Are you looking to remove quotes from string in Typescript? In this Typescript tutorial, I will explain how to escape single quote in Typescript, how to escape double quote in Typescript or remove double quotes from string in Typescript with examples.
To remove quotes from a string in Typescript you can use the replace() method with a regular expression. For instance, str.replace(/[‘”]+/g, ”) will remove both single (‘) and double (“) quotes from the string str. This method is efficient and works well for strings that may contain a mix of single and double quotes.
Remove quotes from string in Typescript
Let us explore different methods to remove quotes from strings in TypeScript using a few real examples.
Method 1: Using String replace() Method
The Typescript replace() method is a straightforward way to remove quotes from a string. It searches a string for a specified value, or a regular expression, and returns a new string where the specified values are replaced.
function removeQuotesUsingReplace(str: string): string {
return str.replace(/['"]+/g, '');
}
// Example
const city = "\"New York\"";
console.log(removeQuotesUsingReplace(city)); // Output: New York
This function will remove both single (‘) and double (“) quotes from the provided string.
After I ran the code using Visual Studio code, you can see the output in the screenshot below:
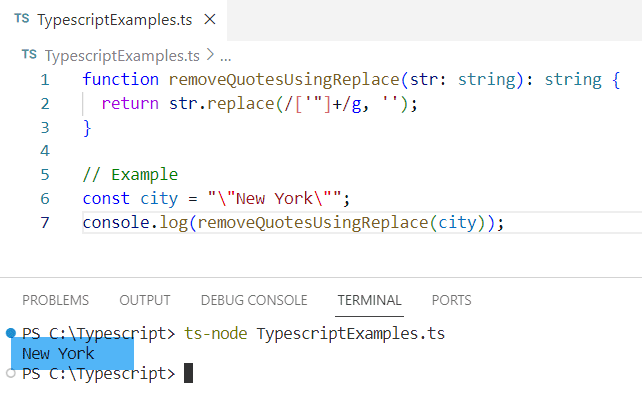
Method 2: Using slice() Method
If the quotes are always at the start and end of the string, you can use the slice() method in Typescript. This method extracts a part of a string and returns the extracted part in a new string.
function removeQuotesUsingSlice(str: string): string {
if (str.startsWith('"') && str.endsWith('"')) {
return str.slice(1, -1);
}
return str;
}
// Example
const city = "\"Chicago\"";
console.log(removeQuotesUsingSlice(city)); // Output: Chicago
This method is particularly useful when you are sure that the string is always quoted.
Once you ran the code, you can see the output like the one in the screenshot below:
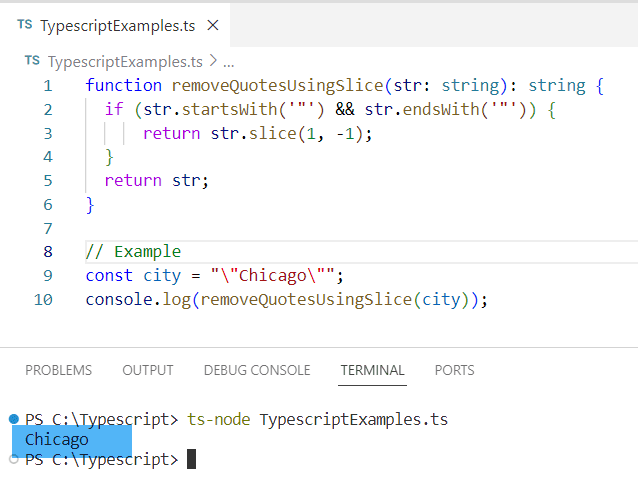
Method 3: Using Regular Expressions
Regular expressions provide a more powerful way to manipulate strings in Typescript. You can use them to target specific patterns, like quotes at the start and end of a string in Typescript. Here is a complete example:
function removeQuotesUsingRegex(str: string): string {
return str.replace(/^"|"$/g, '');
}
// Example
const city = "\"Los Angeles\"";
console.log(removeQuotesUsingRegex(city)); // Output: Los Angeles
This function removes quotes only if they are at the beginning or end of the string.
Method 4: Using trim() with Custom Characters
While the trim() method is typically used to remove whitespace from the beginning and end of a string, you can extend it to remove other characters, including quotes in Typescript. Here is an example:
String.prototype.trimQuotes = function(): string {
return this.replace(/(^['"]|['"]$)/g, '');
};
// Example
const city = "'San Francisco'";
console.log(city.trimQuotes()); // Output: San Francisco
Here, we’ve added a trimQuotes method to the String prototype for ease of use.
Typescript escape double quote
In TypeScript, strings can be enclosed in single quotes ('
), double quotes ("
), or backticks (`
). When your string content includes the same type of quotation marks as those used to enclose the string, those characters need to be escaped to avoid syntax errors. For example, if you have a string enclosed in double quotes and you want to include a double quote character in the string, you will need to escape it.
To escape a double quote in a TypeScript string, you use the backslash (\
) character before the double quote. This tells the TypeScript compiler that the following double quote is a part of the string, not the end of it.
Here is an example of a Typescript escape double quote:
let example = "She said, \"Hello, World!\"";
console.log(example);
// Output: She said, "Hello, World!"
