Do you want to get the first element from a dictionary in Typescript? In this Typescript tutorial, I have explained how to get first element from a Typescript dictionary.
To get the first elment from a Typescript dictionary, you can use various methods like by using Object.keys() and Object.values(), iterating with for in loop, and by using Map and Iterators.
Get the First Element from a Typescript Dictionary
Now, let us check how to get the first element from a Typescript dictionary using various methods.
1. Using Object.keys() and Object.values()
To retrieve the first key-value pair from a Typescript dictionary, one can use Object.keys() to grab the keys and Object.values() to fetch the values. Here is a complete example:
function getFirstDictionaryEntry<T>(dictionary: { [key: string]: T }): [string, T] | undefined {
const entries = Object.entries(dictionary);
if (entries.length === 0) {
return undefined;
}
return entries[0] as [string, T];
}
// Usage
const myDict: { [key: string]: string } = {
apple: 'A fruit',
banana: 'A berry',
cherry: 'A drupe'
};
const firstDictEntry = getFirstDictionaryEntry(myDict);
console.log(firstDictEntry);
Here, Object.entries(dictionary) are used to get an array of [key, value] pairs from the dictionary. If the dictionary is not empty, it returns the first entry.
Output:
[ 'apple', 'A fruit' ]
You can check out the screenshot below the output after I ran the code using VS code.
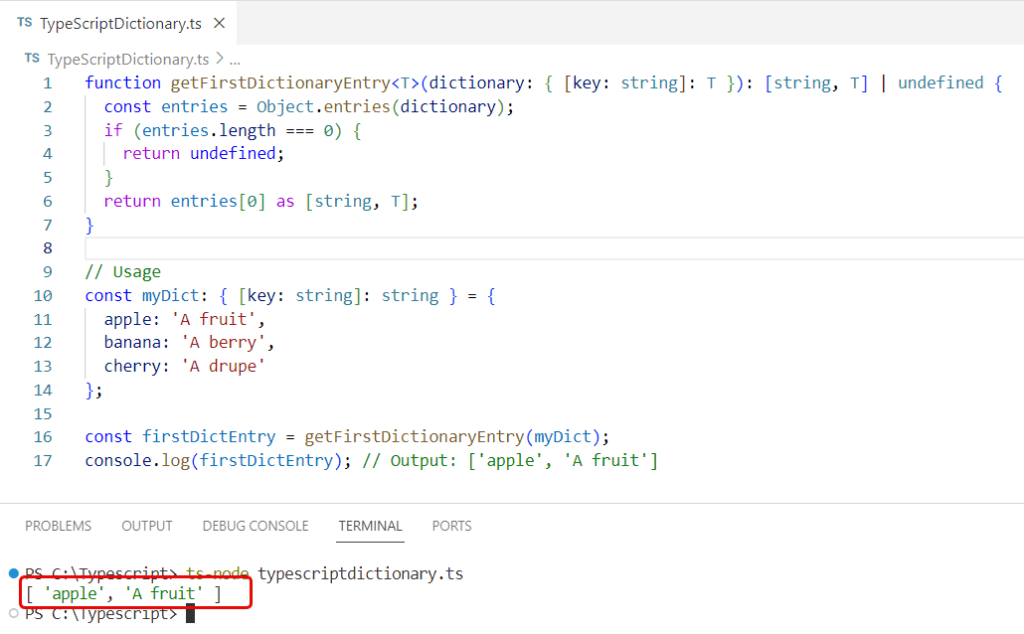
2. Iterate with for…in loop
Another method to get the first element from a TypeScript dictionary is to iterate over dictionary keys in a for-in loop. Here is the complete code.
