In this typescript tutorial, We will see how to convert an array to a string with a separator in typescript using different methods. Here are the methods we are going to cover:
- Using Join ()
- Using For loop
- Using the reduce()
- Using the map() and join ()
Read: How to Get Key by Value from enum String in Typescript
Convert Typescript Array to String With Separator using Join ()
Here we will see how to convert an array to a string with a separator, using join() in typescript.
The join method in typescript is used to join all the items of an array into the string.
Syntax
arr.join(separator)
For example, we have an array of names and by using the join method we will convert the array into string in typescript.
Open the code editor, create a file named as ‘convertArrayToString.ts’, then write the below code:
const names = ['Alex', 'Ron', 'James'];
const separator = ',';
const result = names.join(separator);
console.log(result);
To compile the code and run the below command and you can see the result in the console:
ts-node convertArrayToString.ts
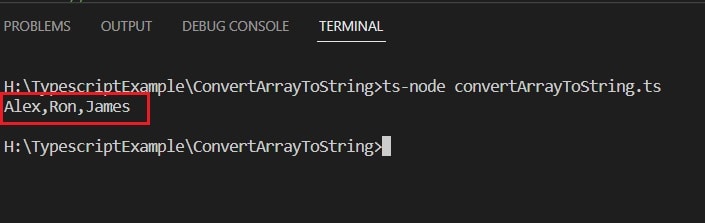
This is an example of how to convert an array to a string in typescript using join().
Convert Typescript Array to String With Separator using For loop()
Here we will see how to convert an array to a string with a separator, using for loop in typescript.
With For loop, a certain section of code can be run a predefined number of times.
For example, we declare an array of names, and then we will use for loop to integrate over the array, concatenate each element with the help of a separator, and create a new string.
In the convertArrayToString.ts file, write the below code:
const names = ['Alex', 'Ron', 'James'];
const separator = ',';
let result = '';
for (let i = 0; i < names.length; i++) {
result += names[i];
if (i !== names.length - 1) {
result += separator;
}
}
console.log(result);
To compile the code and run the below command and you can see the result in the console:
ts-node convertArrayToString.ts
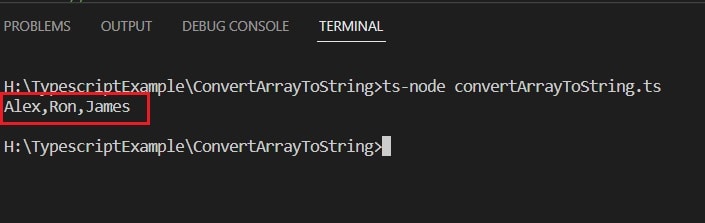
This is how we can convert an array to a string with a separator using for loop in typescript.
Check out: How to push an object into an array in Typescript
Convert Typescript Array to String With Separator using reduce()
Here we will see how to convert an array to a string with a separator, using reduce() in typescript.
To reduce an array to a single value, the reduce() method concurrently applies a function to two items in the array (from left to right).
Syntax:
array.reduce(callback[, initialValue]);
For example, we declare an array of names, then we will use the reduce() to apply a function to each item of an array it will reduce the array to a single value. here the function will concatenate each item of an array with a separator string, creating a new string.
In the convertArrayToString.ts file, write the below code:
const names = ['Alex', 'Ron', 'James'];
const separator = ',';
const result = names.reduce((prev, curr) => prev + separator + curr);
console.log(result);
To compile the code and run the below command and you can see the result in the console:
ts-node convertArrayToString.ts
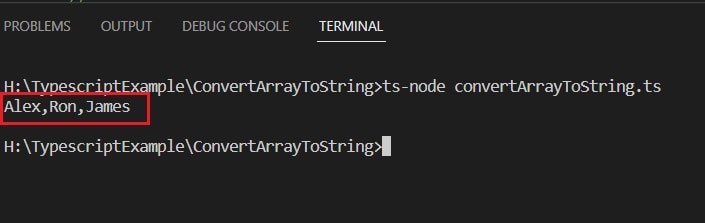
This is an example of converting an array to a string with a separator using reduce() in typescript.
Read: How to get string between 2 characters in Typescript
Convert Typescript Array to String With Separator using the map() and join ()
Here we will see how to convert an array to a string with a separator, using the map() and join() in typescript.
A new array can be created using the TypeScript function arr.map() by calling a specified function on each item of the existing array.
arr.map(callback[, thisObject])
The join method in typescript is used to join all the items of an array into the string.
arr.join(separator)
For example, we declare an array of names, and then we will use map(), which returns the new array with the same item as the primary array. By using the join method on the new array, to convert it to a string with the separator between each item.
In the convertArrayToString.ts file, write the below code:
const names = ['Alex', 'Ron', 'James'];
const separator = ',';
const result = names.map((item) => item).join(separator);
console.log(result);
To compile the code and run the below command and you can see the result in the console:
ts-node convertArrayToString.ts
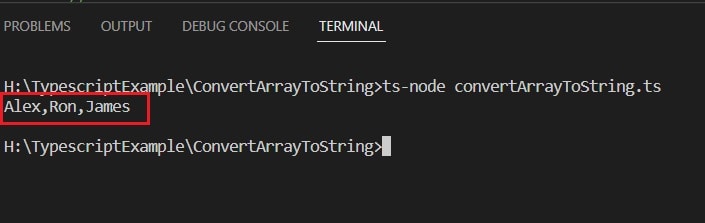
This is an example to convert an array to a string with a separator using the map() and join() in typescript.
Additionally, you may like some more Typescript tutorials:
- How to convert string to double in typescript
- How to get string after character in Typescript
- Typescript string enum reverse mapping
- Sort array of objects in typescript [With 10 examples]
Conclusion
In this typescript tutorial, we saw how to convert an array to a string using different methods. The different methods are
- Using Join ()
- Using For loop
- Using the reduce()
- Using the map() and join ()
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com