Do you want to format date with timezone in Typescript? In this Typescript tutorial, I will explain how to formate date with timezone in Typescript using various methods.
To format dates with timezones in TypeScript, you can use the native JavaScript Date object for basic formatting or the Intl.DateTimeFormat for specific timezones. For more complex scenarios, libraries like date-fns and moment-timezone offer extensive functionalities, allowing precise control over timezone conversions and formatting in a TypeScript-based application.
Typescript format date with timezone
In TypeScript, you can format dates using the native Date object. Here’s a simple example:
const formatDate = (date: Date): string => {
return date.toISOString();
};
const currentDate = new Date();
console.log(formatDate(currentDate));
To format dates with specific timezones, use the Intl.DateTimeFormat API. This API is part of the ECMAScript internationalization API and works seamlessly in TypeScript.
const formatDateWithTimezone = (date: Date, timezone: string): string => {
return new Intl.DateTimeFormat('en-US', {
timeZone: timezone,
dateStyle: 'full',
timeStyle: 'long',
}).format(date);
};
console.log(formatDateWithTimezone(new Date(), 'America/New_York'));
Once you run the code, you can see the output in the below screenshot.
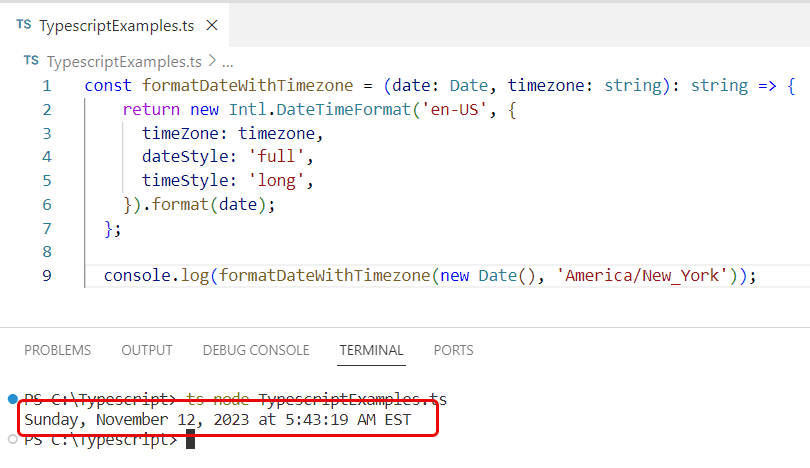
Using a library like date-fns or moment-timezone is recommended for more complex date manipulations and formatting in Typescript. These libraries offer extensive functionalities to work with dates and time zones.
Using date-fns
date-fns is a lightweight library for date manipulation. It provides functions to format dates, which you can use with time zones.
import { format, utcToZonedTime } from 'date-fns-tz';
const formatZonedDate = (date: Date, timezone: string): string => {
const zonedDate = utcToZonedTime(date, timezone);
return format(zonedDate, 'yyyy-MM-dd HH:mm:ssXXX', { timeZone: timezone });
};
console.log(formatZonedDate(new Date(), 'America/Los_Angeles'));
Using moment-timezone
moment-timezone is another popular library that extends Moment.js with timezone support.
import moment from 'moment-timezone';
const formatMomentDateWithTimezone = (date: Date, timezone: string): string => {
return moment(date).tz(timezone).format('YYYY-MM-DD HH:mm:ss Z');
};
console.log(formatMomentDateWithTimezone(new Date(), 'America/Chicago'));
Conclusion
Handling dates and times with respect to different timezones is a crucial aspect of web development. TypeScript, with its strong typing and support for JavaScript libraries, provides multiple ways to format dates. Whether you’re using the native JavaScript Date object, the Intl.DateTimeFormat API, or libraries like date-fns and moment-timezone, Typescript provides various methods. I hope you have an idea now on how to format date with timezone in Typescript.
You may also like:
- Typescript sort array of objects by date descending
- How to Convert Date to UTC in Typescript?
- How to Check Date Less Than Today in Typescript?
- How to Check If Date is Valid in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com