In this example, the backslash in front of the double quotes around Hello, World!
escapes them, so they are included in the output as literal characters. Check out the screenshot below:
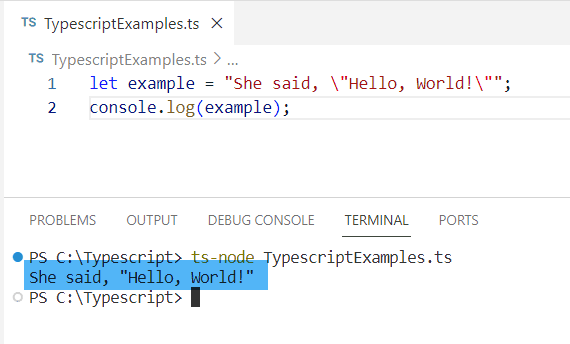
Typescript remove double quotes from string
Now, let us check two methods of removing double quotes from a string in Typescript.
1. Using String replace() Method
The replace() method is the most straightforward way to remove double quotes from a string in Typescript. It can be used to search for a specified substring or a regular expression and replace it with a new substring.
function removeDoubleQuotes(str: string): string {
return str.replace(/"/g, '');
}
// Usage
const stringWithQuotes = 'The city is called "New York"';
console.log(removeDoubleQuotes(stringWithQuotes));
// Output: The city is called New York
In this example, the replace()
method is used with a regular expression that matches all instances of double quotes ("
) and replaces them with an empty string.
Once you run the code, you can see the output below in the screenshot.
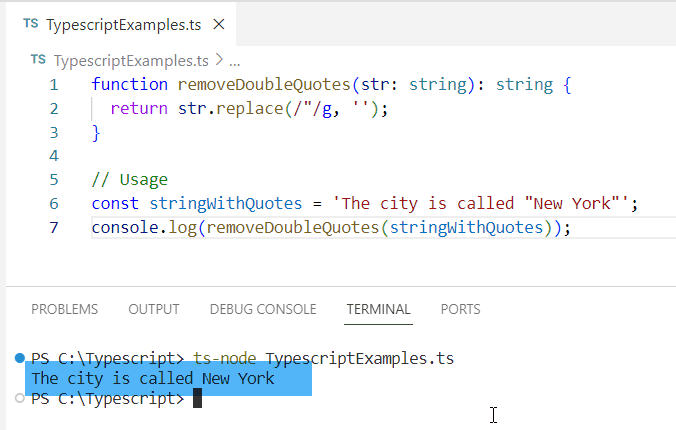
2. Using split()
and join()
Methods
An alternative approach is to use the split()
method to divide the string into an array of substrings, using the double quote character as the separator, and then join()
the array back into a string without the double quotes.
function removeDoubleQuotesSplitJoin(str: string): string {
return str.split('"').join('');
}
// Usage
const exampleString = '"Los Angeles" is sunny.';
console.log(removeDoubleQuotesSplitJoin(exampleString));
// Output: Los Angeles is sunny.
3. Using a Loop
For more complex scenarios, such as when you need to remove double quotes only under certain conditions, a loop can be used in Typescript. Here is a complete example.
function removeDoubleQuotesCustom(str: string): string {
let result = '';
for (let char of str) {
if (char !== '"') {
result += char;
}
}
return result;
}
// Usage
const complexString = 'The "Golden Gate" bridge in "San Francisco"';
console.log(removeDoubleQuotesCustom(complexString));
// Output: The Golden Gate bridge in San Francisco
You can check the output in the screenshot below:
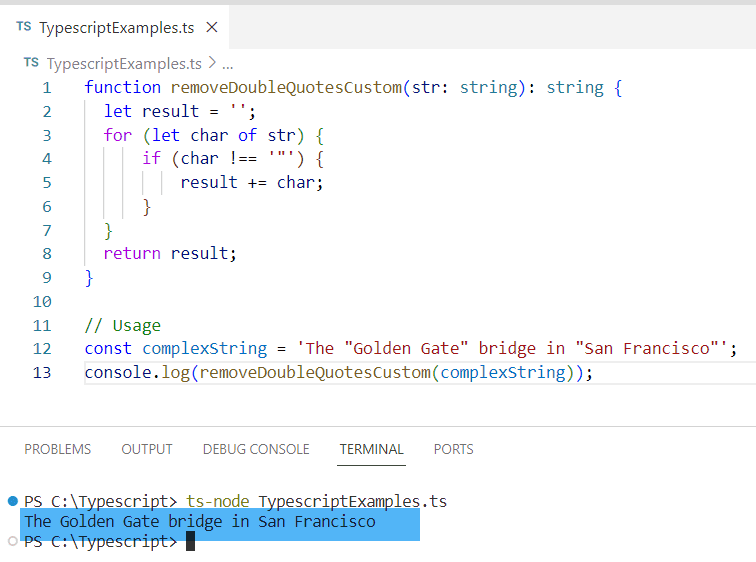
Conclusion
Removing quotes from strings in TypeScript can be achieved through various methods, each suited for different scenarios. Whether you use replace()
, slice()
, regular expressions, or extend the trim()
method, TypeScript provides the flexibility and power to manipulate strings to meet your needs. In this Typescript tutorial, we discussed about:
- Typescript remove quotes from string
- Typescript escape double quote
- Typescript remove double quotes from string
- Typescript escape single quote
You may also like:
- Typescript replace all occurrences in string
- How to split a string with multiple separators in TypeScript?
- Typescript check if a string contains a substring
- How to compare two strings in typescript
- How to check if string is empty in typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com