Are you looking for “Typescript date add days”? In this Typescript tutorial, I will explain to you how to add days to a date in Typescript. Also, we will cover various methods to add days, months, hours, minutes, and seconds to a date in TypeScript.
To add days to a date in TypeScript, utilize the Date object’s setDate() and getDate() methods. You can easily compute a future date by creating a new Date instance and adjusting it with the desired number of days. This approach is versatile, allowing you to add any number of days to a given date straightforwardly and efficiently.
TypeScript Add Days to Date
To add days to a date in TypeScript, you can use the setDate() and getDate() methods of the Date object. Here’s how you can do it with the below complete code:
function addDays(date: Date, days: number): Date {
let result = new Date(date);
result.setDate(result.getDate() + days);
return result;
}
// Example: Adding 5 days to the current date
const currentDate = new Date();
console.log("Current Date: ", currentDate);
const newDate = addDays(currentDate, 5);
console.log("Date After Adding 5 Days: ", newDate);
The output, after I ran the code using Visual Studio Code, is shown in the screenshot below: It adds 5 days to the current date in Typescript.
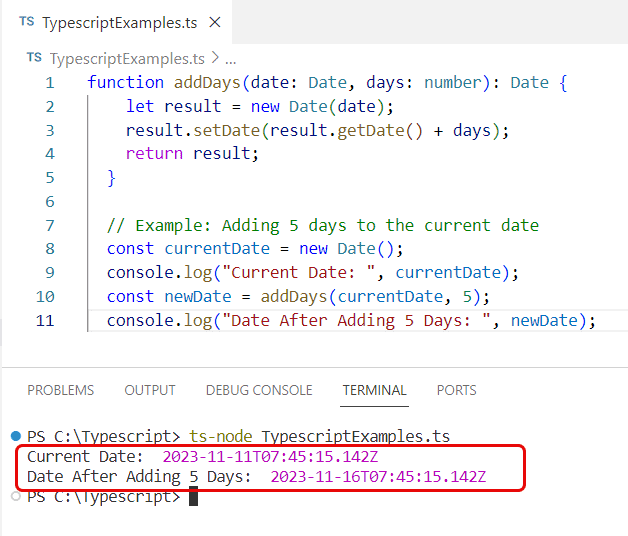
TypeScript Add 30 Days to Date
If you want to add a specific number of days, like you want to add 30 days to a date in Typescript, here is how you can do it.
function addDays(date: Date, days: number): Date {
let result = new Date(date);
result.setDate(result.getDate() + days);
return result;
}
// Example: Adding 5 days to the current date
const currentDate = new Date();
console.log("Current Date: ", currentDate);
const dateAfter30Days = addDays(currentDate, 30);
console.log("Date After Adding 30 Days: ", dateAfter30Days);
For the output, you can see the screenshot below, which I got after executing the code using VS code.
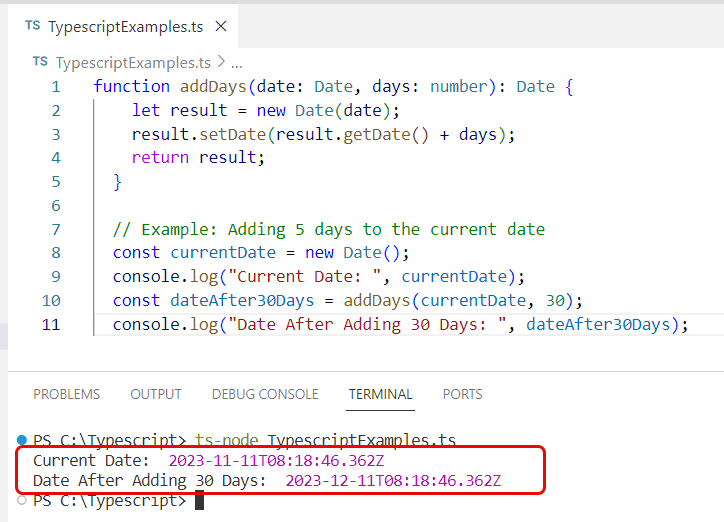
TypeScript Add Months to Date
If you want to add months to date in Typescript, you can use setMonth() and getMonth() methods. Here is a complete code for adding months to a date in Typescript.
function addMonths(date: Date, months: number): Date {
let result = new Date(date);
result.setMonth(result.getMonth() + months);
return result;
}
const currentDate = new Date();
console.log("Current Date: ", currentDate);
// Example: Adding 2 months to the current date
const dateAfter2Months = addMonths(currentDate, 2);
console.log("Date After Adding 2 Months: ", dateAfter2Months);
Check the screenshot below, it is showing the date after adding 2 months to current date in Typescript.
