In this Typescript tutorial, I will explain to you how to get week number from date in Typescript.
A week number refers to the sequential numbering of the weeks in a year. The ISO 8601 standard is commonly used, where the first week of the year is the one that contains the first Thursday.
Get Week Number from Date in TypeScript
To get the week number from a date in Typescript, I will write a custom function that takes a Date object as input and returns the week number. Here is the complete code:
function getWeekNumber(date: Date): number {
// Copying date so the original date won't be modified
const tempDate = new Date(date.valueOf());
// ISO week date weeks start on Monday, so correct the day number
const dayNum = (date.getDay() + 6) % 7;
// Set the target to the nearest Thursday (current date + 4 - current day number)
tempDate.setDate(tempDate.getDate() - dayNum + 3);
// ISO 8601 week number of the year for this date
const firstThursday = tempDate.valueOf();
// Set the target to the first day of the year
// First set the target to January 1st
tempDate.setMonth(0, 1);
// If this is not a Thursday, set the target to the next Thursday
if (tempDate.getDay() !== 4) {
tempDate.setMonth(0, 1 + ((4 - tempDate.getDay()) + 7) % 7);
}
// The weeknumber is the number of weeks between the first Thursday of the year
// and the Thursday in the target week
return 1 + Math.ceil((firstThursday - tempDate.valueOf()) / 604800000); // 604800000 = number of milliseconds in a week
}
// Example usage
const currentDate = new Date();
console.log(`Week number: ${getWeekNumber(currentDate)}`);
Output:
Week number: 45
You can see the above Typescript code is giving me the week number based on today’s date, check the screenshot below:
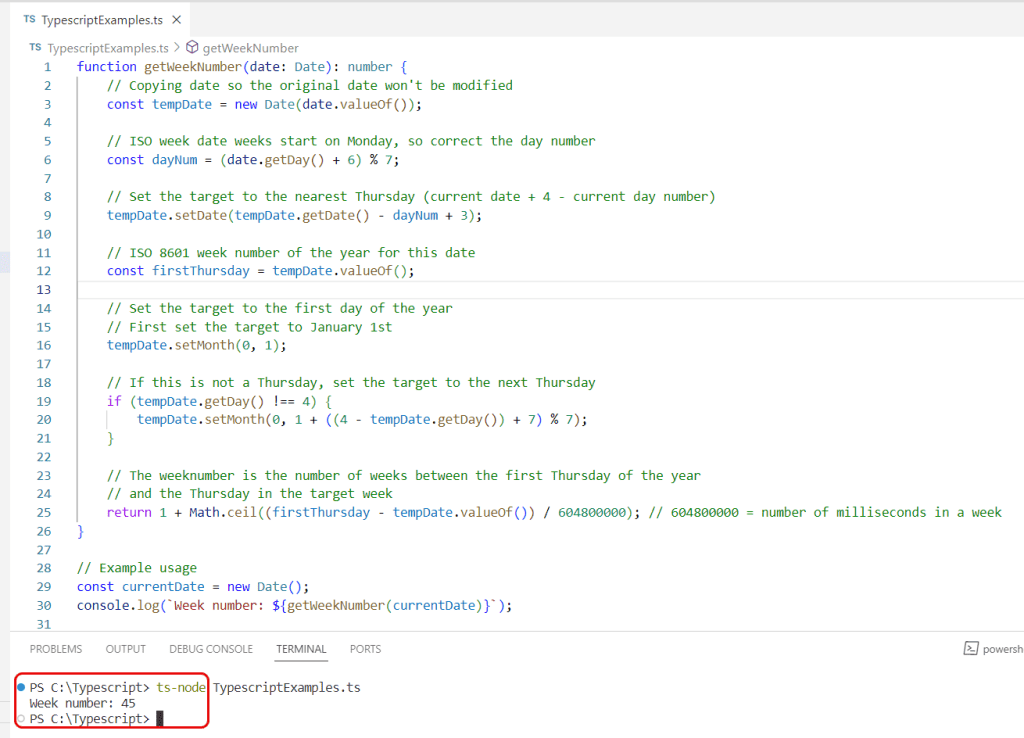
Conclusion
Extracting the week number from a date in TypeScript is a straightforward process once you understand the logic behind the ISO 8601 week date system. I hope now you have an idea of how to get the week number from the date in Typescript.
You may also like:
- How to create date from year month day in Typescript?
- How to Get First and Last Day of Week in Typescript?
- TypeScript Date Add Days
- How to Calculate Date Difference in Days in Typescript?
- How to Remove Time from Date in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com