In this Typescript tutorial, I will show you different methods to check if value exists in a TypeScript dictionary.
To check if a value exists in a dictionary in Typescript, you can use various methods. One of the methods is to use the object.values() function, which returns an array of the dictionary’s values. Other methods you can use are looping through entries and by using Array.some() with Object.entries().
Check if a value exists in a dictionary in Typescript
Now, let us check various methods to check if a value exists in a dictionary in Typescript.
1. Using Object.values()
One of the most straightforward ways to check for the existence of a value in a dictionary is by using the Object.values() function, which returns an array of the dictionary’s values. We can then use the includes() method to check if the array contains the value in Typescript.
Here is the complete code:
interface Dictionary<T> {
[key: string]: T;
}
function valueExists<T>(dict: Dictionary<T>, valueToFind: T): boolean {
return Object.values(dict).includes(valueToFind);
}
// Example usage:
const fruitColors: Dictionary<string> = {
apple: 'green',
banana: 'yellow',
cherry: 'red',
};
const hasYellow = valueExists(fruitColors, 'yellow'); // true
console.log(`Does yellow exist in the dictionary? ${hasYellow}`);
You can see this in the below screenshot the output using Visual Studio Code:
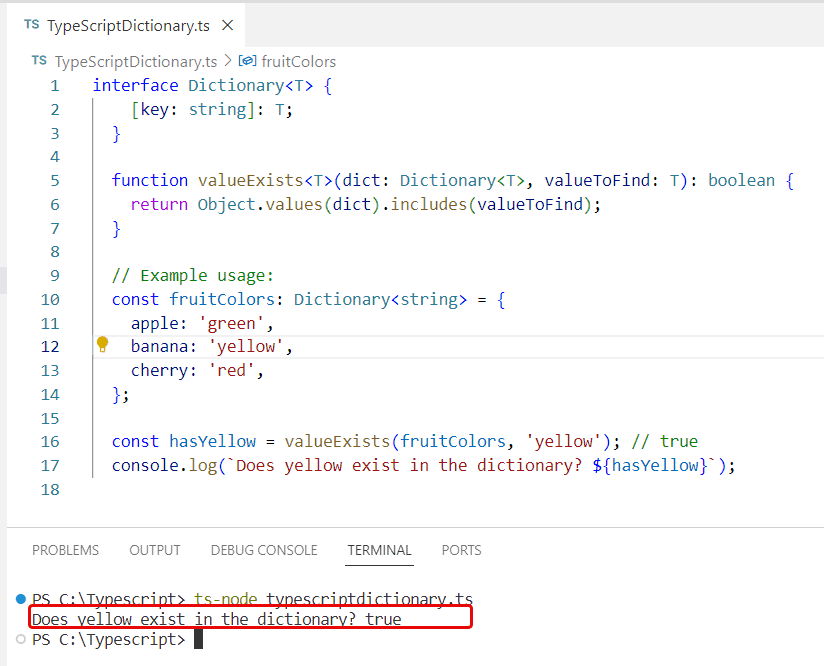
2. Looping Through Entries
If we’re concerned about performance or working with a large dataset, we may want to avoid creating an intermediate array. Instead, we can loop through the dictionary’s entries directly.
Here is a complete code:
interface Dictionary<T> {
[key: string]: T;
}
function valueExistsInDictionary<T>(dict: Dictionary<T>, valueToFind: T): boolean {
for (const key in dict) {
if (Object.prototype.hasOwnProperty.call(dict, key) && dict[key] === valueToFind) {
return true;
}
}
return false;
}
// Example usage:
const fruitColors: Dictionary<string> = {
apple: 'green',
banana: 'yellow',
cherry: 'red',
};
const valueToCheck = 'red';
const exists = valueExistsInDictionary(fruitColors, valueToCheck); // true
console.log(`Does the value "${valueToCheck}" exist in the dictionary? ${exists}`);
// Output: Does the value "red" exist in the dictionary? true
In this example, valueExistsInDictionary is a generic function that takes a dictionary and a value to find within that dictionary. It loops through each key in the dictionary using a for…in loop and checks if the key actually exists on the object itself (as opposed to being inherited from the prototype chain) using Object.prototype.hasOwnProperty.call(). If the current value matches the valueToFind, it returns true. If the loop completes without finding a match, the function returns false.
