In this tutorial, I will explain how to check if a string is empty in TypeScript. As a developer, I once faced an issue where user input was being processed without proper validation, which led to unexpected behavior in our application. Users from New York and Los Angeles reported that their submitted forms were not being processed correctly. This tutorial will help you avoid these kinds of errors or issues.
To check if a string is empty in TypeScript, you can use the length
property by verifying if str.length === 0
, or use strict equality by comparing the string directly to an empty string with str === ""
. Additionally, to handle strings that contain only whitespace, you can use str.trim().length === 0
. These methods ensure proper validation of user inputs in your applications.
Check for Empty Strings in TypeScript
As a developer, you should check for TypeScript empty strings in scenarios such as form validation, data processing, and ensuring the integrity of user inputs. An empty string can lead to errors or unexpected behavior if not handled correctly.
There are various methods to check if a string is empty in TypeScript. Let me explain each method with examples.
1. Using the Length Property
The simplest way to check if a string is empty in TypeScript is by using the length
property. If the length of the string is zero, then the string is empty.
Here is the TypeScript code.
function isEmpty(str: string): boolean {
return str.length === 0;
}
// Example
const userName = "JohnDoe";
console.log(isEmpty(userName)); // Output: false
const emptyString = "";
console.log(isEmpty(emptyString)); // Output: true
I executed the above code using VS code, and you can see the output in the screenshot below:
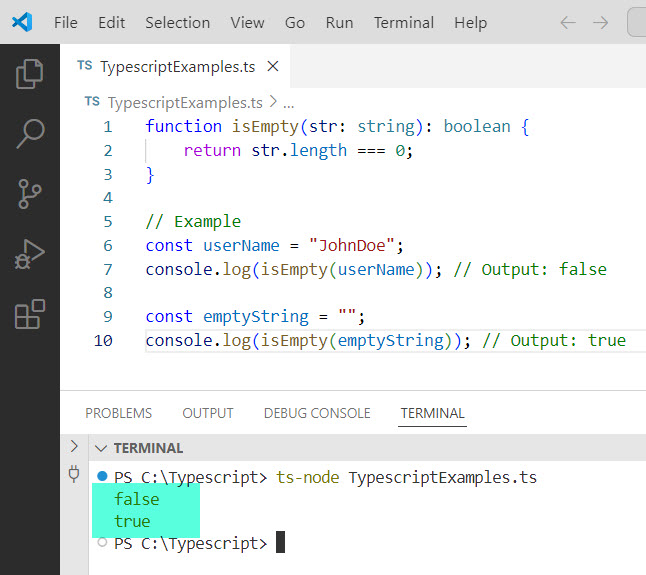
Check out How to Filter Empty Strings from an Array in TypeScript?
2. Using Strict Equality
Another method is to compare the string directly to an empty string using strict equality (===
). Here is the complete code to check if a string is empty using a strict equality operator.
function isEmpty(str: string): boolean {
return str === "";
}
// Example
const city = "San Francisco";
console.log(isEmpty(city)); // Output: false
const noCity = "";
console.log(isEmpty(noCity)); // Output: true
Here is the exact output you can see in the screenshot below:
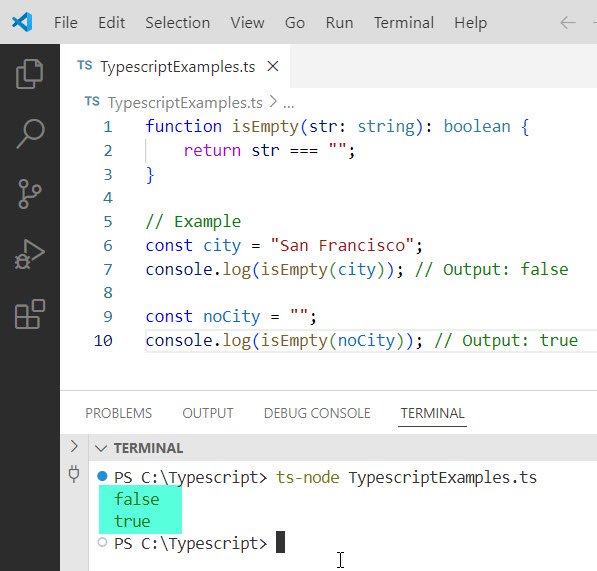
3. Handle Whitespace
Sometimes, you might want to consider strings that contain only whitespace as empty. In this case, you can use the trim()
method before checking the length or using strict equality.
