateString().
function removeTimeFromDate(date: Date): string {
return date.toDateString();
}
// Example Usage
const currentDate = new Date();
console.log(removeTimeFromDate(currentDate));
Once you run the code using Visual Studio code, you can see the output in the screenshot below:
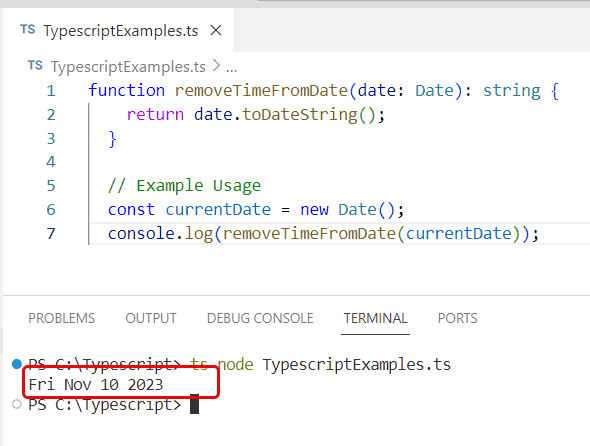
Remove time from date in Typescript by Setting Time to Zero
Another method to remove the time from a date in TypeScript is by setting the time components (hours, minutes, seconds, and milliseconds) to zero. Here is the complete code.
function removeTime(date: Date): Date {
date.setHours(0, 0, 0, 0);
return date;
}
// Example Usage
const today = new Date();
console.log(removeTime(today));
Remove time from date in Typescript using Intl.DateTimeFormat
In Typescript, you can use Intl.DateTimeFormat to remove time from date, and it is helpful for localization.
Here is the complete code.
function formatDate(date: Date): string {
return new Intl.DateTimeFormat('en-US').format(date);
}
// Example Usage
const someDate = new Date();
console.log(formatDate(someDate));
Once you run the code, you can see the output, like the screenshot below.
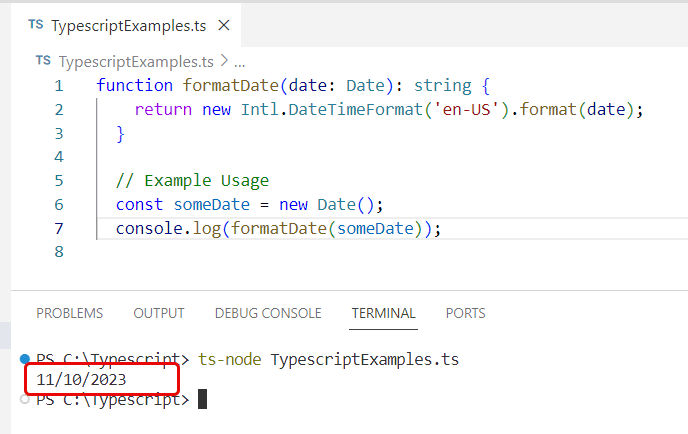
Conclusion
Removing the time from a date in TypeScript is a common requirement in many applications. By using either the toDateString() method, resetting the time to zero, or utilizing Intl.DateTimeFormat, you can easily manipulate date objects to remove time from date in typescript.
You may also like:
- TypeScript Date Add Days
- Calculate Date Difference in Days in Typescript
- Get month day year from date in Typescript
- Convert Date to String Format dd/mm/yyyy in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com