Do you want to convert a dictionary to a string in Typescript? In this Typescript tutorial, I have explained how to convert Typescript dictionary to string using various methods.
To convert a dictionary to a string in TypeScript is using JSON.stringify(). This function converts a JavaScript value to a JSON string. You can write code like const dictionaryAsString: string = JSON.stringify(myDictionary); console.log(dictionaryAsString);
Convert Typescript Dictionary to String
Now, let us check the below methods used to convert a typescript dictionary to a string with complete code and examples.
- Using JSON.stringify() method
- Using Custom Serialization
- Using Third-Party Libraries like Lodash
Using JSON.stringify() method
The easiest method to convert a dictionary to a string in TypeScript is using JSON.stringify(). This function converts a JavaScript value to a JSON string. Here is a complete program and code:
interface Dictionary {
[key: string]: any;
}
const myDictionary: Dictionary = {
name: "John Doe",
age: 30,
occupation: "Software Developer"
};
const dictionaryAsString: string = JSON.stringify(myDictionary);
console.log(dictionaryAsString);
When you run the code using the Visual Studio Code editor, you can see the output below:
{"name":"John Doe","age":30,"occupation":"Software Developer"}
Check out the screenshot below:
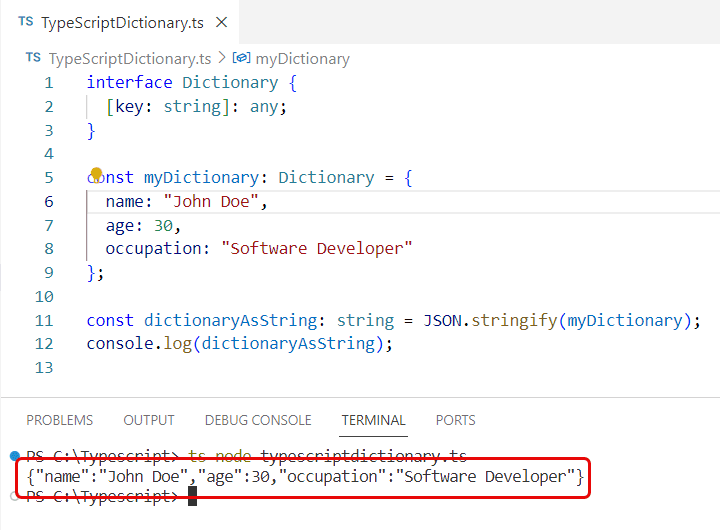
Using Custom Serialization
There may be times when JSON.stringify() is not enough, perhaps when you need to include functions or class instances in your string. In such cases, you can create a custom serialization function. Here is the complete code:
interface Dictionary {
[key: string]: any;
}
function customSerialize(dictionary: Dictionary): string {
let result: string[] = [];
for (const [key, value] of Object.entries(dictionary)) {
if (typeof value === "function") {
result.push(`"${key}": "${value.toString()}"`);
} else {
result.push(`"${key}": ${JSON.stringify(value)}`);
}
}
return `{${result.join(", ")}}`;
}
const myDictionary: Dictionary = {
name: "John Doe",
age: 30,
occupation: "Software Developer",
greet: function() { return `Hello, my name is ${this.name}`; }
};
const dictionaryAsString: string = customSerialize(myDictionary);
console.log(dictionaryAsString);
// Output: '{"name": "John Doe", "age": 30, "occupation": "Software Developer", "greet": "function() { return `Hello, my name is ${this.name}`; }"}'
Once you run the code, you can see the output below screenshot:
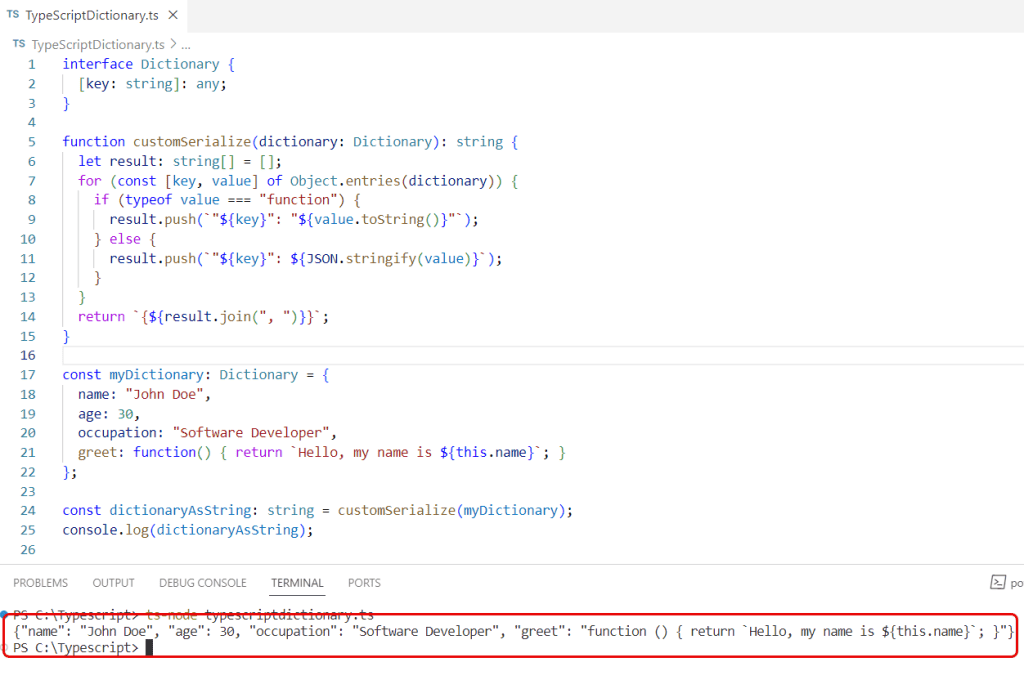
Using Third-Party Libraries like Lodash
You can also use various 3rd party libraries like lodash or immer to convert typescript dictionary to string. Lodash is a utility library that makes JavaScript easier by eliminating the hassle of working with arrays, numbers, objects, strings, etc. Using libraries like lodash can be particularly useful when your TypeScript dictionary contains complex objects or arrays that need custom handling.
Here is a complete code:
import _ from "lodash";
const myDictionary: Dictionary = {
name: "John Doe",
age: 30,
occupation: "Software Developer"
};
const dictionaryAsString: string = JSON.stringify(_.toPlainObject(myDictionary));
console.log(dictionaryAsString);
Conclusion
Converting a TypeScript dictionary to a string can be achieved through several methods, primarily using JSON.stringify() for most cases. You can even use 3rd party libraries like lodash or immer to convert typescript dictionary to string. In this Typescript tutorial, I have explained 3 methods to convert a dictionary to string in Typescript.
You may like the following tutorials:
- How to Loop Through Dictionary in Typescript?
- How to Remove Key from a Dictionary in Typescript?
- How to find key by value in Typescript dictionary?
- How to convert an array to a dictionary in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com