Are you looking to know what are the differences between a Typescript dictionary and a map? Check out this complete tutorial I have explained here: “TypeScript Dictionary vs Map“. When we should use a dictionary in Typescript, and when we should use Map.
What is a TypeScript Dictionary?
A TypeScript Dictionary is an object or a record that facilitates the storage of elements in key-value pairs, with string keys and values of any type. It is a collection where you can store data in a format that allows quick lookup of values based on a key.
What is a TypeScript Map?
Conversely, a TypeScript Map is a collection introduced in ES6 (ECMAScript 2015) that holds key-value pairs where both the keys and values can be of any type. Maps remember the original insertion order of the keys, a feature not available in plain objects.
Dictionary vs. Map: The Differences
To understand the fundamental differences between a TypeScript Dictionary and a Map, let’s review them side by side in a table format:
Feature | TypeScript Dictionary | TypeScript Map |
---|---|---|
Key Types | String keys or number keys only. | Keys can be of any type, including objects and functions. |
Iteration | Iterating over a dictionary requires extracting keys or values first. | Maps are directly iterable using the for...of loop. |
Size | No direct property to get the size of the dictionary. | Has a size property to get the number of elements. |
Element Order | Not guaranteed. | Maintains the order of elements as they were added. |
Default Key Existence | Dictionaries can have default keys as they are objects. | Maps do not have default keys. |
Performance | Generally, faster at storing and retrieving string-keyed data. | Better performance in scenarios involving frequent additions and deletions. |
Methods & Properties | Limited to object properties and methods. | Comes with methods like set , delete , has , clear . |
Now that we’ve established the differences, let’s delve into each data structure with illustrative examples.
TypeScript Dictionary Example
In TypeScript, you can use an interface to define the structure of a dictionary. Here’s an example:
interface IDictionary {
[key: string]: any;
}
let userRoles: IDictionary = {
"john.doe": "Admin",
"jane.doe": "User",
"mike.smith": "Moderator"
};
console.log(userRoles["john.doe"]);
In this example, userRoles is a dictionary where the keys are usernames, and the values are roles.
TypeScript Map Example
Creating and using a Map in TypeScript is slightly different. Here is a complete code.
let userRoles = new Map<string, string>();
userRoles.set("john.doe", "Admin");
userRoles.set("jane.doe", "User");
userRoles.set("mike.smith", "Moderator");
console.log(userRoles.get("john.doe"));
With Maps, we use the set method to add items, and get to retrieve them. We can also maintain the order of insertion, and we can use objects as keys if needed.
When to Use Dictionary vs Map
- Use a Dictionary when dealing with a collection of items with string keys, and you need high-performance lookups.
- Opt for a Map when you need keys of any type and want to maintain the order of elements.
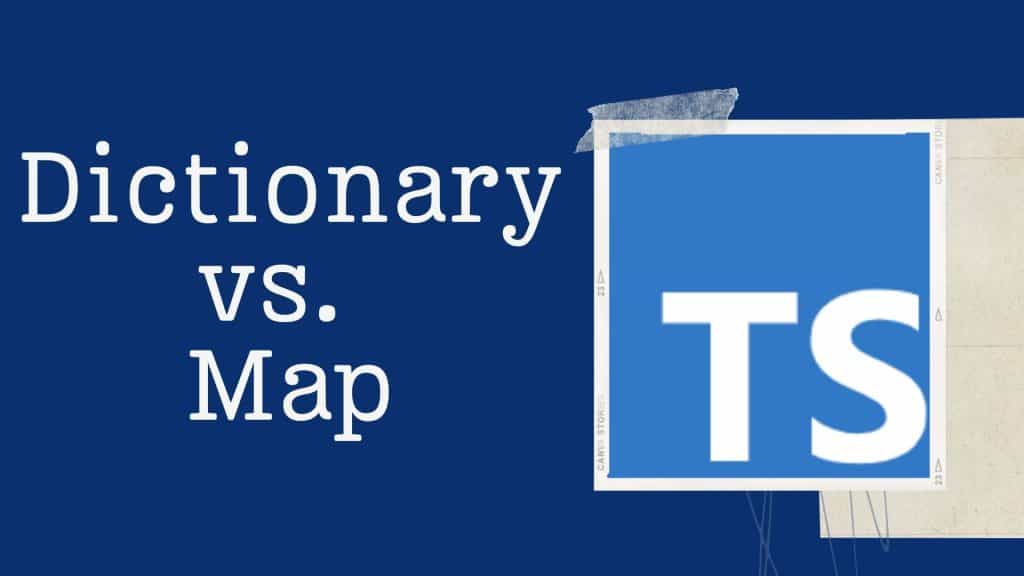
Conclusion
Understanding the differences between a TypeScript Dictionary and a Map is fundamental for developers trying for optimal code performance and clarity. While both structures offer the utility of storing key-value pairs, their differences in key types, iteration capabilities, size property, element order, and methods significantly influence their use cases. In TypeScript, choosing between a Dictionary and a Map hinges on your specific needs regarding key diversity, order preservation, and operation performance.
You may also like:
- Declare a Dictionary in TypeScript
- Loop Through Dictionary in Typescript
- How to Convert Typescript Dictionary to String?
- How to filter a dictionary in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com