Do you want to know about Typescript string compare? In this Typescript tutorial, I have explained everything on “Typescript compare strings” using various methods.
To compare strings in Typescript, you can use various methods like the equality operators, the localeCompare() Method, and string methods like toUpperCase() and toLowerCase(), etc. The easiest way to compare strings in TypeScript is by using the == or === operators. The == operator tests for abstract equality after performing type coercion if necessary, while === tests for strict equality without type coercion.
Typescript Compare Strings
There are various methods available to compare strings in Typescript. Let us check one by one with a complete example.
Using the Equality Operators
The most straightforward way to compare strings in TypeScript is by using the == or === operators.
Strict Equality: ===
The strict equality operator (===) is the most reliable way to compare two strings in TypeScript, as it checks for both value and type equality.
Here is a simple example.
let firstName: string = "John";
let lastName: string = "Doe";
let anotherJohn: string = "John";
console.log(firstName === anotherJohn);
console.log(firstName === lastName);
In the example above, the first comparison between firstName and anotherJohn returns true because they are both of type string and have the same value. The comparison between firstName and lastName, however, returns false because their values differ.
You can see the output below in the screenshot after I ran the code using Visual Studio Code:
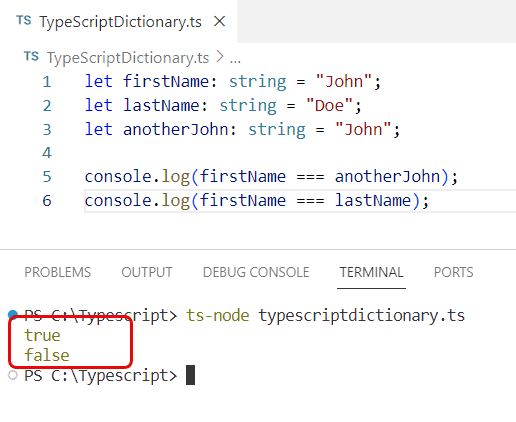
Loose Equality: ==
While TypeScript also supports the loose equality operator (==), it is less strict because it allows type conversion before making the comparison, which can lead to ambiguous results. Therefore, it is recommended to use strict equality for more predictable and accurate comparisons.
Here is a simple example:
console.log('100' == 100); // Outputs: true
Note: it’s not recommended to use loose equality in TypeScript.
Using the localeCompare() Method
Another method to compare strings in TypeScript is using localeCompare(). This method returns a number indicating whether the reference string comes before, after, or is the same as the given string in sort order.
The localeCompare() method is powerful for sorting arrays of strings because it correctly handles Unicode characters and can be customized for different locales.
Here is a complete example:
let city1 = "Atlanta";
let city2 = "Boston";
// A negative number indicates that city1 comes before city2
console.log(city1.localeCompare(city2)); // Output: -1
// A positive number indicates that city2 comes after city1
console.log(city2.localeCompare(city1)); // Output: 1
// Zero indicates that the two strings are equivalent
console.log(city1.localeCompare(city1)); // Output: 0
Once you ran the code using VS code, you can see the output like in the below screenshot.
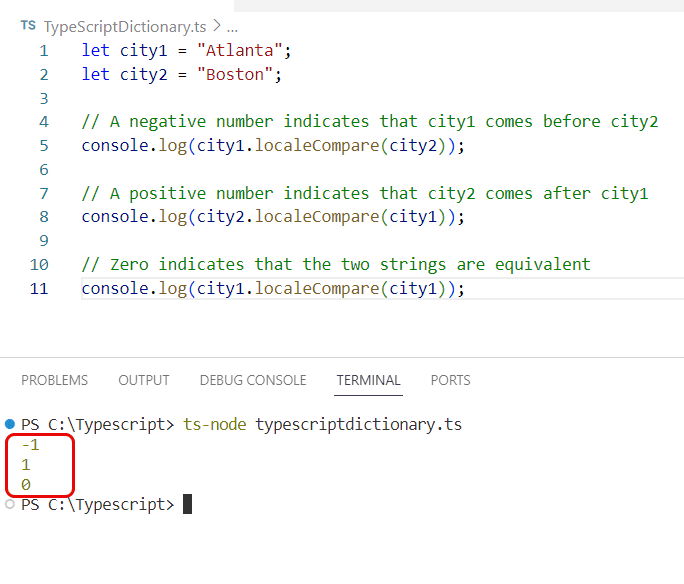
Using String Methods: toUpperCase() and toLowerCase()
To ensure a case-insensitive comparison, you can use the toUpperCase() or toLowerCase() methods before comparing in Typescript.
