In this tutorial, I will explain how to check if a string contains a substring in TypeScript. Recently, while working on a project for a client in New York, I needed to validate user input by ensuring that specific keywords were present in a string. This tutorial will help you understand various methods to achieve this efficiently.
To check if a string contains a substring in TypeScript, use the includes()
method. This method returns a boolean value indicating whether the specified substring is present within the string. The syntax is straightforward: string.includes(substring)
, and it is case-sensitive by default. For example, "New York, USA".includes("USA")
would return true
.
Check if a String Contains a Substring in TypeScript
TypeScript, being a superset of JavaScript, provides several ways to determine if a string contains a specific substring. Below, I will cover the most commonly used methods.
1. Using the includes() Method
The includes()
method in TypeScript is the best way to check if a string contains a substring. This method is case-sensitive and returns a boolean value.
Syntax
string.includes(searchString, position);
searchString
: The substring to search for.position
(optional): The position in the string at which to begin searching. Default is 0.
Example
Now, let me show you an example to help you understand this.
const destination: string = "New York, USA";
const keyword: string = "USA";
if (destination.includes(keyword)) {
console.log("The destination includes 'USA'.");
} else {
console.log("The destination does not include 'USA'.");
}
In this example, the console will log “The destination includes ‘USA’.” because the substring “USA” is present in the string “New York, USA”.
I executed the above TypeScript code, and you can see the exact output in the screenshot below:
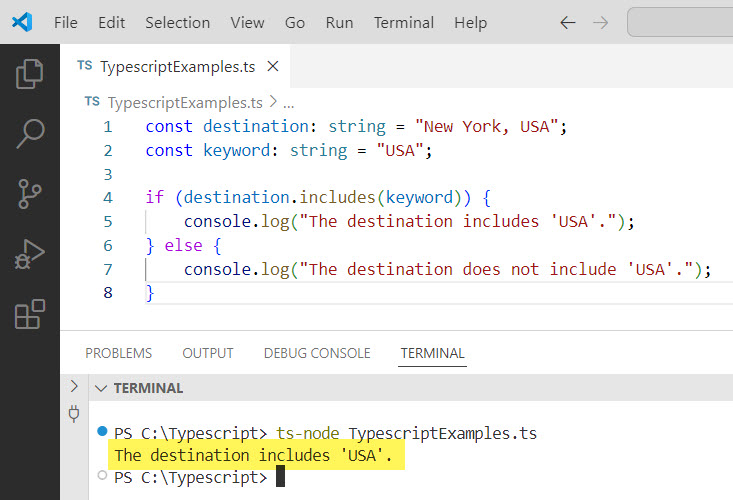
Check out How to Capitalize the First Letter in TypeScript?
2. Using the indexOf() Method
The indexOf()
method returns the index of the first occurrence of a specified substring in TypeScript. If the substring is not found, it returns -1. This method is also case-sensitive.
Syntax
string.indexOf(searchValue, fromIndex);
searchValue
: The substring to search for.fromIndex
(optional): The position in the string at which to begin searching. Default is 0.
Example
Here is an example to check if a string contains a substring in TypeScript.
const city: string = "San Francisco, USA";
const searchString: string = "USA";
if (city.indexOf(searchString) !== -1) {
console.log("The city includes 'USA'.");
} else {
console.log("The city does not include 'USA'.");
}
Here, the console will log “The city includes ‘USA’.” since “USA” is found within the string “San Francisco, USA”.
Here is the exact output in the screenshot below:
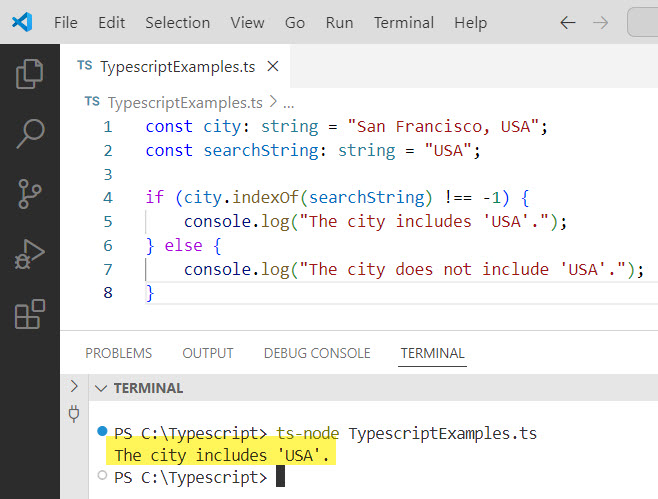
Check out How to Split String By New Line in Typescript?
3. Using the search() Method
The search()
method in TypeScript uses a regular expression to perform the search and returns the index of the first match. If no match is found, it returns -1.
