Recently, while working with strings in TypeScript, I got a requirement to capitalize the first letter of a string. There are various methods to achieve this. In this tutorial, I will explain several methods to capitalize the first letter of a string in TypeScript with examples.
To capitalize the first letter in TypeScript, you can use the charAt()
and toUpperCase()
methods. First, retrieve the first character of the string using charAt(0)
, convert it to uppercase with toUpperCase()
, and then concatenate it with the rest of the string using slice(1)
. For example:
function capitalizeFirstLetter(str: string): string {
return str.charAt(0).toUpperCase() + str.slice(1);
}
This function ensures that the initial character is capitalized while leaving the rest of the string unchanged.
Typescript Capitalize First Letter
There are various methods for making the first letter of a string in Typescript uppercase. Let me explain each method with examples.
Basic Syntax for Uppercase First Letter in Typescript
In TypeScript, capitalizing the first letter of a string involves a few simple steps: extracting the first character, converting it to uppercase, and then concatenating it with the rest of the string. Here’s a basic example:
function capitalizeFirstLetter(str: string): string {
return str.charAt(0).toUpperCase() + str.slice(1);
}
Also, check out Split String by Length in Typescript
Method 1: Using charAt() and toUpperCase()
In Typescript, you can use the charAt() and toUpperCase() methods to convert first letter uppercase in a string.
The charAt()
method returns the character at a specified index, while toUpperCase()
converts a string to uppercase in Typescript. This method is easy to understand and use.
Let me show you an example.
Example
function capitalizeFirstLetter(name: string): string {
return name.charAt(0).toUpperCase() + name.slice(1);
}
console.log(capitalizeFirstLetter("new york")); // Output: New york
console.log(capitalizeFirstLetter("canada")); // Output: Canada
In this example, charAt(0)
retrieves the first character of the string, and toUpperCase()
converts it to uppercase. The slice(1)
method then extracts the rest of the string from the second character onward, which is concatenated back to the uppercase first letter.
I executed the above code using VS code; it returns the exact output. Below is the screenshot for your reference.
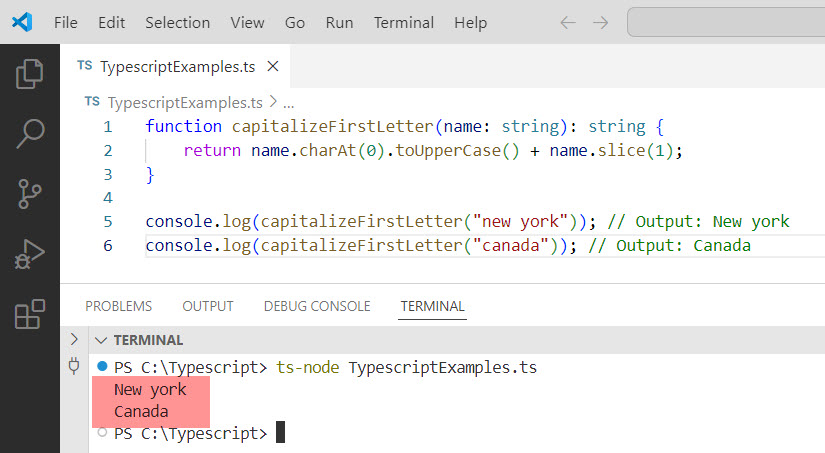
Read Convert String to Enum in Typescript
Method 2: Using Template Literals
Template literals provide a more modern way to handle string interpolation in TypeScript. This method can be used to capitalize the first letter in a string in Typescript.
Example
Here is an example.
function capitalizeFirstLetter(name: string): string {
return `${name.charAt(0).toUpperCase()}${name.slice(1)}`;
}
console.log(capitalizeFirstLetter("andrew")); // Output: Andrew
console.log(capitalizeFirstLetter("jessica")); // Output: Jessica
Using template literals, we achieve the same result with a more concise and readable syntax. This method is especially useful when dealing with more complex string manipulations.
Once you execute the above Typescript code, you can see the exact output in the screenshot below:
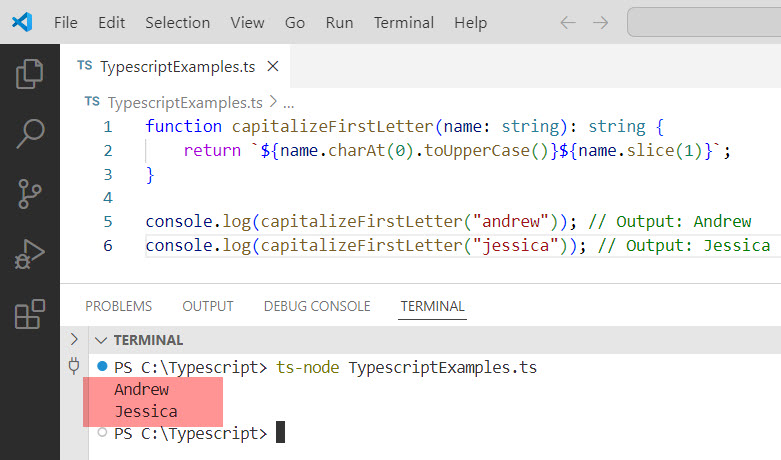
Check out Split String By New Line in Typescript
Method 3: Using substring() and toUpperCase()
The substring()
method can also be used to make first letter uppercase in Typescript. It extracts parts of a string and returns the new substring. This method is similar to slice()
but offers a slightly different syntax.
