TypeScript, a popular programming language that builds on JavaScript, offers various methods to handle loops efficiently. One such method is the for loop, widely used for iterating over arrays, lists, or any iterable objects.
Different types of for loops are available in TypeScript, such as the traditional for loop, for…of, and for…in loops. Each has its unique use cases and benefits. By understanding and utilizing these loops, programmers can iterate over data structures easily and precisely.
Using examples, this tutorial will guide you through using these loops in Typescript. Whether you are iterating through an array of numbers or an object’s properties, as a TypeScript developer, you should know how to use these loops.
What are For Loops in TypeScript
For loops in TypeScript, you can run a block of code multiple times based on a set condition.
Basic Syntax of For Loop
The basic syntax of a for loop in TypeScript is similar to JavaScript. It consists of three main parts: initialization, condition, and increment.
for (let i = 0; i < 5; i++) {
console.log(i);
}
- Initialization: This part runs before the loop starts. It usually initializes a variable (e.g.,
let i = 0
). - Condition: The loop continues running as long as this condition is true (e.g.,
i < 5
). - Increment: This part runs after each iteration, increasing or modifying the loop variable (e.g.,
i++
).
This simple example will print numbers 0 through 4 in the console.
You can see the output in the screenshot below after I executed the complete code using the VS code.
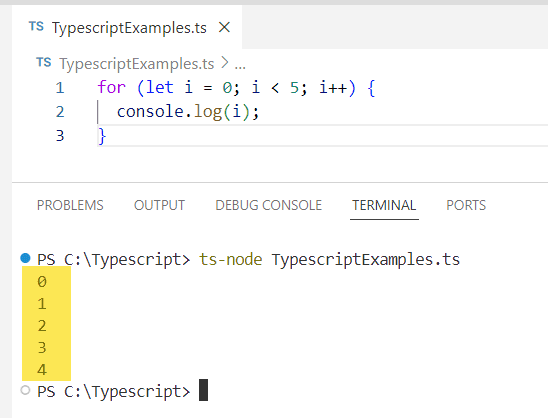
For Loop Variations
TypeScript includes multiple variations of the for loop to handle different situations.
- Traditional For Loop: It is the standard for loop discussed above.
- For..of Loop: Used to iterate over iterable objects like arrays.
let arr = [10, 20, 30];
for (let value of arr) {
console.log(value);
}
This loop prints 10, 20, and 30.
- For..in Loop: Used to iterate over the enumerable properties of an object.
let obj = {a: 1, b: 2, c: 3};
for (let key in obj) {
console.log(key + ": " + obj[key]);
}
This loop prints:
a: 1
b: 2
c: 3
Working with Iterables
When working with iterables in TypeScript, it’s crucial to understand the various types and how to iterate over them effectively. Arrays, strings, tuples, maps, and sets each have specific ways to handle their elements during iteration.
1. Iterating Over Arrays
TypeScript arrays are a common data structure to store a list of values. To iterate over arrays, the for...of
loop is widely used because it directly returns each value of the array, providing a clean and concise syntax.
let numbers: number[] = [1, 2, 3, 4, 5];
for (let num of numbers) {
console.log(num);
}
Another method is the for...in
loop, which iterates over the array’s indices. This can be useful if both the index and value are needed.
let numbers: number[] = [1, 2, 3, 4, 5];
for (let index in numbers) {
console.log(`Index: ${index}, Value: ${numbers[index]}`);
}
You can see the output in the screenshot below; I executed the entire code using VS code.
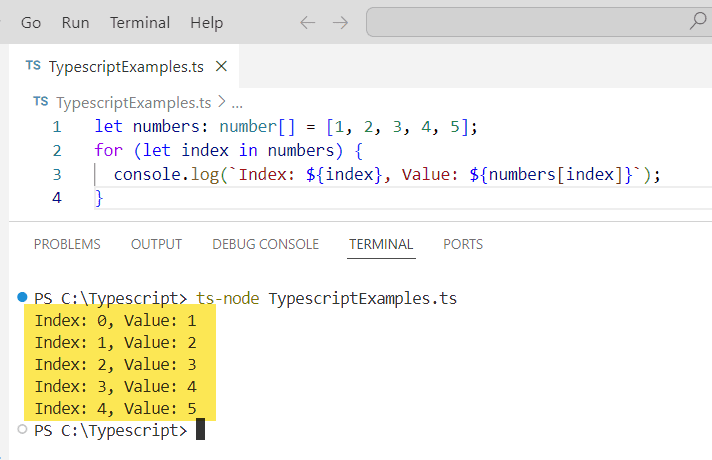
2. Iterating Over Strings
Strings in TypeScript can be treated as arrays of characters. The for...of
loop can be used to iterate over each character in the string.
let message: string = "Hello";
for (let char of message) {
console.log(char);
}
This approach is ideal for processing each character individually. It can help check if the string contains specific characters or transform the string.
3. Iterating Over Tuple Types
Tuples in TypeScript are fixed-size collections of values that can be of different types. Iterating over tuples can be done using a for...of
loop.
let point: [number, number] = [5, 10];
for (let value of point) {
console.log(value);
}
Tuples’ specific element types make them useful when the data structure has a known quantity and type of elements, such as coordinates or pairs.
