In this Typescript tutorial, I will explain to you everything about the TypeScript Exclamation Mark or TypeScript Non-null Assertion Operator.
In TypeScript, the exclamation mark (!) is known as the non-null assertion operator. It is used after a variable to tell the TypeScript compiler that the developer is certain the value is not null or undefined, effectively removing the type null and undefined from the type of the variable.
TypeScript Exclamation Mark
In TypeScript, the exclamation mark (!
) is used to tell the compiler that you are certain that an expression does not evaluate to null
or undefined
. It’s a way to assure the TypeScript type checker that you’ve performed the necessary checks or you are confident that a variable will not be null
or undefined
at runtime.
Here’s a simple example to illustrate:
let userInput!: string; // Non-null assertion operator
userInput = getUserInput(); // Assume this function returns a string
console.log(userInput.trim()); // No error because userInput is asserted not to be null
In this example, we used the !
operator when declaring userInput
. By doing so, we’re telling TypeScript that userInput
will not be null
or undefined
when we try to call .trim()
on it.
Why Use the Exclamation Mark in Typescript?
TypeScript’s strict null-checking feature can prevent many runtime errors by ensuring variables are not null
or undefined
before you try to use them. However, there are cases where you know more about the presence of a value than TypeScript can infer. In these cases, the exclamation mark comes in handy.
For instance, when interacting with the DOM, you might be certain that an element exists:
const myElement = document.getElementById('myElementId')!;
console.log(myElement.tagName); // No error
Without the exclamation mark, TypeScript would complain that myElement
could potentially be null
.
TypeScript Exclamation Mark: Declaration, Syntax, and Basic Use
Declaration: In TypeScript, a variable can be declared with the potential to be null
or undefined
. The non-null assertion operator directly follows the variable or property name without any spaces and asserts that the value is not null or undefined.
Syntax: The operator is a single exclamation mark !
placed after a variable’s name or property before its usage.
Basic Use:
- Purpose: It tells the TypeScript compiler to suspend strict null checks for the following expression temporarily.
- Functionality: By using the exclamation mark operator, it informs the TypeScript compiler the developer asserts the value to be non-null or non-undefined.
Here’s how it might look in code:
let userInput: string | null = document.getElementById('userInput')!.value;
Above, document.getElementById('userInput')
could potentially return null
, but adding !
after it (and before accessing .value
) asserts that it won’t be null or undefined at that point.
Errors: Without the non-null assertion operator, TypeScript may raise an error if strict null checks are enabled and a variable could be null
or undefined
. Using the exclamation mark operator prevents such errors when a developer knows there will be a value.
TypeScript Exclamation Mark Operator
In TypeScript, developers often encounter nullable types, which may lead to compile-time errors if not handled properly. The exclamation mark (!
), known as the non-null assertion operator, plays a crucial role in handling such cases. When a variable or property is appended with !
, TypeScript interprets this as the developer asserting that the value is not null
or undefined
.
For example:
let exampleVariable: string | undefined;
exampleVariable = "This is a string";
console.log(exampleVariable!.length); // Using ! to assert non-null value
Here you can see the output in the screenshot below:
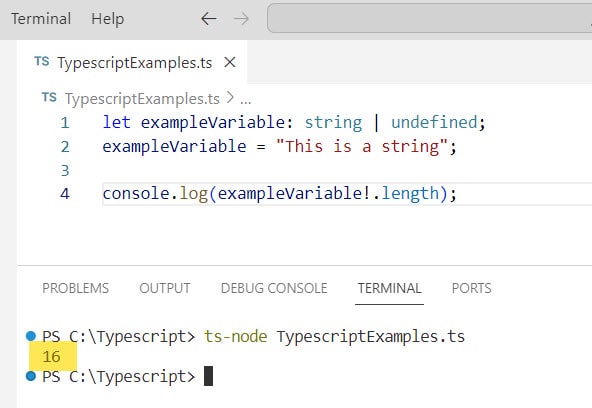
Here’s a brief breakdown of its purpose and usage:
- Purpose: To inform the TypeScript compiler that the developer guarantees a value is not null or undefined.
- Usage: Applicable when TypeScript’s strict null checks are enabled, and the developer has clear knowledge that a variable will not be null or undefined at runtime.
However, developers should exercise caution when utilizing the non-null assertion operator for the following reasons:
- Overuse risks: Overusing
!
can undermine the robustness of type-checking, potentially leading to runtime errors. - Better alternatives: Proper type checks and narrowing are often preferable to guarantee type safety.
Below is a summary table to highlight the operator’s essence:
Operator | Syntax Usage | Purpose |
---|---|---|
! | variable! or object!.property | Assures the compiler the value is not null or undefined |
In practice, while the operator is helpful, it should be used sparingly. A developer should only resort to it when they are confident that the value at the point of use will not be null or undefined and when other type-checking measures are not feasible.
TypeScript Exclamation Mark After Variable
In TypeScript, the exclamation mark (!
) after a variable is called the non-null assertion operator. This operator is used when the developer is certain that a variable, which possibly could be null
or undefined
, is in fact not. By placing !
directly after a variable or property name, the compiler is instructed to trust the developer’s assertion.
Common Usage and Syntax
- Basic Syntax
variable!
: Asserts thatvariable
is notnull
orundefined
.
- Before Property Access
object!.property
: Accessesproperty
with the certainty thatobject
is notnull
orundefined
.
