When working with strings in TypeScript, I was required to split string by a new line in Typescript. In this TypeScript tutorial, I will explain how to do this.
Different operating systems represent new lines with different characters. Windows uses a carriage return followed by a line feed (\r\n
), while Unix-based systems, including Linux and macOS, use just a line feed (\n
).
Example 1: Split a Simple Multiline String in Typescript
Let’s start with a simple example; below is a simple multiline string in Typescript separated by new lines:
const names = `Robert Doe
Jane Smith
Michael Johnson
Emily Davis`;
To split this string into an array of names, we can use the split()
method with a regular expression that matches both \r\n
and \n
.
const nameArray = names.split(/\r?\n/);
console.log(nameArray);
In this example, the regular expression /\r?\n/
matches a carriage return (\r
) followed by a line feed (\n
), or just a line feed (\n
) on its own.
Here is the complete code:
const names = `Robert Doe
Jane Smith
Michael Johnson
Emily Davis`;
const nameArray = names.split(/\r?\n/);
console.log(nameArray);
You can see the output after I executed the code using VS code.
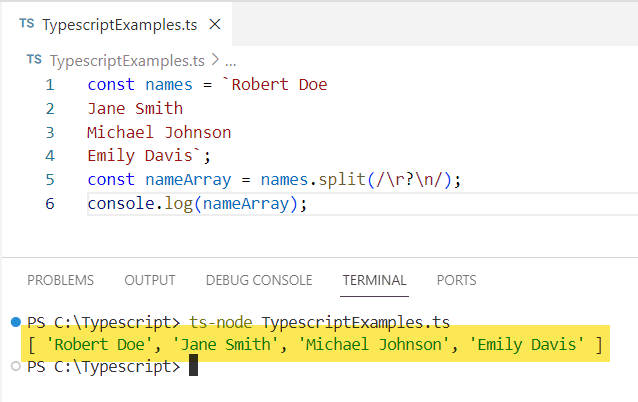
Example 2: Splitting a String with Mixed New Line Characters
Now, let’s consider a more complex example where the input string could contain a mix of Windows and Unix-style new lines:
const mixedNames = `Christopher Walker\r\nLaura Brown\nKevin Miller\r\nRachel Green`;
We can use the same approach as in the first example to split this string:
const mixedNameArray = mixedNames.split(/\r?\n/);
console.log(mixedNameArray);
Even though the mixedNames
string contains both types of new line characters, the regular expression /\r?\n/
ensures that the string is split correctly.
After I executed the code, you can see the output in the screenshot below:
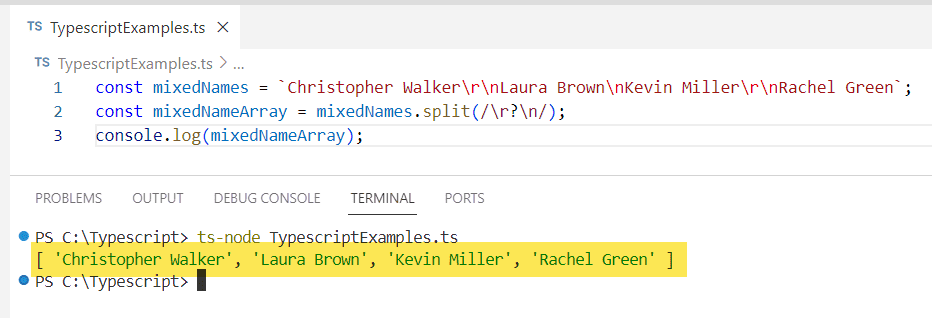
Example 3: Handling Empty Lines
There will be scenarios where you will have empty lines in a string in Typescript. Here is a how to handle empty lines in Typescript.
const namesWithEmptyLines = `Alice Johnson
Bob Lee
Carol King
Derek Hale`;
To split this string and remove any empty lines from the resulting array, you can chain the split()
method with filter()
:
const namesWithoutEmptyLines = namesWithEmptyLines.split(/\r?\n/).filter(name => name.trim() !== '');
console.log(namesWithoutEmptyLines);
Here, the filter()
method is used to remove any strings that are empty after being trimmed of whitespace.
Conclusion
Splitting a string by new line characters in TypeScript can be achieved using the split()
method with a regular expression. I hope, now you got an idea of how to split a string by new line characters in Typescript using various methods.
You may also like:
- How to Split String by Length in Typescript?
- How to Split String by Regex in TypeScript?
- Typescript split string multiple separators
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com