4. Iterate Over Map and Set Objects
Maps and sets in TypeScript provide unique ways to handle key-value pairs and unique values, respectively. For Map
objects, the for...of
loop can iterate over either keys, values, or entries.
let exampleMap: Map<string, number> = new Map([["a", 1], ["b", 2]]);
for (let [key, value] of exampleMap.entries()) {
console.log(`Key: ${key}, Value: ${value}`);
}
Sets, like maps, can be iterated using for...of
loops. Since sets only contain values, the iteration is straightforward.
let exampleSet: Set<number> = new Set([1, 2, 3]);
for (let value of exampleSet) {
console.log(value);
}
This straightforward approach makes maps and sets powerful and versatile for storing and processing collections of unique items and pairs.
Control Flow Within For Loops in Typescript
In TypeScript, for
loops allow developers to write concise and controlled iterations. By using break
and continue
statements, it’s possible to manage the flow of these loops effectively.
Using Break to Terminate Loops
The break
statement exits the loop before it completes all iterations. This is useful when a certain condition is met, and continuing the loop is unnecessary. For instance, finding a particular value in an array:
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] === 3) {
console.log("Found 3 at index", i);
break; // Exit the loop when value is found
}
}
Here, the break
stops the loop once it finds the number 3, preventing further iterations. This can enhance performance by reducing the number of unnecessary checks.
Using Continue for Skipping Iterations
The continue statement skips the current iteration and proceeds with the next one. This statement is useful for skipping specific values or conditions:
let numbers = [1, 2, 3, 4, 5];
for (let i = 0; i < numbers.length; i++) {
if (numbers[i] % 2 === 0) {
continue; // Skip even numbers
}
console.log("Odd number:", numbers[i]);
}
In this example, continue
skips any even number, ensuring that the console.log
statement only runs for odd numbers.
Typescript For Loop Examples and Patterns
For loops in TypeScript are essential for iterating over arrays, lists, and other collections. Let’s look at some examples of how to use TypeScript for loops.
Looping with Indexes
Looping with indexes is common when you need precise control over the number of iterations. A for loop in TypeScript often starts by initializing a counter variable.
let numbers = [10, 20, 30, 40, 50];
for (let i = 0; i < numbers.length; i++) {
console.log(numbers[i]);
}
In this example, i
is the loop counter, starting from 0 and incrementing by 1 until it reaches the length of the array. This loop will log each number in the given array.
Nested For Loops
Nested for loops are useful when working with multidimensional arrays or performing complex iterations. Each nested loop should have its own counter variable.
let matrix = [
[1, 2, 3],
[4, 5, 6],
[7, 8, 9]
];
for (let i = 0; i < matrix.length; i++) {
for (let j = 0; j < matrix[i].length; j++) {
console.log(matrix[i][j]);
}
}
In this example, i
iterates over rows and j
iterates over columns, allowing access to each element in a 2D array.
Conditional Execution within For Loops
Adding conditions within for loops helps to control the flow of iterations based on specific requirements. This ensures that only certain elements are processed.
let values = [10, 15, 20, 25, 30];
for (let i = 0; i < values.length; i++) {
if (values[i] > 20) {
console.log(values[i]);
}
}
Here, the condition values[i] > 20
ensures that only values greater than 20 are logged to the console.
You can see the output in the screenshot below; I executed the above code.
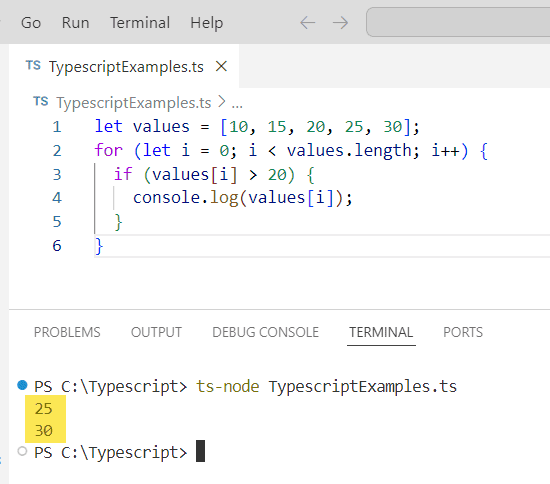
Updating Loop Variables
Updating loop variables within the loop body can change how the loop behaves, such as skipping certain iterations or updating the counter in atypical ways.
for (let i = 0; i < 10; i++) {
console.log(i);
if (i % 2 === 0) {
i++;
}
}
In this example, the loop counter i
is incremented additionally inside the loop when it is even. This results in the loop skipping the next index, leading to unique iteration patterns.
By mastering for loop examples and patterns, developers can optimize their TypeScript code to effectively handle different data structures and iteration needs.
Conclusion
In TypeScript, the for loop is a fundamental tool for performing repeated tasks.
It allows precise control over iteration, making it suitable for many programming scenarios.
Recap of main points:
- The traditional
for
loop: Ideal for situations where the number of iterations is known. for...of
loop: Simplifies iterating over array elements.for...in
loop: Used for iterating over object properties.
Understanding these loops can help programmers write clearer and more efficient code.
Developers often have questions about which loop to use.
Here’s a brief comparison:
Loop Type | Use Case | Example Code |
---|---|---|
for | When the number of iterations is known. | for (let i = 0; i < 5; i++) { console.log(i); } |
for...of | When iterating over array elements. | for (let value of myArray) { console.log(value); } |
for...in | When iterating over object properties. | for (let key in myObject) { console.log(key); } |
These examples show how to use the TypeScript loops. TypeScript makes looping through arrays and objects straightforward and powerful.
I hope you learn now how to use the loops in Typescript with these real examples.
You may also like the following tutorials:
- How to Convert String to Number in TypeScript?
- How to Convert String to Date in TypeScript?
- How to Merge Two Objects in TypeScript?
- TypeScript Exclamation Mark
- How to Merge Object Arrays Without Duplicates in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com