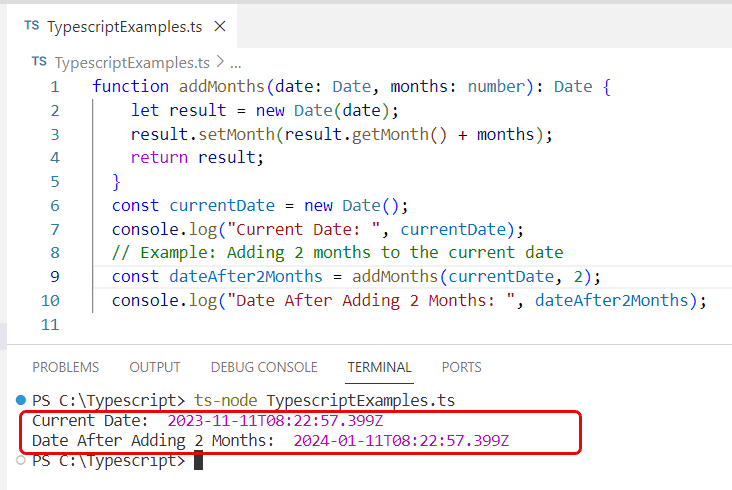
TypeScript Add Hours to Date
To add hours to date in Typescript, you can utilize the setHours() and getHours() methods. Here is the complete code:
function addHours(date: Date, hours: number): Date {
let result = new Date(date);
result.setHours(result.getHours() + hours);
return result;
}
const currentDate = new Date();
console.log("Current Date: ", currentDate);
// Example: Adding 3 hours to the current date
const dateAfter3Hours = addHours(currentDate, 3);
console.log("Date After Adding 3 Hours: ", dateAfter3Hours);
You can see here in the below screenshot, I have added 3 hours to current date in Typescript.
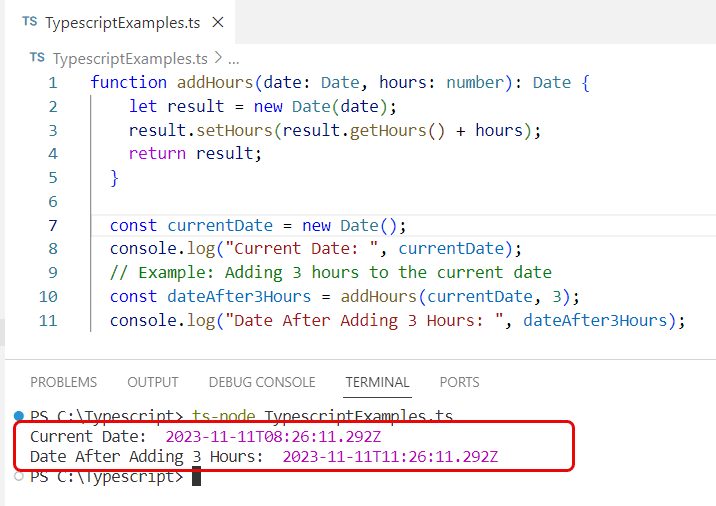
TypeScript Add Minutes to Date
Similarly, for adding minutes to date in Typescript, use setMinutes() and getMinutes() methods like below:
function addMinutes(date: Date, minutes: number): Date {
let result = new Date(date);
result.setMinutes(result.getMinutes() + minutes);
return result;
}
const currentDate = new Date();
console.log("Current Date: ", currentDate);
// Example: Adding 45 minutes to the current date
const dateAfter45Minutes = addMinutes(currentDate, 45);
console.log("Date After Adding 45 Minutes: ", dateAfter45Minutes);
Check out the below screenshot after adding minutes to current date in Typescript.