Here is an example to check if the string is empty in TypeScript.
function isEmptyOrWhitespace(str: string): boolean {
return str.trim().length === 0;
}
// Example
const address = " 123 Main St ";
console.log(isEmptyOrWhitespace(address)); // Output: false
const emptyAddress = " ";
console.log(isEmptyOrWhitespace(emptyAddress)); // Output: true
Check out Check if a String Contains a Substring in TypeScript
4. Using Type Guards
TypeScript’s type guards can also be used to ensure that the input is a string before performing the check. This is particularly useful in scenarios where the input might be of various types.
function isString(value: any): value is string {
return typeof value === 'string';
}
function isEmptyString(value: any): boolean {
return isString(value) && value.length === 0;
}
// Example
const state = "California";
console.log(isEmptyString(state)); // Output: false
const noState = "";
console.log(isEmptyString(noState)); // Output: true
const numberValue = 12345;
console.log(isEmptyString(numberValue)); // Output: false
Here is the exact output in the screenshot below:
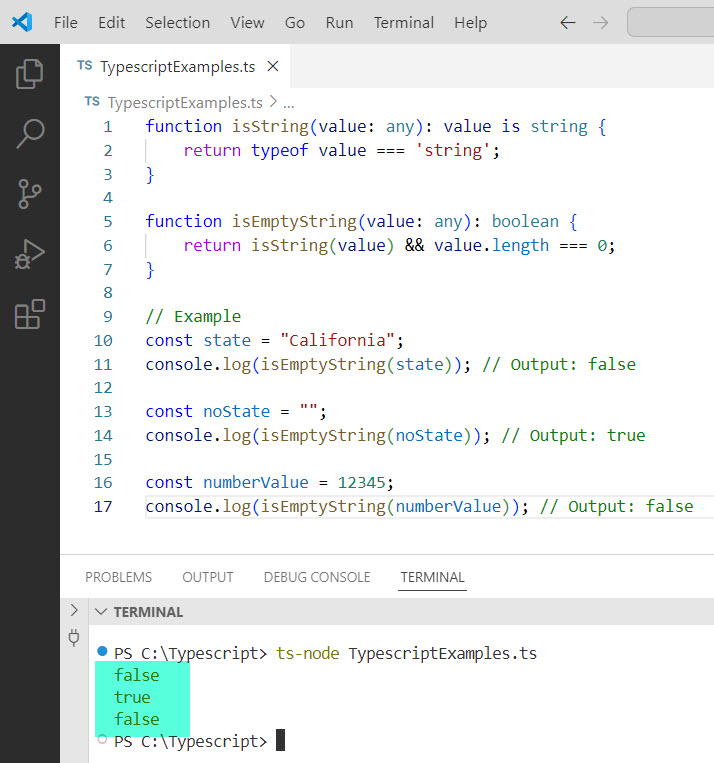
Check out How to Capitalize the First Letter in TypeScript?
5. Combine Checks for Null, Undefined, and Empty
In some cases, you might want to check for null, undefined, or empty strings all at once. This can be done using a combination of conditions. Here is the complete code.
function isNullOrEmpty(str: string | null | undefined): boolean {
return str === null || str === undefined || str.trim().length === 0;
}
// Example
const country = "USA";
console.log(isNullOrEmpty(country)); // Output: false
const noCountry = "";
console.log(isNullOrEmpty(noCountry)); // Output: true
const undefinedCountry = undefined;
console.log(isNullOrEmpty(undefinedCountry)); // Output: true
TypeScript string is null or empty
Let me show you how to check if a string is null or empty in TypeScript.
