Do you need to split string by length in Typescript? In this Typescript tutorial, I will explain how to split a string by length in Typescript using various methods.
There are various methods to split a string by length in Typescript:
- Using custom function
- Using regular expression
- Using Array.from() Method, etc.
1. Using a Custom Function
One way to split a string by length in TypeScript is to write a custom function that loops through the string and extracts substrings of the desired length. Here’s a simple function that does just that:
function chunkString(str: string, length: number): string[] {
const chunks: string[] = [];
let index = 0;
while (index < str.length) {
chunks.push(str.substring(index, index + length));
index += length;
}
return chunks;
}
// Example usage:
const result = chunkString("United States of America", 3);
console.log(result);
In this example, the chunkString
function takes two parameters: the string to split (str
) and the length of each chunk (length
). It uses a while loop to go through the string, and the substring
method to extract chunks of the specified size.
After I executed the code using VS code, you can see the output in the screenshot below:
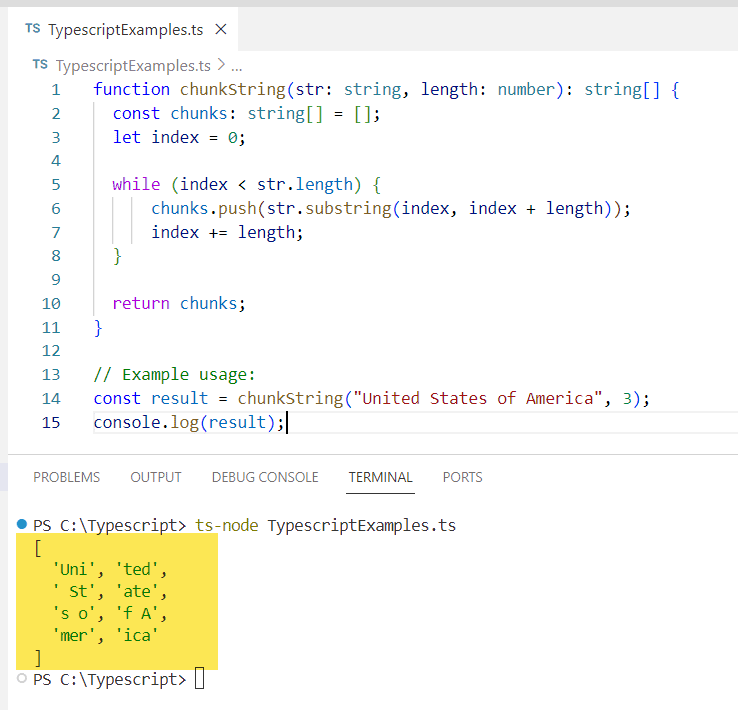
2. Using Regular Expressions
Another approach to splitting a string by length in Typescript is to use regular expressions. Here’s an example of how you can use a regular expression to achieve the same result:
function splitStringByLength(str: string, length: number): string[] {
const regex = new RegExp(`.{1,${length}}`, 'g');
return str.match(regex) || [];
}
// Example usage:
const result = splitStringByLength("UnitedStatesOfAmerica", 4);
console.log(result);
The regular expression .{1,${length}}
matches any character (.
) between 1 and length
times, and the g
flag indicates that the match should be performed globally across the entire string. The match
method then extracts all matches into an array.
You can see the output in the screenshot below after I executed the code using Visual Studio code:
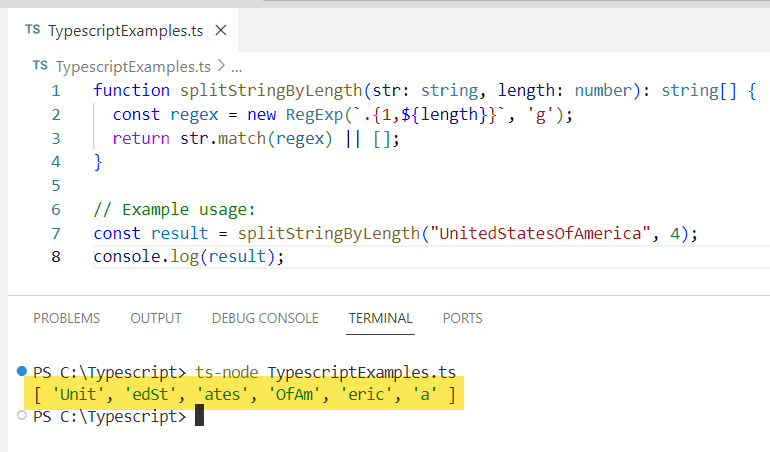
3. Using Array.from() Method
If you want to use a more functional programming approach, you can utilize the Array.from()
method to split a string into chunks of equal length in Typescript. Here’s how you can do it:
function splitIntoChunks(str: string, length: number): string[] {
return Array.from(
{ length: Math.ceil(str.length / length) },
(_, i) => str.substring(i * length, (i + 1) * length)
);
}
// Example usage:
const result = splitIntoChunks("HelloTypeScript", 5);
console.log(result); // Output: ['Hello', 'TypeS', 'cript']
In this function, Array.from()
is used to create a new array with a length equal to the number of chunks. The second argument is a mapping function that takes the index (i
) and returns a substring of the appropriate length.
Conclusion
Splitting a string by length in TypeScript is possible by using a custom function, regular expressions, or the Array.from()
method. I hope now you have an idea of how to split a string by length in Typescript.
You may also like:
- How To Split String And Get Last Element In Typescript?
- Split String by Regex in TypeScript
- TypeScript Split String Into Array
- How to Split String By New Line in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com