3. Utilize Array.some() with Object.entries()
A more functional approach involves using Array.some() in combination with Object.entries() to check if a value exists within a dictionary in Typescript.
interface Dictionary<T> {
[key: string]: T;
}
function valueExistsWithSome<T>(dict: Dictionary<T>, valueToFind: T): boolean {
// Check if any value in the dictionary matches valueToFind
return Object.entries(dict).some(([, value]) => value === valueToFind);
}
// Example usage:
const fruitColors: Dictionary<string> = {
apple: 'green',
banana: 'yellow',
cherry: 'red',
};
const valueToCheck = 'red';
const exists = valueExistsWithSome(fruitColors, valueToCheck); // true
console.log(`Does the value "${valueToCheck}" exist in the dictionary? ${exists}`);
// Output: Does the value "red" exist in the dictionary? true
In this TypeScript code snippet, valueExistsWithSome is a generic function that takes a dictionary (dict) and a value (valueToFind). The function uses Object.entries(dict) to get an array of [key, value] pairs from the dictionary. It then applies the Array.some() method to determine if any value in the dictionary is equal to the value we’re looking for. If such a value is found, Array.some() immediately returns true; otherwise, it returns false after checking all entries.
You can see the output in the screenshot below:
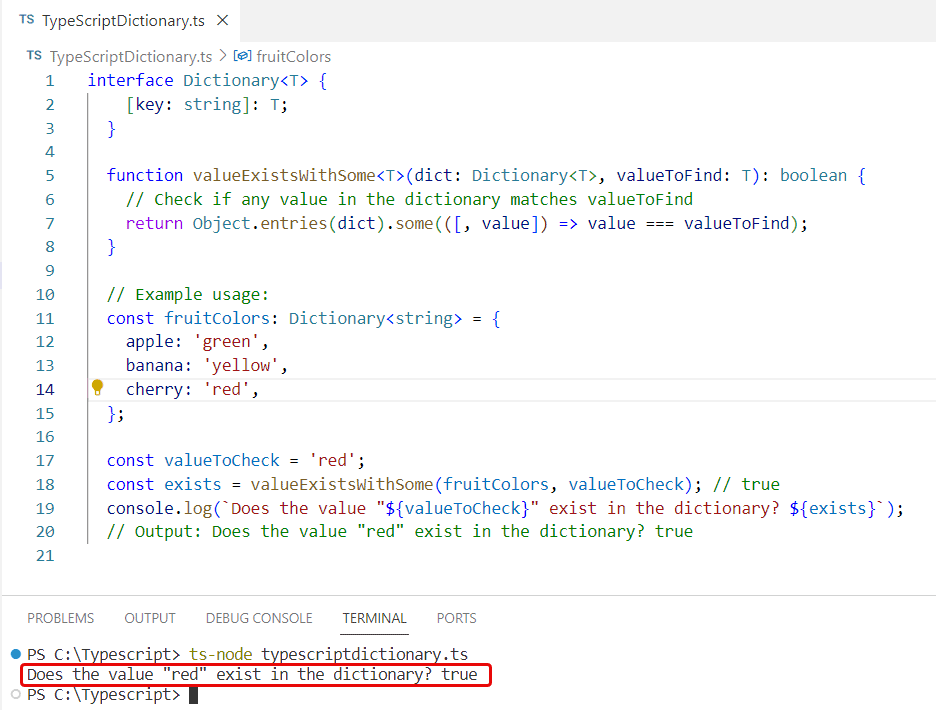
Conclusion
In TypeScript, checking if a value exists in a dictionary can be achieved using various methods. The choice of method can depend on factors like code readability, performance considerations, and the size of the dataset whether you utilize Object.values() with Array.includes(), loop through entries manually, or employ Array.some() with Object.entries(), TypeScript provides you with the tools necessary to effectively manage and query dictionary data structures.
You may like the following tutorials:
- How to get value from a dictionary in Typescript?
- How to initialize empty dictionary in Typescript?
- How to Sort a TypeScript Dictionary by Value?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com