To check if a TypeScript string is null or empty, you can use the following two methods:
1. Using Strict Equality and Length Check
This method combines checking for null
and undefined
with verifying if the string length is zero.
function isNullOrEmpty(str: string | null | undefined): boolean {
return str === null || str === undefined || str.length === 0;
}
// Example
const name1: string | null = "Alice";
console.log(isNullOrEmpty(name1)); // Output: false
const name2: string | null = "";
console.log(isNullOrEmpty(name2)); // Output: true
const name3: string | null = null;
console.log(isNullOrEmpty(name3)); // Output: true
const name4: string | undefined = undefined;
console.log(isNullOrEmpty(name4)); // Output: true
Here is the exact output you can see in the screenshot below:
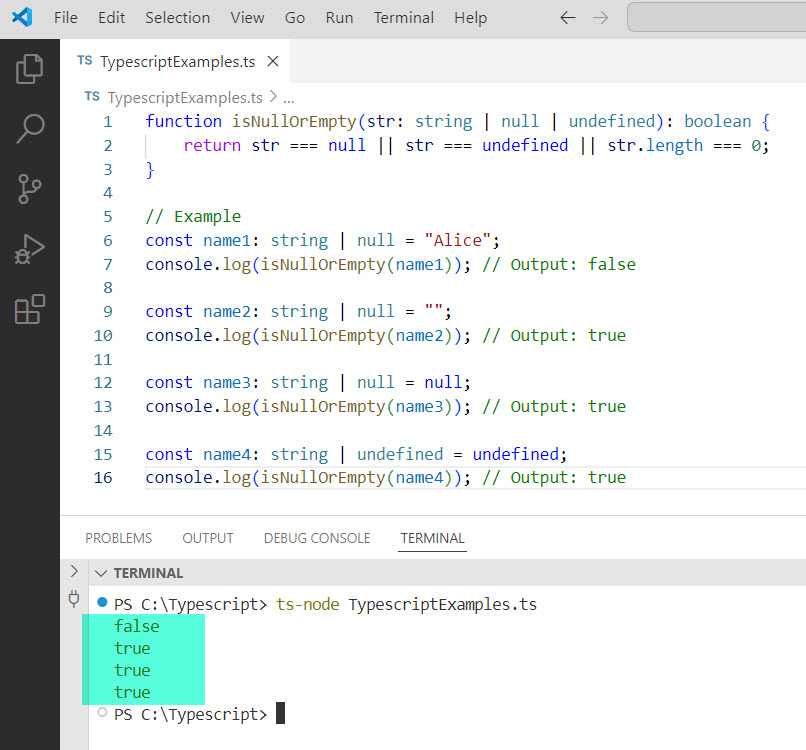
Check out How to Split String by Length in Typescript?
2. Using Trim() Method for Whitespace Handling
This method ensures that strings containing only whitespace are also considered empty by using the trim()
method before checking the length.
function isNullOrWhitespace(str: string | null | undefined): boolean {
return str === null || str === undefined || str.trim().length === 0;
}
// Example
const address1: string | null = "123 Main St";
console.log(isNullOrWhitespace(address1)); // Output: false
const address2: string | null = " ";
console.log(isNullOrWhitespace(address2)); // Output: true
const address3: string | null = null;
console.log(isNullOrWhitespace(address3)); // Output: true
const address4: string | undefined = undefined;
console.log(isNullOrWhitespace(address4)); // Output: true
These methods ensure that your TypeScript application robustly handles strings that are null, undefined, or empty, improving data validation and user input handling.
Conclusion
Validating strings to check if they are empty is important in TypeScript to avoid errors. By using methods such as checking the length
property, using strict equality, handling whitespace, applying type guards, and combining checks for null or undefined, you can ensure that you are checking if a string is empty before. I also explained how to check if a string is null or empty in TypeScript using different methods.
You may also like:
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com