function getFirstEntryImproved<T>(dictionary: { [key: string]: T }): [string, T] | undefined {
for (const key in dictionary) {
if (Object.prototype.hasOwnProperty.call(dictionary, key)) {
return [key, dictionary[key]];
}
}
return undefined;
}
// Usage
const myDict: { [key: string]: string } = {
apple: 'A fruit',
banana: 'A berry',
cherry: 'A drupe'
};
const firstEntryImproved = getFirstEntryImproved(myDict);
console.log(firstEntryImproved);
Once you run the code, you can
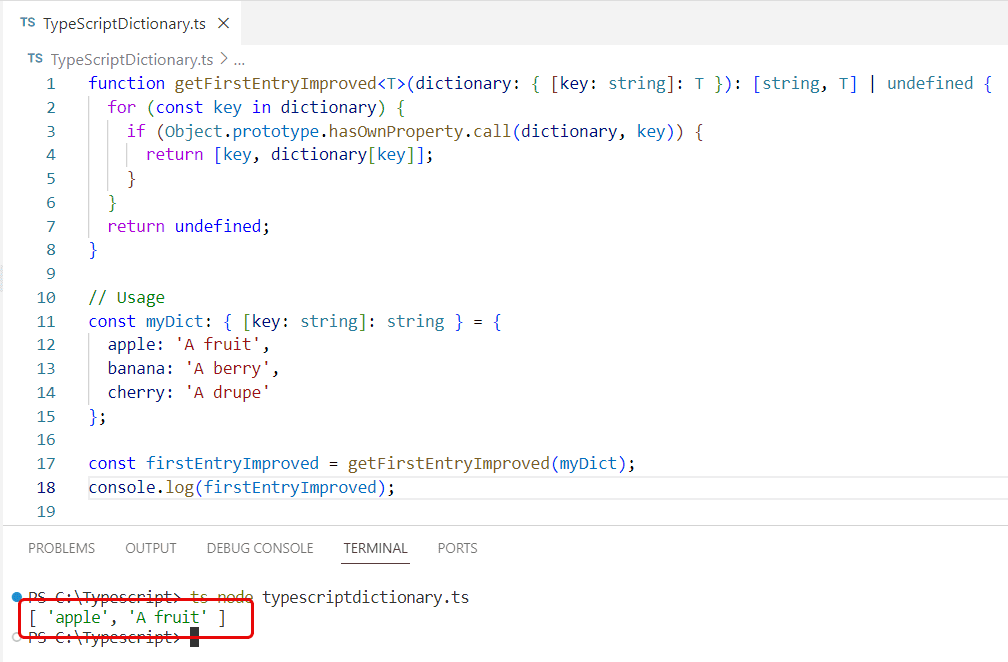
3. Using Map and Iterators
TypeScript’s Map object stores elements in insertion order, which can be utilized to retrieve the first element. Here is a complete code to get the first entry from a Map in TypeScript:
function getFirstMapEntry<K, V>(map: Map<K, V>): [K, V] | undefined {
for (const entry of map.entries()) {
return entry; // Returns the first [key, value] pair
}
return undefined;
}
// Usage
const myMap = new Map<number, string>([
[1, 'Apple'],
[2, 'Banana'],
[3, 'Cherry']
]);
const firstMapEntry = getFirstMapEntry(myMap);
console.log(firstMapEntry);
You can check out the screenshot below:
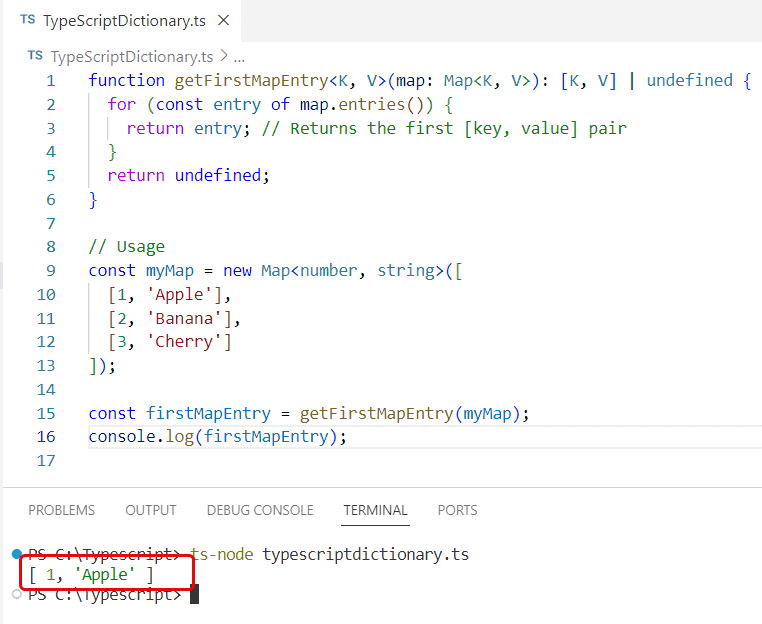
Conclusion
In this Typescript tutorial, I have explained how to get the first element from a TypeScript dictionary using the below methods:
- Using Object.keys() and Object.values()
- Iterate with for…in loop
- Using Map and Iterators
You may also like:
- How to check if a key exists in a dictionary in typescript?
- How to Check if a Dictionary is Empty in TypeScript?
- How to Find Dictionary Length in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com