Example
Let me show you an example to help you understand better.
function capitalizeFirstLetter(city: string): string {
return city.substring(0, 1).toUpperCase() + city.substring(1);
}
console.log(capitalizeFirstLetter("chicago")); // Output: Chicago
console.log(capitalizeFirstLetter("miami")); // Output: Miami
In this example, substring(0, 1)
extracts the first character, and substring(1)
extracts the rest of the string from the second character onward. This method is functionally equivalent to using slice()
.
Here is the output in the screenshot below:
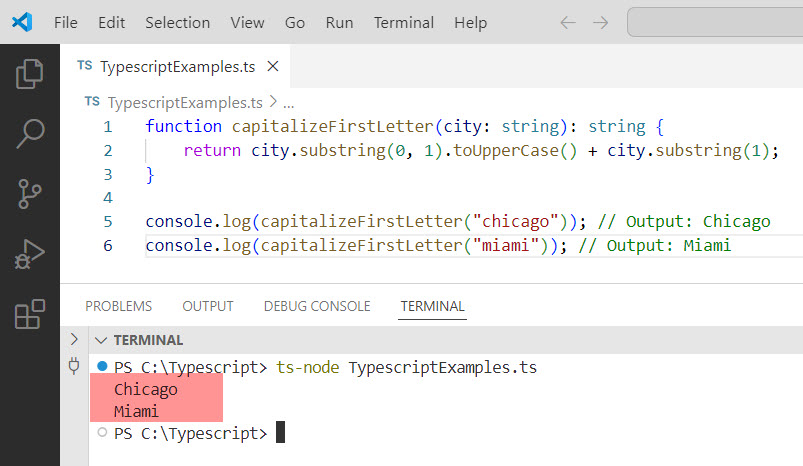
Read Convert String to Date in TypeScript
Method 4: Using Regular Expressions
Regular expressions offer a powerful way to perform complex string manipulations in Typescript. This method is slightly more advanced but very efficient, especially for more complex string transformations.
Let me show you how we can use regular expressions to capitalize first letter in Typescript.
Example
Here is an example.
function capitalizeFirstLetter(state: string): string {
return state.replace(/^\w/, (c) => c.toUpperCase());
}
console.log(capitalizeFirstLetter("california")); // Output: California
console.log(capitalizeFirstLetter("texas")); // Output: Texas
In this example, the regular expression /^\w/
matches the first word character in the string. The replace()
method then replaces this character with its uppercase equivalent using a callback function. This method is particularly useful when performing more complex or bulk string transformations.
I executed the above code, and you can see the exact output in the screenshot below:
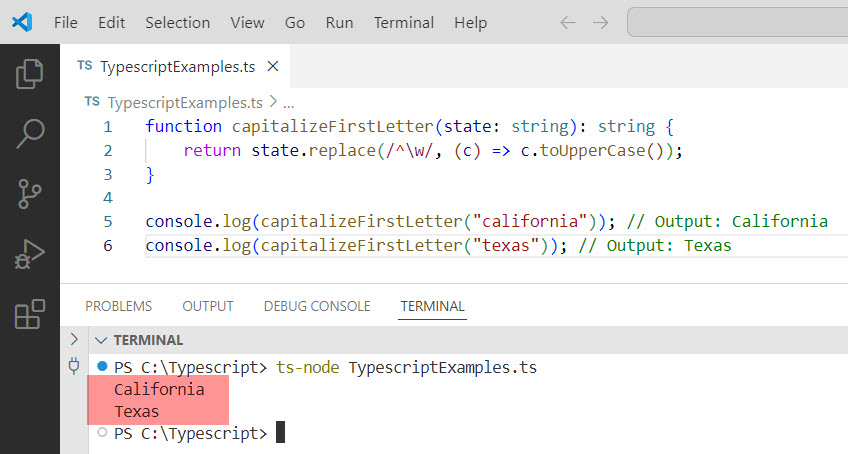
Read Convert String to Number in TypeScript
Method 5: Using TypeScript’s Capitalize Utility Type
TypeScript provides a built-in utility type called Capitalize
that can capitalize the first letter of string literal types. However, it’s important to note that this works at the type level and not at runtime.
Example
Here is an example.
type CapitalizedName = Capitalize<"john">; // Type is "John"
type CapitalizedCity = Capitalize<"boston">; // Type is "Boston"
The Capitalize
utility type is useful for ensuring that string literals are correctly capitalized at the type level. This can be particularly beneficial in type-safe applications where you want to enforce specific string formats.
Conclusion
In this tutorial, I explained several methods to capitalize the first letter of a string in TypeScript, such as charAt()
and toUpperCase()
, template literals, regular expressions, etc.
I executed all the examples using VS code, and I hope all the code works for you, too. Do let me know in the comments if you face any issues.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com