Do you want to get value from a Typescript dictionary? In this Typescript tutorial, I have explained how to get value from a dictionary in Typescript.
To get value from a dictionary in Typescript, you can use the Object.values() method, which returns an array of the dictionary’s values. Like const allValues = Object.values(dictionary); console.log(allValues); There are also other methods you can check out.
Typescript get value from a dictionary
Now, let us check out a few methods to get value from a Typescript dictionary using various methods with complete code and examples.
1. Using the Bracket Notation
One of the simplest ways to retrieve a value from a dictionary is using bracket notation in Typescript. This method allows you to access the value associated with a specific key.
Here is the complete code:
interface IDictionary {
[key: string]: any;
}
// Create a dictionary
const dictionary: IDictionary = {
"apple": "fruit",
"carrot": "vegetable",
"bluebird": "animal"
};
// Access the value using a key
const appleValue = dictionary["apple"];
console.log(appleValue);
Here, you can see the output. I ran the code using the Visual Studio Code editor.
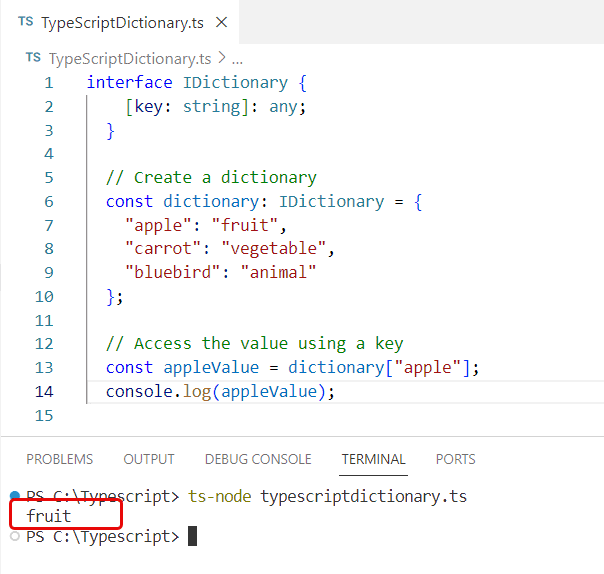
2. Using the get Method
For dictionaries created using the Map object, TypeScript provides the get method. It is a more sophisticated approach compared to the bracket notation and is especially useful when dealing with complex data structures.
Here is the complete code to get value from a dictionary in Typescript using the get() method.
// Create a Map
const dictionary = new Map<string, string>();
dictionary.set("apple", "fruit");
dictionary.set("carrot", "vegetable");
dictionary.set("bluebird", "animal");
// Retrieve the value using get method
const carrotValue = dictionary.get("carrot");
console.log(carrotValue);
Here is the output in the screenshot below after executing using VS code.
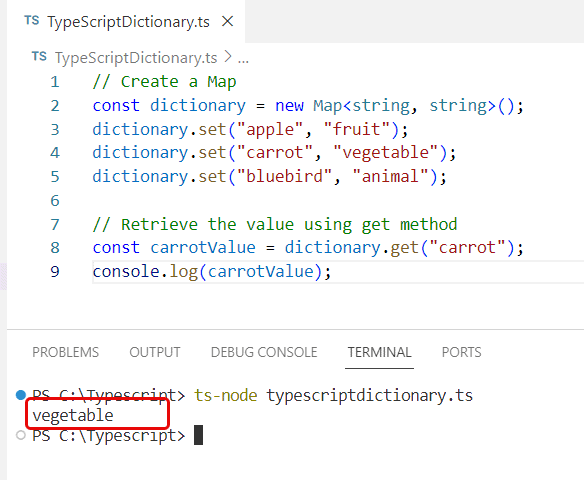
3. Using Object Destructuring
Object destructuring is a modern JavaScript feature that TypeScript also supports. It allows you to unpack values from arrays or properties from objects into distinct variables in Typescript.
const dictionary = {
"apple": "fruit",
"carrot": "vegetable",
"bluebird": "animal"
};
// Destructure the dictionary
const { apple, carrot } = dictionary;
console.log(apple);
console.log(carrot);
You can see the screenshot below about the output.
