This Typescript tutorial explains how to find key by value in Typescript dictionary using various methods.
To find a key by value in Typescript dictionary, you can use various methods like Using Object.keys() and Array.prototype.find(), Using for in loop, Using Map and Iterators. The easiest way to find a key by value is by using Object.keys() to retrieve an array of keys and then applying the find() method.
Find key by value in Typescript dictionary
In TypeScript, a dictionary is an object that maps keys to values. It’s a collection of key-value pairs where each key is unique. Now, let us check various methods for finding keys by value in Typescript dictionary.
Using Object.keys() and Array.prototype.find()
One of the simplest ways to find a key by value in a TypeScript dictionary is by using Object.keys() to retrieve an array of keys and then applying the find() method.
Here is the complete code:
interface IDictionary {
[key: string]: string;
}
// Sample dictionary with color codes
const colorCodes: IDictionary = {
red: "#FF0000",
green: "#00FF00",
blue: "#0000FF"
};
// Function to find a key by value using Object.keys() and Array.find()
function findKeyByValue(dict: IDictionary, value: string): string | undefined {
return Object.keys(dict).find(key => dict[key] === value);
}
// Example usage:
const valueToFind = "#FF0000";
const key = findKeyByValue(colorCodes, valueToFind);
if (key) {
console.log(`The key for value ${valueToFind} is ${key}.`);
} else {
console.log(`No key found for value ${valueToFind}.`);
}
Once you run the code, you can see the output, like the screenshot below.
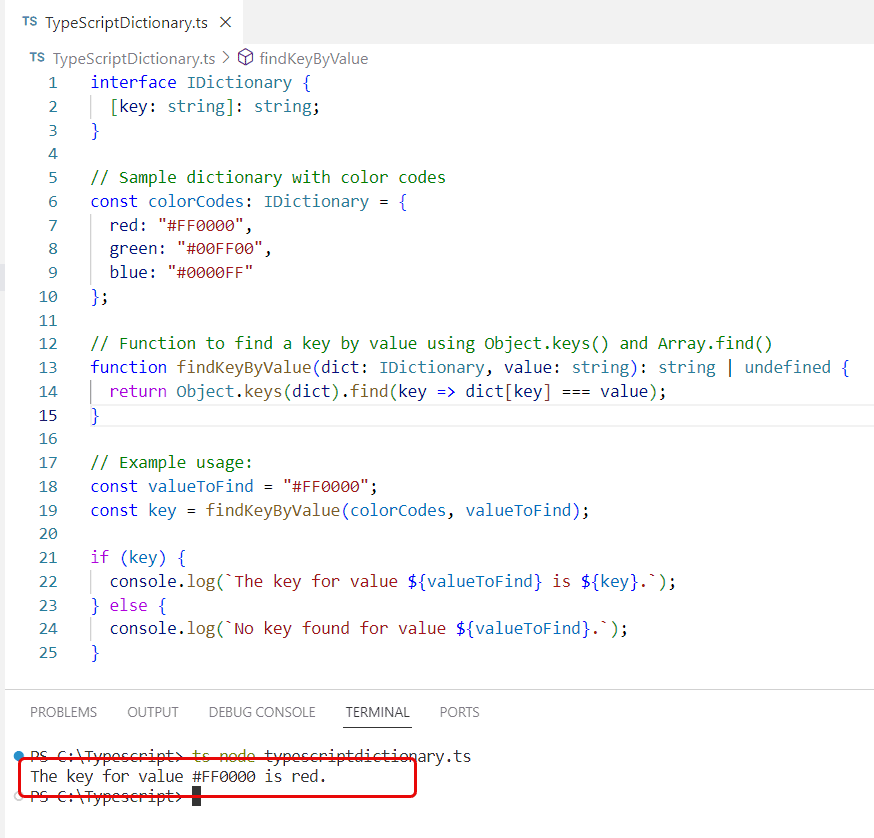
Using for in loop
We can also use the for-in loop in Typescript to find key by value in the Typescript dictionary.
