One of my team members recently searched for a way to format a date in Typescript. As a Typescript developer, this is a very common requirement when working with Typescript dates. There are various methods to do this. In this tutorial, I will explain everything about the Typescript date format with examples.
Typescript Date Format
TypeScript, being a superset of JavaScript, inherits all the date handling capabilities of JavaScript. This means you can use JavaScript’s Date
object and its methods in TypeScript.
Before checking into formatting, let’s start by creating a Date
object. Here’s how you can do it:
const currentDate: Date = new Date();
console.log(currentDate);
This will output the current date and time. But what if you need to format this date in a specific way?
Typescript provides different methods to format a date.
1. Format Dates Using toLocaleDateString()
The toLocaleDateString()
method in Typescript is a powerful way to format dates according to the locale. It allows you to customize the output format easily.
Syntax
Here is the syntax:
date.toLocaleDateString(locales?: string | string[], options?: Intl.DateTimeFormatOptions): string;
Example
Let me show you an example, where we can format a date to display in the US format (MM/DD/YYYY) using the below Typescript code.
const date: Date = new Date('2025-10-15T10:20:30Z');
const formattedDate: string = date.toLocaleDateString('en-US');
console.log(formattedDate); // Output: 10/15/2025
I executed the above Typescript code using VS code, and you can see the exact output in the screenshot below:
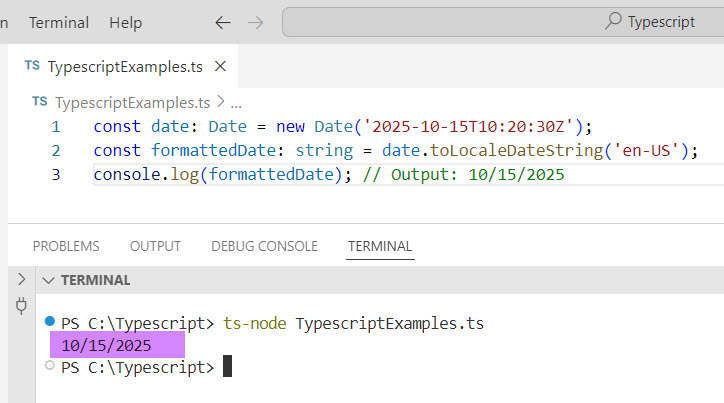
Customize the Format
You can also customize the format using the options
parameter. Here is the complete code.
const date: Date = new Date('2025-10-15T10:20:30Z');
const options: Intl.DateTimeFormatOptions = { year: 'numeric', month: 'long', day: '2-digit' };
const customFormattedDate: string = date.toLocaleDateString('en-US', options);
console.log(customFormattedDate); // Output: October 15, 2025
Check out Convert String to Date in TypeScript
2. Using toLocaleTimeString()
If you need to format the time part of a date, toLocaleTimeString()
is your go-to method in Typescript.
Syntax
Here is the syntax:
date.toLocaleTimeString(locales?: string | string[], options?: Intl.DateTimeFormatOptions): string;
Example
Here is an example. Let’s format the time to display in the US format:
const date: Date = new Date('2025-10-15T10:20:30Z');
const time: string = date.toLocaleTimeString('en-US');
console.log(time); // Output: 3:20:30 AM
Customize the Time Format
You can also customize the time format; using the below Typescript code.
const date: Date = new Date('2025-10-15T10:20:30Z');
const timeOptions: Intl.DateTimeFormatOptions = { hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true };
const customFormattedTime: string = date.toLocaleTimeString('en-US', timeOptions);
console.log(customFormattedTime); // Output: 03:20:30 AM
Check out Typescript sort array by date
3. Using toLocaleString()
For a combined date and time format, toLocaleString()
is very useful in Typescript.
Syntax
Here is the syntax:
date.toLocaleString(locales?: string | string[], options?: Intl.DateTimeFormatOptions): string;
Example
Here is an example.
Format both date and time:
const date: Date = new Date('2025-10-15T10:20:30Z');
const dateTime: string = date.toLocaleString('en-US');
console.log(dateTime); // Output: 10/15/2025, 3:20:30 AM
Customize Date and Time
Customize both date and time:
const date: Date = new Date('2025-10-15T10:20:30Z');
const dateTimeOptions: Intl.DateTimeFormatOptions = {
year: 'numeric', month: 'short', day: '2-digit',
hour: '2-digit', minute: '2-digit', second: '2-digit', hour12: true
};
const customFormattedDateTime: string = date.toLocaleString('en-US', dateTimeOptions);
console.log(customFormattedDateTime); // Output: Oct 15, 2025, 03:20:30 AM
4. Parse and Format with date-fns
The date-fns library is a great choice for more advanced data manipulation and formatting. It provides a wide range of functions for working with dates in a functional style.