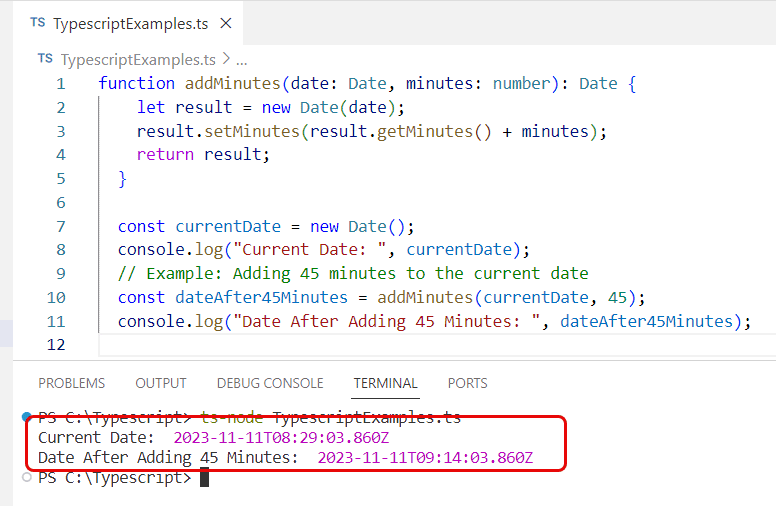
TypeScript Add Seconds to Date
If you want to add seconds to date in Typescript, you can use the setSeconds() and getSeconds() methods in Typescript.
function addSeconds(date: Date, seconds: number): Date {
let result = new Date(date);
result.setSeconds(result.getSeconds() + seconds);
return result;
}
const currentDate = new Date();
console.log("Current Date: ", currentDate);
// Example: Adding 30 seconds to the current date
const dateAfter30Seconds = addSeconds(currentDate, 30);
console.log("Date After Adding 30 Seconds: ", dateAfter30Seconds);
After I executed the Typescript code using VS code, you can see the output like in the below screenshot.
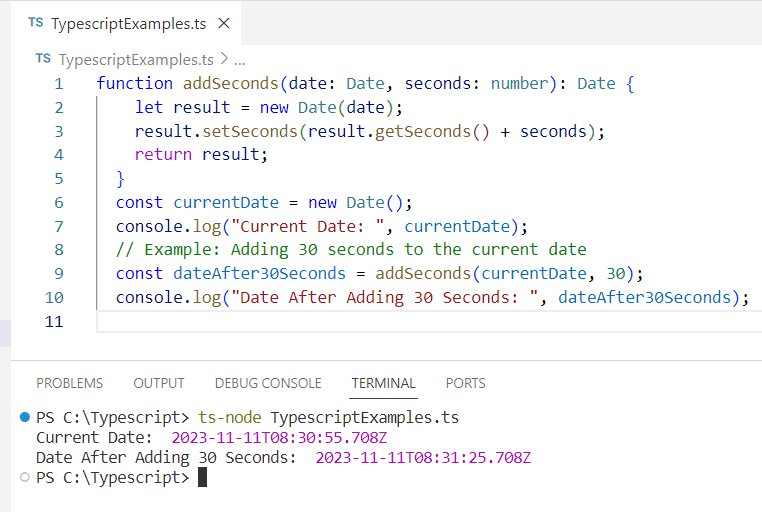
Conclusion
Managing dates in TypeScript is made efficient through the use of the Date object’s methods. By understanding and applying functions like setDate(), getDate(), setMonth(), getMonth(), setHours(), getHours(), setMinutes(), and setSeconds(), you can effortlessly add days, months, hours, minutes, and seconds to any date in Typescript. Here, we cover with practical examples:
- Add days to date in Typescript
- Add 30 days to date in Typescript
- How to add months to date in Typescript
- Add hours to date in Typescript
- Add minutes to date in Typescript
- How to add seconds to date in Typescript
You may also like:
- Calculate Date Difference in Days in Typescript
- How to Remove Time from Date in TypeScript?
- Typescript Date Format
- Get month day year from date in Typescript
- Convert Date to String Format dd/mm/yyyy in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com