let city1 = "Seattle";
let city2 = "seattle";
console.log(city1.toLowerCase() === city2.toLowerCase()); // Output: true
console.log(city1.toUpperCase() === city2.toUpperCase()); // Output: true
Sorting Strings in an Array in Typescript
The sort() method can be used to sort strings in Typescript. Here is an example:
let cities = ["Phoenix", "Austin", "New York", "Las Vegas"];
// Sort cities in ascending order
cities.sort((a, b) => a.localeCompare(b));
console.log(cities);
TypeScript Compare Strings for Sorting
If you want to organize data, then sorting string is very common in Typescript. To sort strings effectively, TypeScript provides developers with various methods to handle different sorting criteria, including lexicographical order and locale-specific sorting.
Sorting Strings Alphabetically
To sort strings alphabetically, we typically use the sort() function on an array in Typescript. It sorts the array elements in place and returns the sorted array. By default, it converts the elements to strings and compares their sequences of UTF-16 code units values.
Here is a complete example:
let cities = ["Washington", "New York", "Boston", "San Francisco"];
cities.sort(); // The sort method without a compare function sorts elements as strings by UTF-16 code units order.
console.log(cities);
Sorting becomes more interesting and complex when dealing with case sensitivity and locale-specific rules.
The output will come like the screenshot below.
[ 'Boston', 'New York', 'San Francisco', 'Washington' ]
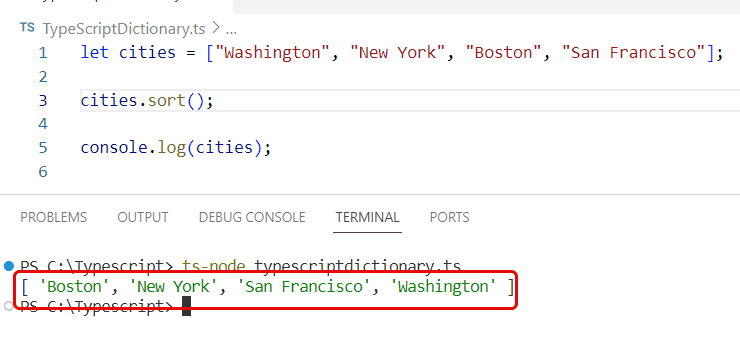
Sorting with localeCompare()
If you want to do sorting with localization in mind, the localeCompare() function is used within the sorting function in Typescript. This allows sorting to be case-insensitive and to respect the user’s locale, which can affect the order of certain characters.
let cities = ["raleigh", "Nashville", "austin", "Denver"];
// Sort cities with localeCompare for case-insensitivity and locale awareness
cities.sort((a, b) => a.localeCompare(b, 'en', { sensitivity: 'base' }));
console.log(cities);
Custom Compare Functions for Sorting
TypeScript also allows you to create custom compare functions to handle complex sorting logic. This can be particularly useful when you need to sort strings based on a specific criterion.
Here is a complete code:
let cities = ["Miami", "Los Angeles", "New York", "Albuquerque"];
// Sort cities by their length
cities.sort((a, b) => {
return a.length - b.length;
});
console.log(cities);
This custom compare function sorts the city names by their length, from the shortest to the longest name.
Conclusion
In TypeScript, comparing strings is very common, and you can do it by using various methods like:
- Using the Equality Operators
- Using the localeCompare() Method
- Using String Methods: toUpperCase() and toLowerCase()
- Sorting Strings in an Array in Typescript
Also, we saw different methods for “TypeScript Compare Strings for Sorting”:
- Sorting Strings Alphabetically
- Sorting with localeCompare()
- Custom Compare Functions for Sorting
You may also like:
- How to Find Dictionary Length in TypeScript?
- How to Convert Typescript Array to String With Separator
- How to get string between 2 characters in Typescript
- How to convert string to double in typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com