Do you want to filter a dictionary in Typescript? In the Typescript tutorial, I have explained how to filter a dictionary in Typescript.
Filter a dictionary in Typescript
Filtering a dictionary in TypeScript involves selecting specific key-value pairs that meet certain criteria from an existing dictionary. I will show you 3 different methods to filter a dictionary in Typescript.
- Using Object.keys() and Array.prototype.filter()
- Using Object.entries() and reduce()
- Using Custom Filter Function
1. Using Object.keys() and Array.prototype.filter()
You can filter a dictionary in Typescript using Object.keys() and Array.prototype.filter(). Here is a complete code:
function filterDictionaryByKey(dict: { [key: string]: any }, predicate: (key: string) => boolean): { [key: string]: any } {
let result: { [key: string]: any } = {};
Object.keys(dict).filter(key => predicate(key)).forEach(filteredKey => {
result[filteredKey] = dict[filteredKey];
});
return result;
}
// Example usage:
const myDictionary = {
apple: 1,
banana: 2,
cherry: 3,
date: 4
};
const filteredDictionary = filterDictionaryByKey(myDictionary, key => key.startsWith('a'));
console.log(filteredDictionary);
You can see the output below in the screenshot after running the code using Visual Studio Code.
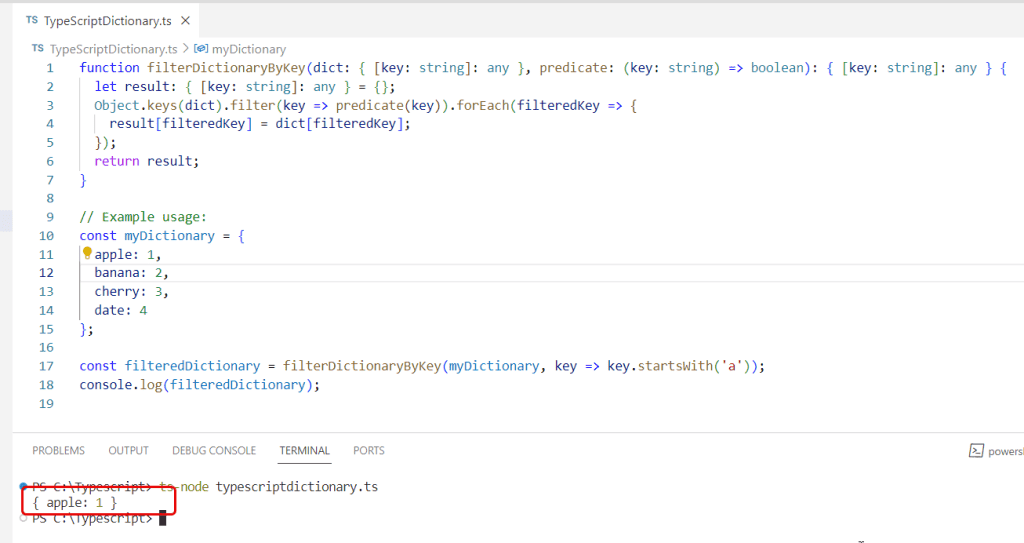
2. Using Object.entries() and reduce()
Another method is using Object.entries() in combination with reduce() to filter the dictionary in Typescript.
function filterDictionaryByValue(dict: { [key: string]: number }, predicate: (value: number) => boolean): { [key: string]: number } {
return Object.entries(dict).reduce((acc, [key, value]) => {
if (predicate(value)) {
acc[key] = value;
}
return acc;
}, {} as { [key: string]: number });
}
// Example usage:
const filteredByValue = filterDictionaryByValue(myDictionary, value => value >= 3);
console.log(filteredByValue);
Once you run the code using Visual Studio Code, you can see the output below:
{ cherry: 3, date: 4 }
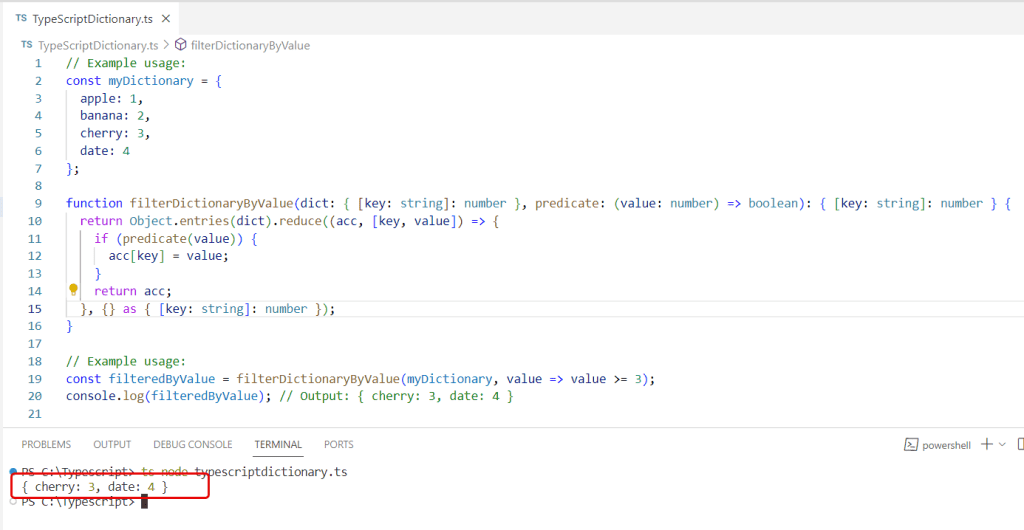
3. Using Custom Filter Function
You can also create a function that iterates over the dictionary entries to filter a dictionary in Typescript.
function customFilter<T>(dict: { [key: string]: T }, filterFn: (key: string, value: T) => boolean): { [key: string]: T } {
let result: { [key: string]: T } = {};
for (let [key, value] of Object.entries(dict)) {
if (filterFn(key, value)) {
result[key] = value;
}
}
return result;
}
// Example usage:
const customFilteredDictionary = customFilter(myDictionary, (key, value) => value % 2 === 0);
console.log(customFilteredDictionary);
Conclusion
In this Typescript tutorial, I have explained how to filter a dictionary in Typescript.
- Using Object.keys() and Array.prototype.filter()
- Using Object.entries() and reduce()
- Using Custom Filter Function
You may like the following tutorials:
- How to filter a dictionary in Typescript?
- How to find key by value in Typescript dictionary?
- How to convert an array to a dictionary in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com