Do you want to convert an array to a dictionary in Typescript? In this Typescript tutorial, I have explained how to convert an array to a dictionary in Typescript using various methods.
Convert an array to a dictionary in Typescript
Here, we will discuss a few methods to convert an array to a dictionary in Typescript.
1. Using the Array reduce() Function
The reduce() method is a versatile tool in TypeScript for accumulating values. Here’s how you can use it to convert an array to a dictionary in Typescript.
Here is the complete code:
interface Dictionary<T> {
[key: string]: T;
}
function arrayToDictionary<T>(array: T[], keyField: keyof T): Dictionary<T> {
return array.reduce((obj, item) => {
obj[item[keyField]] = item;
return obj;
}, {} as Dictionary<T>);
}
// Example usage:
interface User {
id: string;
name: string;
}
const users: User[] = [
{ id: 'user1', name: 'John Doe' },
{ id: 'user2', name: 'Jane Doe' }
];
const userDictionary = arrayToDictionary(users, 'id');
console.log(userDictionary);
2. Using the for…of Loop
The Typescript for…of loop offers a straightforward way to create a dictionary from an array. Here is the complete code:
interface Dictionary<T> {
[key: string]: T;
}
function arrayToDictLoop<T>(array: T[], keyField: keyof T): Dictionary<T> {
const dictionary: Dictionary<T> = {};
for (const item of array) {
dictionary[item[keyField] as any] = item;
}
return dictionary;
}
// Example usage:
interface User {
id: string;
name: string;
}
const users: User[] = [
{ id: 'user1', name: 'John Doe' },
{ id: 'user2', name: 'Jane Doe' }
];
const userDictLoop = arrayToDictLoop(users, 'id');
console.log(userDictLoop);
Output:
{
user1: { id: 'user1', name: 'John Doe' },
user2: { id: 'user2', name: 'Jane Doe' }
}
In this snippet, the arrayToDictLoop function is iterating over each element of the array using the for…of loop and adding each item to a new dictionary object based on the specified key field.
You can see the output in the screenshot below.
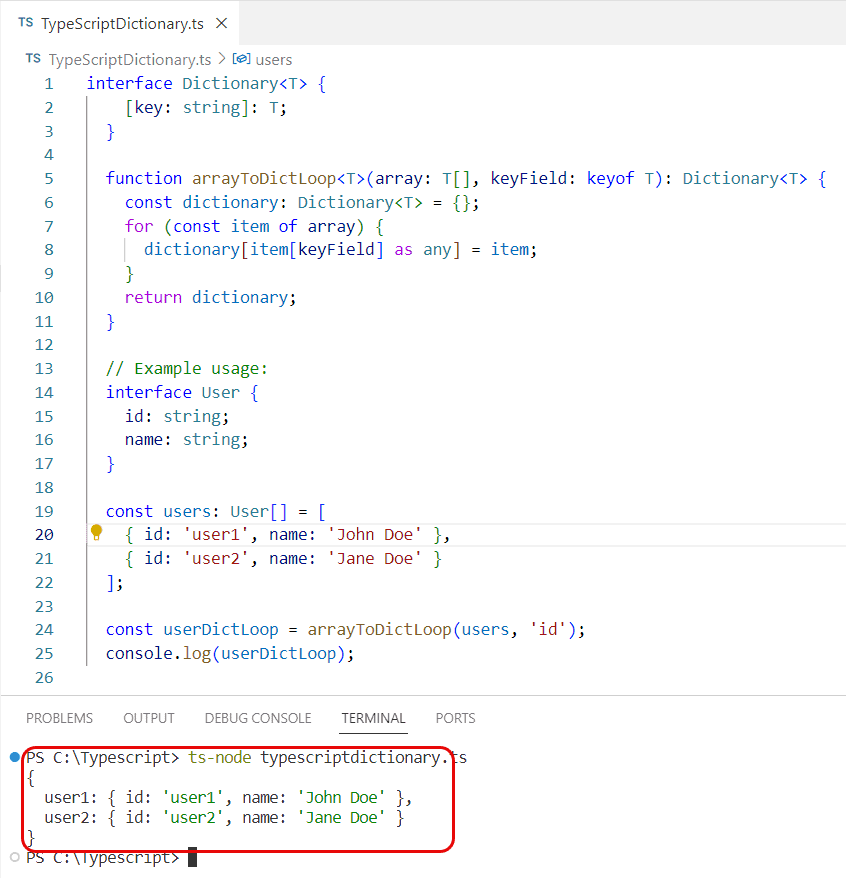
3. Using Array forEach() Method
TypeScript’s forEach() method on arrays is another way to iterate through elements and perform operations:
interface Dictionary<T> {
[key: string]: T;
}
function arrayToDictForEach<T extends Record<K, PropertyKey>, K extends keyof T>(
array: T[],
keyField: K
): Dictionary<T> {
const dictionary: Dictionary<T> = {};
array.forEach((item) => {
dictionary[item[keyField]] = item;
});
return dictionary;
}
// Example usage with a User interface:
interface User {
id: string;
name: string;
}
const users: User[] = [
{ id: 'user1', name: 'John Doe' },
{ id: 'user2', name: 'Jane Doe' }
];
const userDictForEach = arrayToDictForEach(users, 'id');
console.log(userDictForEach);
Conclusion
In this Typescript tutorial, I have explained how to convert an array to a dictionary in Typescript using reduce(), for…of loops, and forEach() methods.
You may also like:
- TypeScript Dictionary vs Map
- How to Get First Element from a Typescript Dictionary?
- How to check if value exists in dictionary in Typescript?
- How to get value from a dictionary in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com