Do you want to split string with multiple separators in Typescript? In this Typescript tutorial, I will explain how to split string with multiple separators in Typescript with various methods.
To split strings in TypeScript with multiple separators, like separating by commas, semicolons, and spaces, you can use regular expressions. For instance, cityString.split(/[,; ]+/) will split a string like “New York, Los Angeles; Chicago Houston” into an array of individual city names. This method is efficient and concise, making it ideal for handling strings with varied separators.
Split string with multiple separators in Typescript
Here, we will see various methods to split strings in TypeScript using multiple separators.
Method 1: Using Regular Expressions
The most versatile method for splitting strings with multiple separators in TypeScript is by using regular expressions. This method allows you to define a pattern that includes multiple separators.
Example:
Let’s say we have a string of city names separated by commas, semicolons, and spaces. We use a regular expression that matches commas, semicolons, and spaces to split this string into individual city names. Here is the complete code.
let cityString: string = "New York, Los Angeles; Chicago Houston";
let separators: RegExp = /[,; ]+/;
let cities: string[] = cityString.split(separators);
console.log(cities);
Output:
[ 'New', 'York', 'Los', 'Angeles', 'Chicago', 'Houston' ]
You can see the output in the screenshot below after I executed the code using Visual Studio code:
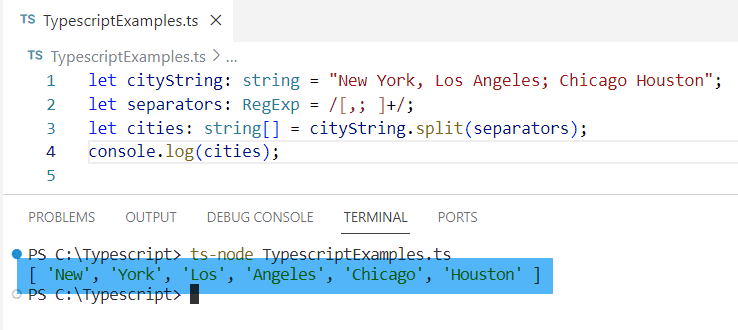
Also, read Typescript Split String
Method 2: Chaining split()
and concat()
If you prefer not using regular expressions, another approach is to chain split()
and concat()
methods. Here is a complete code:
Example:
let cityString: string = "New York, Los Angeles; Chicago Houston";
let cities: string[] = cityString.split(',')
.map(part => part.split(';'))
.reduce((acc, val) => acc.concat(val), [])
.map(part => part.trim().split(' '))
.reduce((acc, val) => acc.concat(val), []);
console.log(cities);
We can split the string multiple times and concatenate the results; you can see the output in the screenshot below:
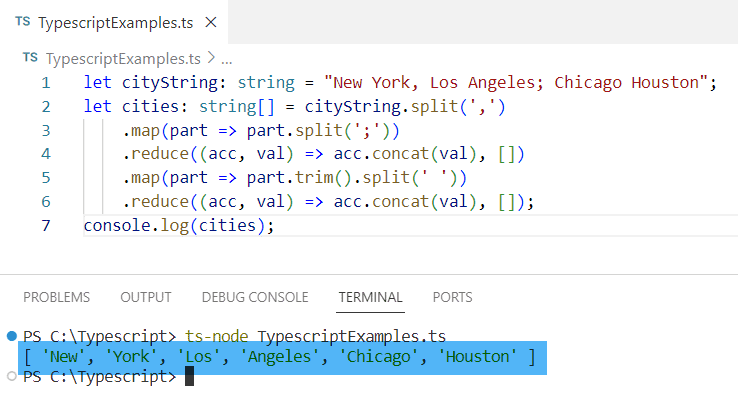
Method 3: Using replace()
and split()
Another method involves replacing all separators with a common separator and then using split()
method in Typescript.
Example:
let cityString: string = "New York, Los Angeles; Chicago Houston";
let normalizedString: string = cityString.replace(/(;| )+/g, ',');
let cities: string[] = normalizedString.split(',');
console.log(cities);
Here, it replace all semicolons and spaces with commas, then splits:
Method 4: Custom Split Function
For more complex scenarios, you might want to create a custom split function to split string multiple separators in Typescript.
Example:
function customSplit(input: string, separators: string[]): string[] {
return input.split(new RegExp(separators.join('|'), 'g'));
}
let cityString: string = "New York, Los Angeles; Chicago Houston";
let cities: string[] = customSplit(cityString, [',', ';', ' ']);
console.log(cities);
Conclusion
In TypeScript, splitting strings with multiple separators can be achieved in various ways, each suitable for different scenarios. Regular expressions offer the most flexibility while chaining split()
and concat()
or using replace()
provides simpler, albeit less powerful, alternatives.
In this Typescript tutorial, I have explained how to split string multiple separators in Typescript using various methods with examples.
You may also like:
- Typescript remove quotes from string
- Typescript replace all occurrences in string
- How to Convert Date to String Format dd/mm/yyyy in Typescript
- Typescript compare strings for sorting
- How to Convert Typescript Dictionary to String?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com