Example
First, install date-fns
:
npm install date-fns
Then, use it in your TypeScript code:
import { format } from 'date-fns';
const date: Date = new Date('2025-10-15T10:20:30Z');
const formattedDate: string = format(date, 'MM/dd/yyyy');
console.log(formattedDate); // Output: 10/15/2025
Now, let me show you some real examples of Typescript date formats.
Check out Convert Date to UTC in Typescript
With the complete Typescript code, I will show the below examples:
- Typescript date format dd-mm-yyyy
- Typescript date format yyyy-mm-dd
- Typescript format date mm/dd/yyyy with time
- Typescript format date mm/dd/yyyy
You can use the Date object to format date and time in Typescript. By using various formatting options, you can display the date in Typescript. Here are a few examples with the complete code.
TypeScript Date Format dd-mm-yyyy
If you want to format a date in dd-mm-yyyy in Typescript, check the Typescript code below.
The DD-MM-YYYY format is widely used in many countries and is often preferred for its clarity. In TypeScript, formatting a date to this style involves converting the date object into a string and rearranging its components.
Here is a complete example of formatting date in dd-mm-yyyy in Typescript.
function formatDate(date: Date): string {
const day = date.getDate().toString().padStart(2, '0');
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const year = date.getFullYear();
return `${day}-${month}-${year}`;
}
const today = new Date();
console.log(formatDate(today));
Here, you can see the output after I ran the code using Visual Studio code.
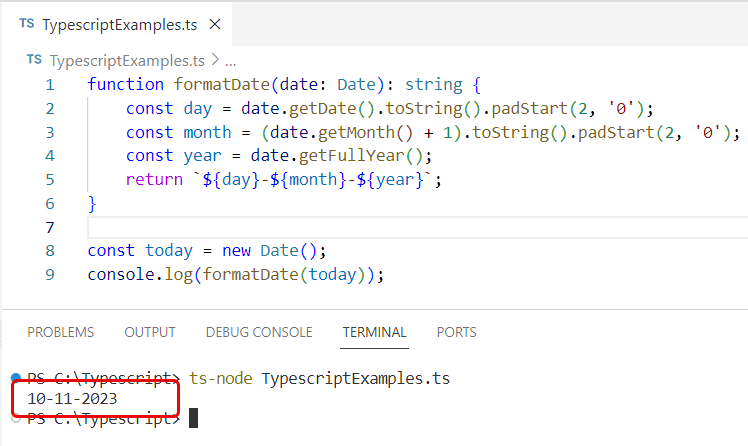
Read How to Format Date with Timezone in Typescript?
TypeScript Date Format yyyy-mm-dd
The YYYY-MM-DD format is ISO standard and is often used in various Typescript programs.
You can use the below code if you want to format the date in yyyy-mm-dd format in Typescript.
function formatISODate(date: Date): string {
const year = date.getFullYear();
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const day = date.getDate().toString().padStart(2, '0');
return `${year}-${month}-${day}`;
}
const today = new Date();
console.log(formatISODate(today));
The output is as shown in the screenshot below.
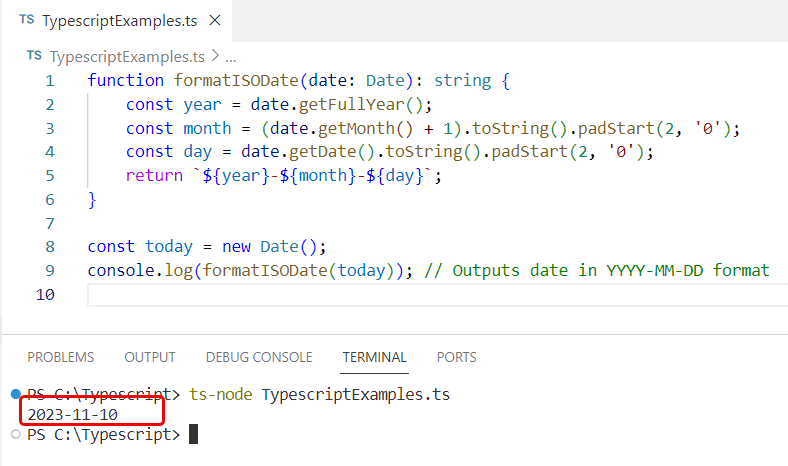
TypeScript Format Date mm/dd/yyyy with time
Sometimes, we need not just the date but also the time. The MM/DD/YYYY with time format is particularly useful in scenarios where you want to get the date with time in Typescript.
