Recently, while working in a Typescript array, I was required to filter empty strings. In this tutorial, I have explained how to filter empty strings from an array in Typescript.
To filter empty strings from an array in TypeScript, you can use the Array.prototype.filter() method, which is straightforward and efficient. For example, given an array const arrayWithEmptyStrings = [“apple”, “”, “banana”, “”, “cherry”];, you can create a new array without empty strings by using const filteredArray = arrayWithEmptyStrings.filter(str => str !== “”);. This method checks each element, and if it is not an empty string, it includes it in the new array, resulting in [“apple”, “banana”, “cherry”].
Filter Empty Strings from an Array in TypeScript
There are different methods you can use to filter empty strings from a Typescript array. Let us check out with complete code and examples.
Method 1: Using Array.prototype.filter()
The filter() method in Typescript creates a new array with all elements that pass the test implemented by the provided function. This is one of the easiest ways to remove empty strings from an array in Typescript.
Here is a complete code:
const arrayWithEmptyStrings = ["New York", "", "Los Angeles", "", "Chicago"];
const filteredArray = arrayWithEmptyStrings.filter(str => str !== "");
console.log(filteredArray);
In this example, the filter()
method checks each element in the Typescript array. If the element is not an empty string, it is included in the new array.
You can see the output in the screenshot below after I executed the Typescript code using VS code. It gives the new array like:
[ 'New York', 'Los Angeles', 'Chicago' ]
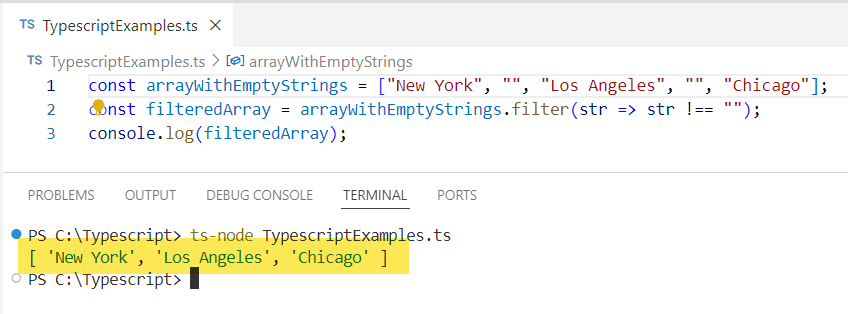
Method 2: Using Array.prototype.reduce()
The reduce()
method applies a function against an accumulator and each element in the array to reduce it to a single value. This can also be used to filter out empty strings from a Typescript array.
Here is an example; you can see the complete code.
const arrayWithEmptyStrings: string[] = ["New York", "", "Los Angeles", "", "Chicago"];
const filteredArray = arrayWithEmptyStrings.reduce<string[]>((acc, str) => {
if (str !== "") {
acc.push(str);
}
return acc;
}, []);
console.log(filteredArray);
Here, reduce()
iterates over each element and pushes non-empty strings to the accumulator array.
You can see the output in the screenshot below after I executed the code using VS code.
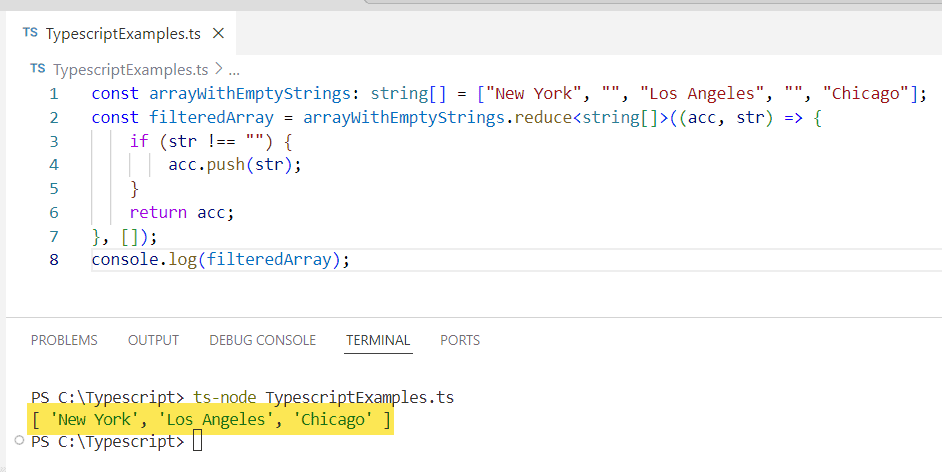
Method 3: Using TypeScript Type Guards
Type guards can be used to filter out empty strings in the Typescript array while ensuring type safety.
Here is an example.
function isNonEmptyString(str: string): str is string {
return str !== "";
}
const arrayWithEmptyStrings = ["New York", "", "Los Angeles", "", "Chicago"];
const filteredArray = arrayWithEmptyStrings.filter(isNonEmptyString);
console.log(filteredArray);
In this example, isNonEmptyString
is a type guard that ensures only non-empty strings are included in the filtered array.
Method 4: Using Regular Expressions
Regular expressions can be very helpful to match and filter strings based on patterns in Typescript.
Here is the complete example.
const arrayWithEmptyStrings = ["New York", "", "Los Angeles", "", "Chicago"];
const filteredArray = arrayWithEmptyStrings.filter(str => /\S/.test(str));
console.log(filteredArray);
The regular expression \S
matches any non-whitespace character, effectively filtering out empty strings.
Conclusion
Filtering empty strings from an array in TypeScript can be done in several ways, like filter()
, reduce()
, the type safety of type guards, or by using regular expressions.
I hope now you can filter empty strings from an array in Typescript.
You may also like the following tutorials:
- Filter Duplicates from an Array in TypeScript
- TypeScript Array Filter with Multiple Conditions
- Remove Null Values from an Array in TypeScript
- TypeScript for Loop with Examples
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com