Do you want to split a string and get the last element in Typescript? In this Typescript tutorial, I will explain with examples “Typescript split string and get last” with complete code.
To split a string and get the last element in TypeScript, use the split() method to divide the string by a specified separator, creating an array of substrings. Then, access the last element of this array using array[array.length – 1]. For example, if you have const str = “a,b,c”, you can get “c” by doing const lastElement = str.split(“,”)[str.split(“,”).length – 1].
Typescript split string and get last element
Let us see how to split a string and get the last element using TypeScript.
Let’s understand the Typescript split() method. In TypeScript, as in JavaScript, the split method divides a string into an ordered list of substrates, placing these substrates into an array. Using the split method’s argument, you can specify the character or pattern for splitting the string.
Here’s the basic syntax:
string.split(separator: string | RegExp, limit?: number): string[]
- separator: The character or regular expression to use for splitting the string.
- limit (optional): An integer that specifies the number of splits; items after the split limit will not be included in the array.
Let us consider a string example separated by commas:
const cityNames = "New York,Los Angeles,Chicago,Houston,Phoenix";
We want to split this string and get the last city name, “Phoenix,” in this case, by using the Typescript split method.
Follow the below steps:
Step 1: Split the String
First, we split the string by the comma separator:
const citiesArray = cityNames.split(",");
Step 2: Get the Last Element
After splitting the string, we can access the last element of the array using the array’s length:
const lastCity = citiesArray[citiesArray.length - 1];
Here’s the complete TypeScript code to split the string and get the last element in Typescript.
const cityNames = "New York,Los Angeles,Chicago,Houston,Phoenix";
const citiesArray = cityNames.split(",");
const lastCity = citiesArray[citiesArray.length - 1];
console.log(lastCity);
You can see the output in the screenshot below once you run the code using Visual Studio Code.
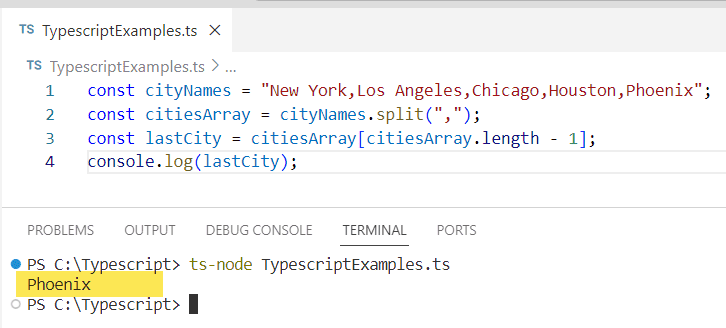
Split the string and get last element in Typescript using Destructuring
TypeScript also supports the destructuring assignment syntax, which can be used to unpack values from arrays. We can use this feature to get the last element of an array in Typescript more concisely.
Here is the complete Typescript code:
const cityNames = "New York,Los Angeles,Chicago,Houston,Phoenix";
const [, ...restCities] = cityNames.split(",").reverse();
const lastCity = restCities[0];
console.log(lastCity);
In this example, we first reverse the array, then use destructuring to ignore all elements except the first one (which is actually the last city name when the array is not reversed).
Here is another example where you can handle special cases, such as empty strings or strings without the separator. Here’s how you can deal with such scenarios:
const cityNames = ""; // An empty string
const citiesArray = cityNames.split(",");
const lastCity = citiesArray.length > 0 ? citiesArray[citiesArray.length - 1] : "";
console.log(lastCity); // Output: ""
In this code, we check if the array’s length is greater than zero before trying to access the last element. If the string is empty or does not contain the separator, lastCity
will be an empty string.
Conclusion
In this Typescript tutorial, I have explained how to split the string and get the last element in Typescript with practical examples.
You may also like:
- TypeScript Split String Into Array
- Typescript string to boolean
- Typescript split string by dot
- Typescript split string multiple separators
- Typescript remove quotes from string
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com