Syntax
string.search(regexp);
regexp
: A regular expression object.
Example
Let me show you an example.
const location: string = "Houston, USA";
const regex: RegExp = /USA/;
const result: number = location.search(regex);
if (result !== -1) {
console.log("The location includes 'USA'.");
} else {
console.log("The location does not include 'USA'.");
}
In this example, the search()
method returns the index of the first occurrence of “USA”. Since “USA” is found in the string “Houston, USA”, the console will log “The location includes ‘USA’.”
Check out How to Split String by Length in Typescript?
4. Using Regular Expressions
Regular expressions provide a powerful way to search for patterns within strings. The test()
method of a regular expression can be used to check for the presence of a substring in TypeScript.
Syntax
regexp.test(string);
regexp
: A regular expression object.string
: The string to search within.
Example
Here is an example.
const address: string = "Chicago, USA";
const pattern: RegExp = /USA/;
if (pattern.test(address)) {
console.log("The address includes 'USA'.");
} else {
console.log("The address does not include 'USA'.");
}
In this example, the console will log “The address includes ‘USA’.” because the regular expression /USA/
matches the substring “USA” in “Chicago, USA”.
Here is the exact output in the screenshot below:
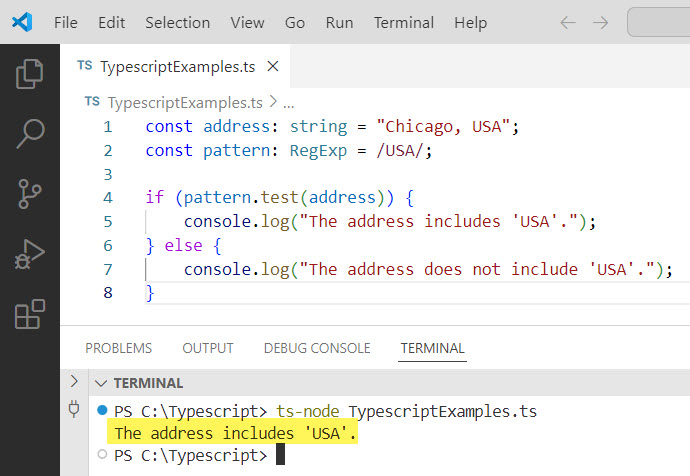
Check out How to Convert String to Number in TypeScript?
5. Case-Insensitive Search
If you need to perform a case-insensitive search, you can use the toLowerCase()
method in conjunction with includes()
, indexOf()
, or regular expressions.
Example
Let me show you an example.
const place: string = "Miami, usa";
const searchTerm: string = "USA";
if (place.toLowerCase().includes(searchTerm.toLowerCase())) {
console.log("The place includes 'USA' (case-insensitive).");
} else {
console.log("The place does not include 'USA' (case-insensitive).");
}
In this case, the console will log “The place includes ‘USA’ (case-insensitive).” because both strings are converted to lowercase before the comparison.
Here is the exact output in the screenshot below:
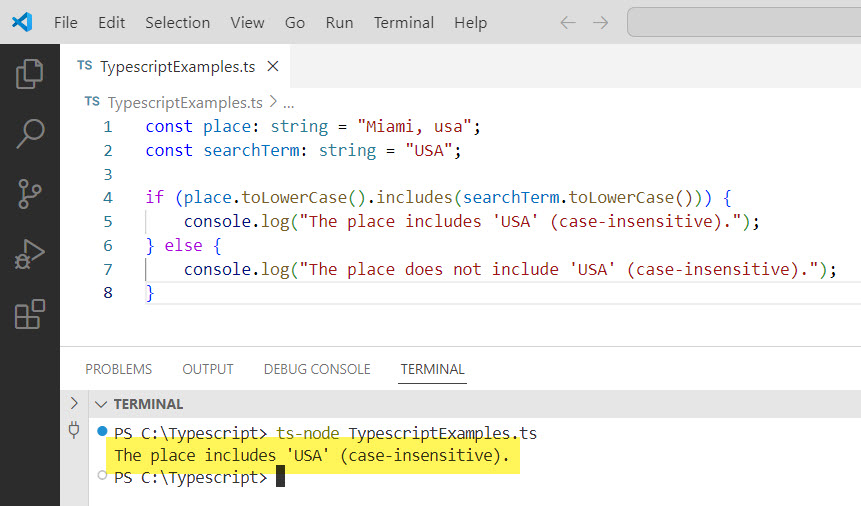
Conclusion
In this tutorial, we have explored various methods to check if a string contains a substring in TypeScript. Whether using the includes()
method, indexOf()
, search()
, or regular expressions, etc.
You may also like the following tutorials:
- How To Split String And Get Last Element In Typescript?
- TypeScript Split String Into Array
- How to Split String by Regex in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com