Do you need to check if a dictionary is empty in Typescript? In this Typescript tutorial, I have explained how to check if a dictionary is empty in Typescript using various methods.
To check if a dictionary is empty in Typescript, you can use various methods like below:
- Using Object.keys()
- Using Object.getOwnPropertyNames()
- Using a for-in loop
- Using JSON.stringify()
Typescript check if a dictionary is empty using Object.keys()
The Object.keys() method is a convenient way to get all the keys of a dictionary as an array. If the array’s length is 0, it means the dictionary does not have any keys, implying that it is empty.
Here is a complete example to check if a dictionary is empty using Object.keys().
function isEmptyDict(dictionary: Record<string, any>): boolean {
return Object.keys(dictionary).length === 0;
}
// Example usage:
const myDict: Record<string, any> = {};
if (isEmptyDict(myDict)) {
console.log('The dictionary is empty.');
} else {
console.log('The dictionary is not empty.');
}
You can see here I got the output “The dictionary is empty.” after executing the code using Visual Studio Code. Check out the screenshot below.
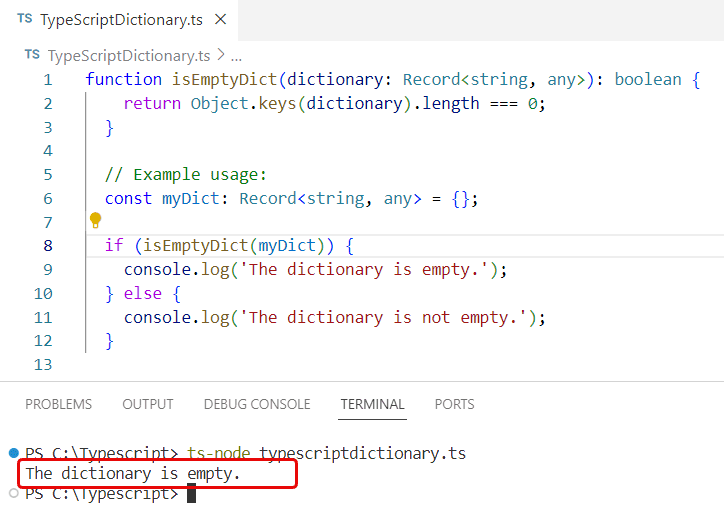
Typescript check empty dictionary using Object.getOwnPropertyNames()
Here is another way to check an empty dictionary in Typescript using the Object.getOwnPropertyNames() method. Similar to Object.keys(), it returns an array of all properties found directly upon a given object.
Here is the complete code:
function isEmptyDict(dictionary: Record<string, any>): boolean {
return Object.getOwnPropertyNames(dictionary).length === 0;
}
// Example usage:
const myDict: Record<string, any> = {};
if (isEmptyDict(myDict)) {
console.log('The dictionary is empty.');
} else {
console.log('The dictionary is not empty.');
}
Here is the output after I ran the code using Visual Studio code in the screenshot below:
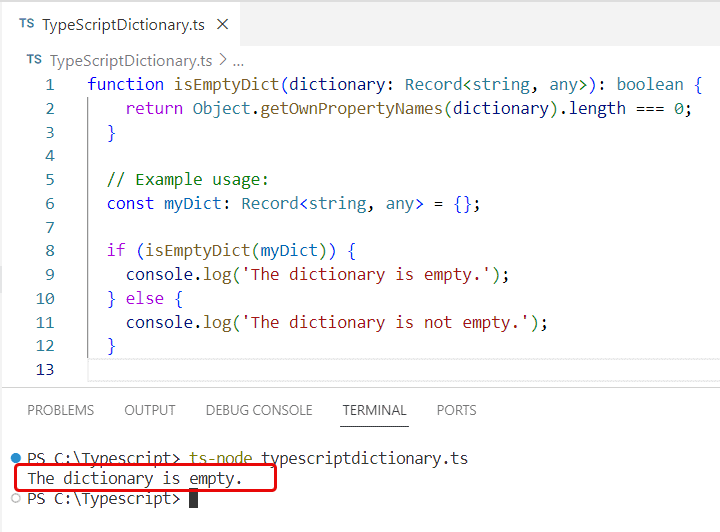
Check if a dictionary is empty in Typescript using a for-in loop
Now, let us check how to use a for-in loop to check if a dictionary is empty in Typescript. Using a for-in loop to iterate over the properties of the dictionary. If the loop executes, it means there is at least one property.
Here is the complete code:
function isEmptyDict(dictionary: Record<string, any>): boolean {
for (const key in dictionary) {
if (dictionary.hasOwnProperty(key)) {
return false;
}
}
return true;
}
// Example usage:
const myDict: Record<string, any> = {};
if (isEmptyDict(myDict)) {
console.log('The dictionary is empty.');
} else {
console.log('The dictionary is not empty.');
}
Here is the screenshot below after running the code using the VS code editor.
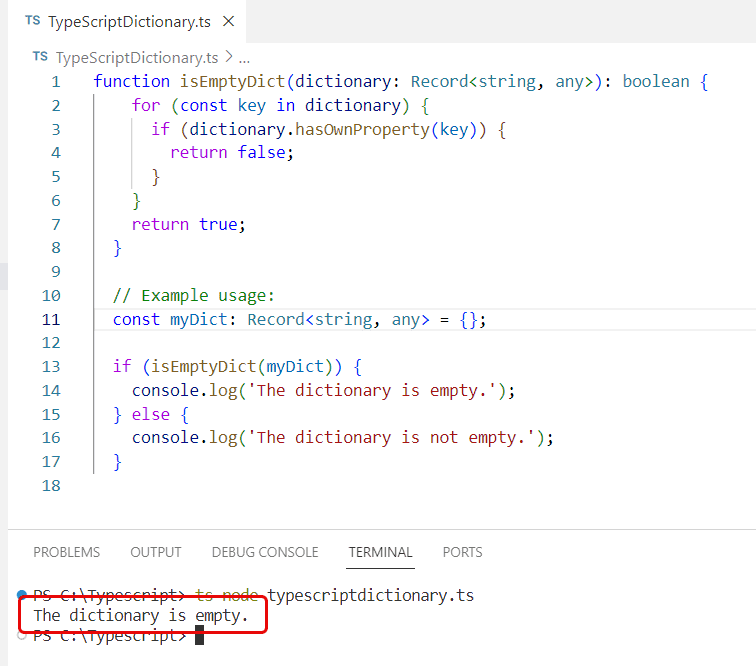
Check if a dictionary is empty in Typescript using JSON.stringify()
Here is the final method to check if a dictionary is empty in Typescript using JSON.stringify(). Although not the most efficient for large objects, using JSON.stringify() can be a quick way to check for an empty object, as it converts the dictionary to its JSON string representation.
Here is the complete code:
function isEmptyDict(dictionary: Record<string, any>): boolean {
return JSON.stringify(dictionary) === '{}';
}
// Example usage:
const myDict: Record<string, any> = {};
if (isEmptyDict(myDict)) {
console.log('The dictionary is empty.');
} else {
console.log('The dictionary is not empty.');
}
Conclusion
In this tutorial, I have explained different methods to check if a dictionary is empty in TypeScript. Each method has its own use case and performance considerations, whether you opt for Object.keys(), Object.getOwnPropertyNames(), the traditional for-in loop, or JSON.stringify(); your choice should depend on your specific context and performance needs in Typescript.
You may like the following tutorials:
- How to check if a key exists in a dictionary in typescript?
- How to Find Dictionary Length in TypeScript?
- How to Remove Key from a Dictionary in Typescript?
- How to Declare a Dictionary in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com