While working on a project, you might need to calculate the date difference in days in Typescript. In this tutorial, I have explained different methods to calculate the date difference in days using TypeScript.
To calculate the date difference in days using TypeScript, you can use methods like getTime(), which compares the time in milliseconds, or Date.UTC(), for a time zone-agnostic approach. Both methods involve converting dates into a comparable format and then dividing the difference by the number of milliseconds in a day, giving you an accurate day count.
Calculate Date Difference in Days in Typescript using getTime() Method
The getTime() method is a straightforward approach. It converts a date into the number of milliseconds since January 1, 1970. By finding the difference in milliseconds and converting it back to days, we get the desired result. This way, you can calculate the date difference in days in Typescript.
function differenceInDays(date1: Date, date2: Date): number {
const oneDay = 24 * 60 * 60 * 1000; // hours*minutes*seconds*milliseconds
const diffInTime = date2.getTime() - date1.getTime();
return Math.round(diffInTime / oneDay);
}
// Real-time example
const startDate = new Date('2024-01-19');
const endDate = new Date('2024-01-29');
console.log(differenceInDays(startDate, endDate));
Once I ran the code using Visual Studio code, you can see the date difference in days in Typescript in the screenshot below.
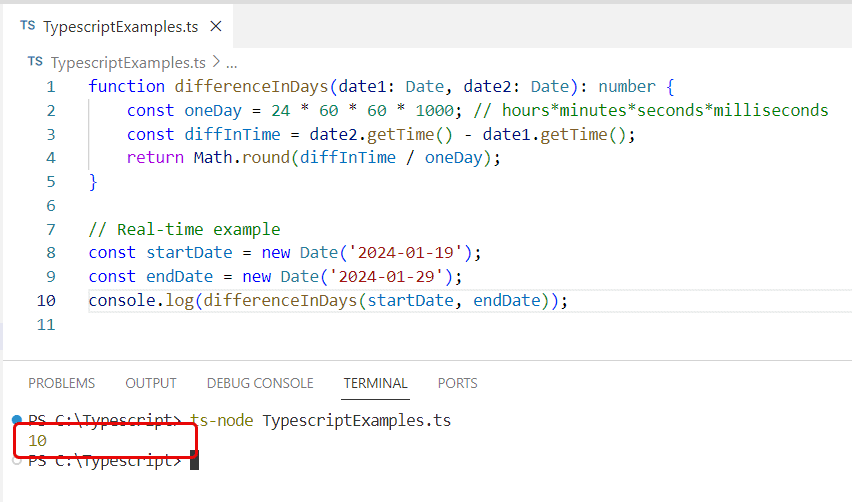
Calculate Date Difference in Days in Typescript using toISOString and Date Math
Another approach to calculating the date difference in days in Typescript is by converting the dates to ISO strings and then performing date arithmetic in Typescript. Here is a complete example with Typescript code.
function differenceInDaysISO(date1: Date, date2: Date): number {
const startDate = new Date(date1.toISOString().split('T')[0]);
const endDate = new Date(date2.toISOString().split('T')[0]);
return (endDate.valueOf() - startDate.valueOf()) / (1000 * 3600 * 24);
}
// Real-time example
const projectStart = new Date('2024-02-01');
const projectEnd = new Date('2024-02-15');
console.log(differenceInDaysISO(projectStart, projectEnd));
In this way, you can calculate the date difference in days in Typescript, like the screenshot below:
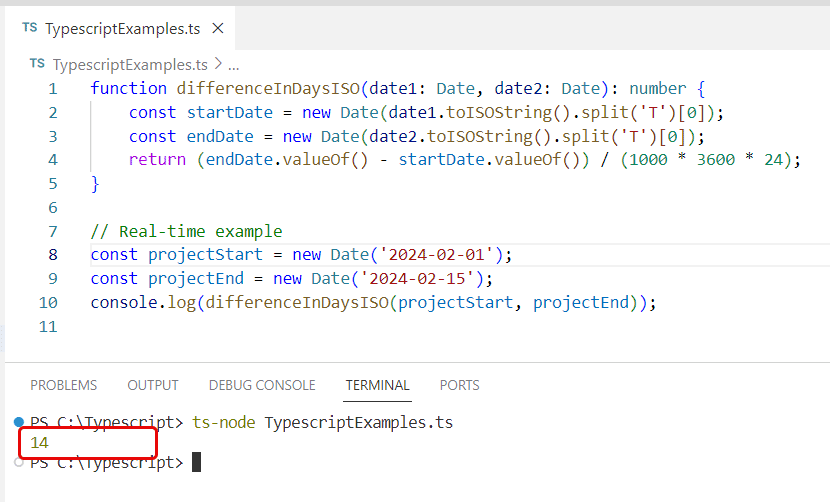
Calculate Date Difference in Days in Typescript using Date.UTC()
The Date.UTC() method treats the dates as UTC and calculates the difference, which can be useful for avoiding timezone-related issues. So, this is another way to Typescript date difference in days.
Here is a complete and real example.
function differenceInDaysUTC(date1: Date, date2: Date): number {
const diff = Date.UTC(date2.getFullYear(), date2.getMonth(), date2.getDate()) -
Date.UTC(date1.getFullYear(), date1.getMonth(), date1.getDate());
return diff / (1000 * 60 * 60 * 24);
}
// Real-time example
const eventStart = new Date('2024-05-01');
const eventEnd = new Date('2025-05-04');
console.log(differenceInDaysUTC(eventStart, eventEnd));
You can see the output below in the screenshot.
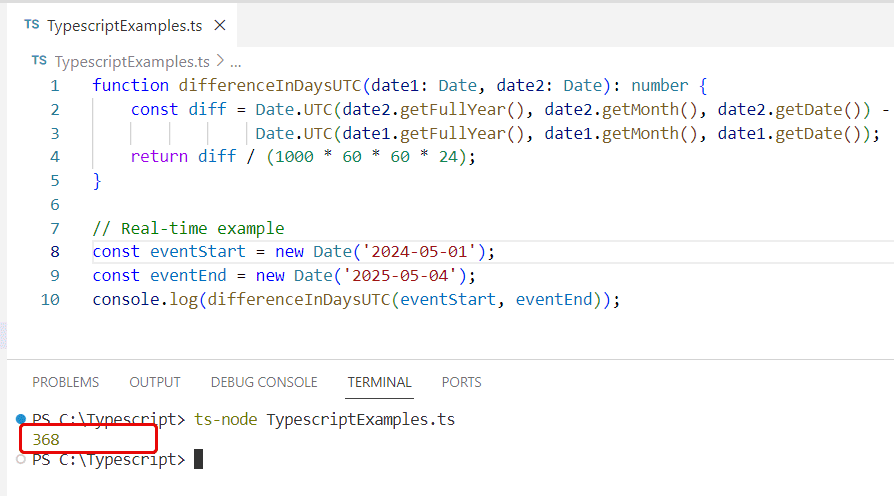
Conclusion
Calculating the date difference in days using TypeScript is a very common requirement in various applications. By using various Typescript methods like getTime(), toISOString, or Date.UTC(), developers can easily calculate the date difference in days in Typescript.
You may also like:
- Convert Date to String Format dd/mm/yyyy in Typescript
- TypeScript Date Add Days
- Remove Time from Date in TypeScript
- How to Format Date in Typescript
- Get month day year from date in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com