Here is the complete code to format a date in mm/dd/yyyy with time in Typescript.
function formatDate(date: Date): string {
const day = date.getDate().toString().padStart(2, '0');
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const year = date.getFullYear();
return `${day}-${month}-${year}`;
}
function formatDateTime(date: Date): string {
const formattedDate = formatDate(date); // Reuse formatDate function
const hours = date.getHours().toString().padStart(2, '0');
const minutes = date.getMinutes().toString().padStart(2, '0');
const seconds = date.getSeconds().toString().padStart(2, '0');
return `${formattedDate} ${hours}:${minutes}:${seconds}`;
}
const now = new Date();
console.log(formatDateTime(now));
I also reused the formatDate function here. The output is shown in the screenshot below; I ran the code using VS code.
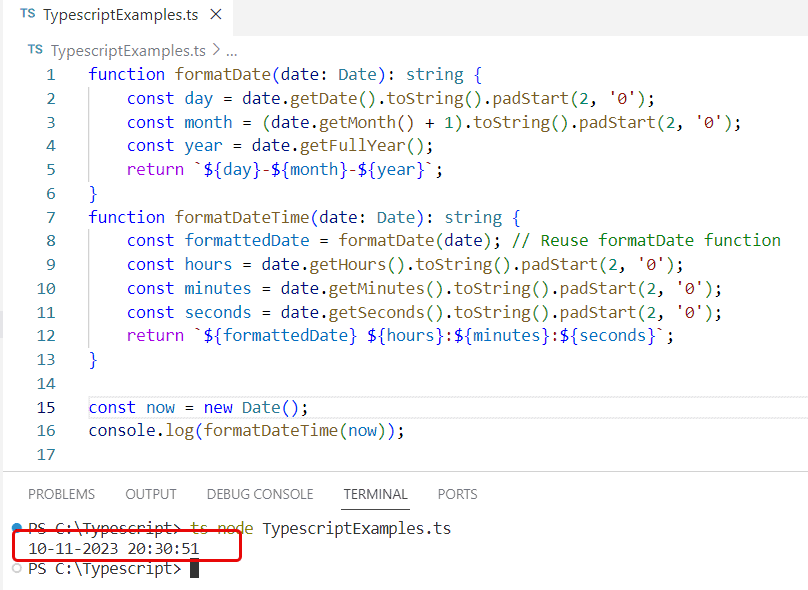
Check out Check Date Less Than Today in Typescript
TypeScript Date Format mm/dd/yyyy
The MM/DD/YYYY format is predominantly used in the United States and is familiar to a wide audience. Formatting dates in this style in TypeScript is straightforward and efficient and below is the complete code.
function formatUSDate(date: Date): string {
const month = (date.getMonth() + 1).toString().padStart(2, '0');
const day = date.getDate().toString().padStart(2, '0');
const year = date.getFullYear();
return `${month}/${day}/${year}`;
}
const today = new Date();
console.log(formatUSDate(today));
Here is the output of the above typescript code to format the date in mm/dd/yyyy format in Typescript.
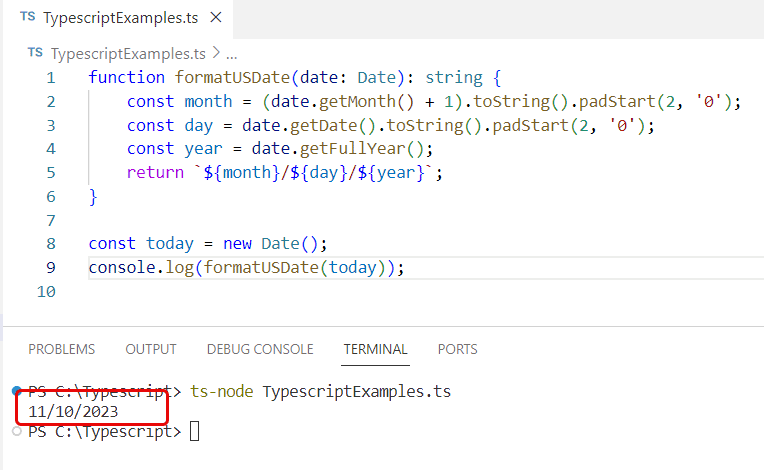
Conclusion
In this Typescript tutorial, I have explained how to format a date in Typescript, and then we also cover the below Typescript date format examples:
- TypeScript Date Format dd-mm-yyyy
- TypeScript Date Format yyyy-mm-dd
- TypeScript Format Date mm/dd/yyyy with time
- TypeScript Date Format mm/dd/yyyy
You may also like the following tutorials:
- Check If Date is Valid in Typescript
- Subtract Days from Current Date in Typescript
- How to create date from year month day in Typescript?
- Get First and Last Day of Week in Typescript
- Get Week Number from Date in TypeScript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com