The for…in loop iterates over enumerable properties of an object. The if statement checks if the current value matches the specified value and returns the key if it does.
interface IDictionary {
[key: string]: string;
}
// Sample dictionary with color codes
const colorCodes: IDictionary = {
red: "#FF0000",
green: "#00FF00",
blue: "#0000FF"
};
// Function to find a key by value using a for...in loop
function findKeyByForInLoop(dict: IDictionary, value: string): string | undefined {
for (const key in dict) {
if (dict.hasOwnProperty(key) && dict[key] === value) {
return key;
}
}
return undefined;
}
// Example usage:
const valueToFind = "#00FF00";
const key = findKeyByForInLoop(colorCodes, valueToFind);
if (key) {
console.log(`The key for value ${valueToFind} is ${key}.`);
} else {
console.log(`No key found for value ${valueToFind}.`);
}
You can see the output in the screenshot below:
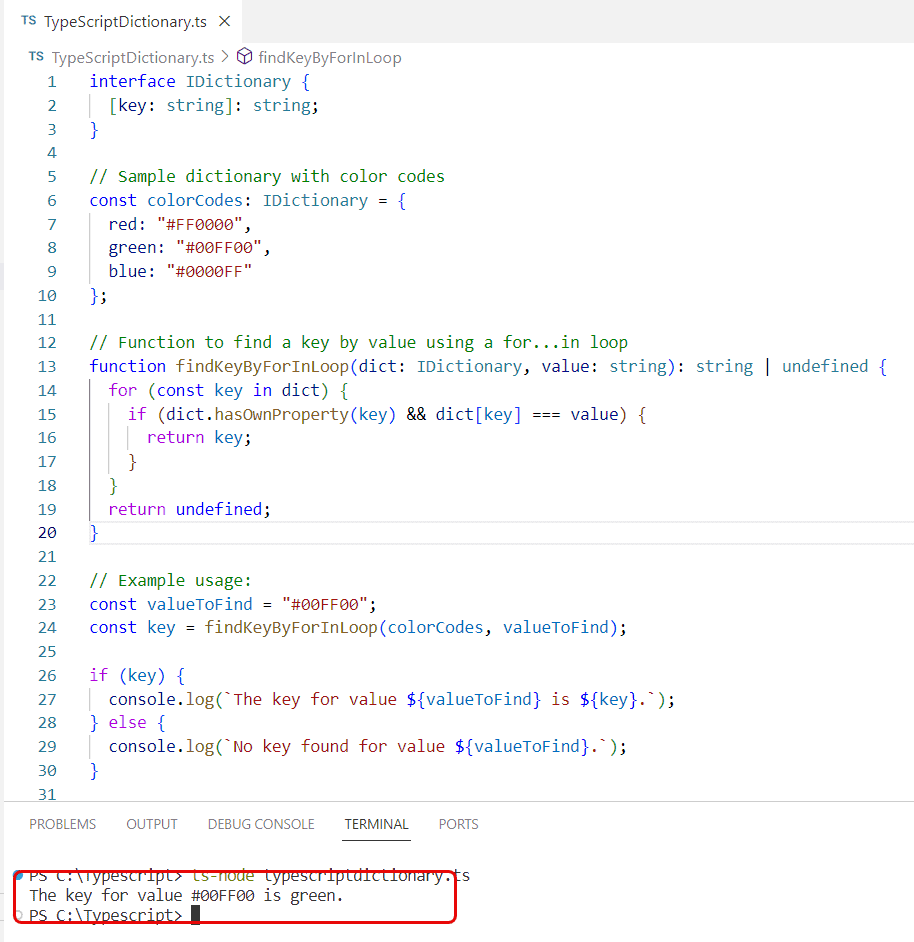
Using Map and Iterators
TypeScript also provides the Map object, which can be particularly efficient for this kind of reverse lookup. We create a Map object that stores key-value pairs. We use the .entries() method, which returns an iterator for iterating through [key, value] pairs.
const colorMap = new Map<string, string>([
["red", "#FF0000"],
["green", "#00FF00"],
["blue", "#0000FF"]
]);
function findKeyByValueInMap(colorMap: Map<string, string>, value: string): string | undefined {
for (const [key, val] of colorMap.entries()) {
if (val === value) {
return key;
}
}
return undefined;
}
const colorName = findKeyByValueInMap(colorMap, "#0000FF");
console.log(colorName);
Conclusion
In this Typescript tutorial, I have learned how to find the key by value in Typescript dictionary using various methods like using Object.keys() and Array.prototype.find(), employing a for…in loop, or utilizing Map and its iterators.
You may like the following tutorials:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com