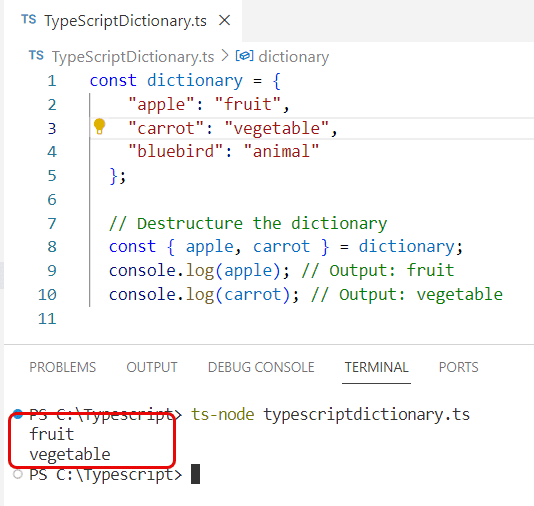
4. Iterating with for…in
If you need to retrieve all values from a dictionary in Typescript, the for…in loop is a handy method. It iterates over the dictionary’s keys, allowing you to access each value.
const dictionary = {
"apple": "fruit",
"carrot": "vegetable",
"bluebird": "animal"
};
for (const key in dictionary) {
if (dictionary.hasOwnProperty(key)) {
const value = dictionary[key];
console.log(value);
}
}
5. Using Object.values()
Another approach to retrieve all values from a TypeScript dictionary is to use the Object.values() method, which returns an array of the dictionary’s values. Here is a complete code:
const dictionary = {
"apple": "fruit",
"carrot": "vegetable",
"bluebird": "animal"
};
const allValues = Object.values(dictionary);
console.log(allValues);
Output:
[ 'fruit', 'vegetable', 'animal' ]
You can see the output in the screenshot below.
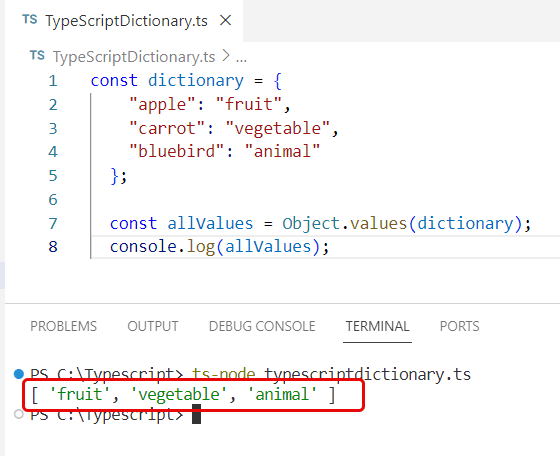
Typescript dictionary get value by key
Now, here is an example of a “typescript dictionary get value by key”.
Here is the complete code to get a value from a dictionary by its key in Typescript.
// Define a dictionary with string keys and values
interface ItemCategoryDictionary {
[key: string]: string;
}
// Initialize the dictionary with some items and their categories
const itemCategory: ItemCategoryDictionary = {
"apple": "fruit",
"carrot": "vegetable",
"almond": "nut"
};
// Function to get the category of an item by its key
function getCategory(item: string): string | undefined {
return itemCategory[item];
}
// Get the category of 'apple'
const appleCategory = getCategory("apple");
console.log(appleCategory);
In this example, getCategory is a simple helper function that takes an item’s name as an argument and returns the category by accessing the dictionary with the provided key. If the key exists in the dictionary, the function returns the corresponding value; otherwise, it returns undefined.
Conclusion
I hope you got an idea of getting values from a dictionary in Typescript. Here, I have explained the below ways to get value from a dictionary in Typescript:
- Using the Bracket Notation
- Using the get Method
- Using Object Destructuring
- Iterating with for…in
- Using Object.values()
You may also like:
- How to check if value exists in dictionary in Typescript?
- How to Convert a Dictionary to an Array in Typescript?
- How to check if a key exists in a dictionary in typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com