In this Typescript tutorial, I will explain how to convert date to UTC in Typescript using various methods.
To convert a date to UTC in TypeScript, you can use the JavaScript Date object’s toISOString() method for quick conversion. Alternatively, for more control, manually construct a UTC date using Date.UTC(). Libraries like moment.js or date-fns are also excellent for handling more complex date conversions.
Convert Date to UTC in Typescript using the Date object
The easiest way to convert date to UTC format in Typescript is using the Date object. Here is a complete code:
function convertToUTC(date: Date): string {
return date.toISOString();
}
const localDate = new Date();
const utcDate = convertToUTC(localDate);
console.log(utcDate);
This function takes a Date object and converts it to the ISO string format, which is inherently in UTC.
You can see the output in the screenshot below:
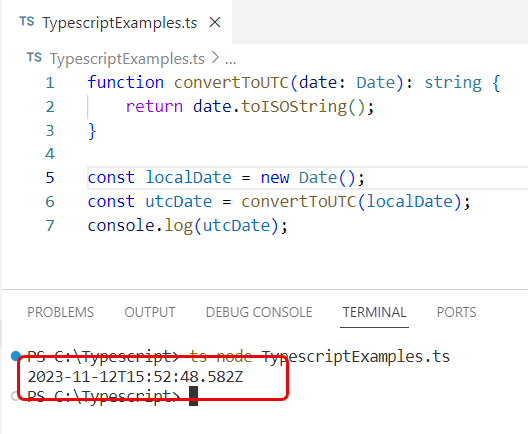
Convert Date to UTC in Typescript using Manual Conversion
You can also convert the date to UTC in Typescript using manual conversion. Here is the complete code:
function manualConvertToUTC(date: Date): Date {
return new Date(Date.UTC(date.getFullYear(), date.getMonth(), date.getDate(), date.getHours(), date.getMinutes(), date.getSeconds()));
}
const localDate = new Date();
const utcDate = manualConvertToUTC(localDate);
console.log(utcDate);
You can see the below screenshot.
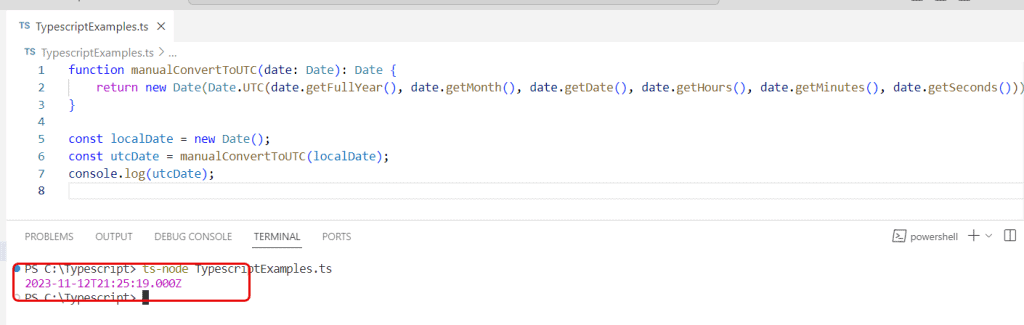
Typescript convert date to UTC using various libraries
For more complex applications, you might consider using a library like moment.js or date-fns to convert date to UTC in Typescript.
Using moment.js:
You can use the moment.js library to convert a date to UTC in Typescript. Here is the complete code:
import moment from 'moment';
function convertUsingMoment(date: Date): string {
return moment(date).utc().format();
}
const localDate = new Date();
const utcDate = convertUsingMoment(localDate);
console.log(utcDate);
Using date-fns:
Here is the complete code to convert a date to UTC in Typescript using date-fns library. Here is the complete code:
import { formatUTC } from 'date-fns';
const localDate = new Date();
const utcDate = formatUTC(localDate, 'yyyy-MM-dd HH:mm:ssX');
console.log(utcDate);
Conclusion
Various methods can be used to convert dates to UTC in Typescript, such as using the Date object with third-party libraries like moment.js, date-fns, etc.
You may also like:
- Typescript sort array of objects by date descending
- How to Format Date with Timezone in Typescript?
- How to Check Date Less Than Today in Typescript?
- How to Check If Date is Valid in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com