Practical Examples
Variable Assignment:
let input: string | null = document.querySelector('#input-field')!.value;
The !
indicates to TypeScript that #input-field
exists.
Function Parameters:
function processUser(user: User | null) {
let userId = user!.id;
// ...
}
The !
asserts user
is not null
within the function.
TypeScript Exclamation Mark Before Variable
In TypeScript, when a developer places an exclamation mark (!
) before a variable, it is known as a non-null assertion operator. This syntax serves as a directive to the TypeScript compiler, and it has a specific purpose: to indicate that the variable is guaranteed not to be null
or undefined
.
Usage Example:
let employeeName: string | undefined;
employeeName = getEmployeeName();
// Using the exclamation mark to assert non-null/undefined
console.log(employeeName!.toUpperCase());
In the example above, employeeName
could potentially be undefined
. However, by using employeeName!
, the developer asserts that, at this point in the code, employeeName
will definitely hold a string
.
Use Cases and Benefits:
- Avoids compiler errors: This is especially useful when interacting with DOM elements that TypeScript isn’t aware of.
- Conveys developer’s intent: Signifies the developer is confident about the presence of a value.
Risks and Considerations:
- Runtime errors: If the assertion is incorrect, this can lead to runtime errors.
- Usage restraint: It should be used sparingly, as overuse can undermine TypeScript’s null-safety guarantees.
In summary, the non-null assertion operator is a means for developers to communicate their certainty about the presence of a value to the TypeScript compiler. It is a valuable tool but should be used with a clear understanding of the potential runtime implications.
TypeScript Exclamation Mark at the End
In TypeScript, the exclamation mark at the end of a variable – known as the non-null assertion operator – plays a critical role in type safety. Coders use it when they are certain that an expression does not evaluate to null
or undefined
. This operator effectively informs the TypeScript compiler to trust the developer’s assertion that a value will exist at runtime.
Example Usage:
let user: User | undefined;
user = getUser();
let username: string = user!.name; // Asserting that user is not undefined
The expression user!.name
signals that user
is not null
or undefined
. Here’s the breakdown of the syntax:
user
is the variable that might benull
orundefined
.!
is the non-null assertion operator.name
is the property being accessed.
Best Practices
- Do Use Sparingly: This operator should be used sparingly as it bypasses TypeScript’s type checks.
- Do Not Assume: Avoid assuming that an object is not
null
orundefined
– only use!
when there’s certainty. - Do Validate: Before using
!
, consider runtime checks for a more robust application.
Typescript Exclamation Mark in Class Property
In TypeScript, developers occasionally encounter scenarios where they are certain about the presence of a class property’s value, yet the compiler requires an explicit declaration to confirm it. This is where the exclamation mark (!
), known as the definite assignment assertion operator, comes into play.
When a developer uses the exclamation mark in a class property, they inform the TypeScript compiler that the property will certainly be assigned by the time the class is fully initialized, even if it’s not immediately clear from the class constructor. Here is a typical usage:
class Example {
property!: string;
}
The syntax above does two things:
- Asserts Non-Null: It tells the TypeScript compiler that
property
will not remainnull
orundefined
. - Suppresses Initialization Checks: The compiler will not emit an error complaining about
property
lacking an initializer.
The implications of using the exclamation mark are clear. Its presence indicates confidence in the initialization without the explicit need for redundant checks. It is particularly useful when utilizing libraries or frameworks where the property might be set by library code outside of the constructor, or through dependency injection.
Key Points:
- The exclamation mark is a non-null assertion on class properties.
- Prevents the TypeScript compiler from generating initialization errors.
- Use with caution: Incorrect usage can lead to runtime errors if assumptions about assignment prove incorrect.
It is paramount for developers to use this operator with a full understanding of their codebase to prevent unforeseen runtime errors due to improper assumptions about property assignments.
TypeScript Exclamation Mark in Type Definition
The TypeScript exclamation mark, when used in type definitions, serves a specific purpose. It is important to distinguish its function from its general use as a non-null assertion operator. In type definitions, the exclamation mark indicates that a property is definite; that is, it must be initialized by the time the constructor finishes executing. This use of the exclamation mark is part of TypeScript’s syntax for defining the shape of objects.
Usage in Class Property Declarations:
When a class is defined in TypeScript, class properties might be declared without initialization. However, TypeScript’s strict property initialization checks will enforce that these properties are assigned values in the constructor. Bypassing this behavior is possible by using an exclamation mark after the property name:
class Person {
name!: string;
age!: number;
}
In this example, name
and age
are marked as definite assignments, even though they are not immediately initialized.
Implications for Developers:
Developers using this feature should be cautious. The responsibility is on them to ensure that the properties are indeed initialized before they’re accessed. TypeScript’s compiler trusts the developer’s assertion and will not perform the usual strict initialization checks.
TypeScript Versions:
The definite assignment assertion was introduced in TypeScript 2.7, providing developers with more flexibility and control over class properties during type definition. It is particularly useful when integrating TypeScript with libraries or patterns that initialize class properties outside of the constructor, such as dependency injection or property decorators.
Non-null Assertion Operator Best Practices
In TypeScript, using the non-null assertion operator judiciously is crucial for maintaining type safety. It allows a developer to assert that a value is not null or undefined, bypassing the compiler’s checks.
Appropriate Usage
When a developer is certain a value will not be null or undefined, but TypeScript’s static analysis is unable to infer this, the non-null assertion operator can be used. It is essentially a ‘trust me’ note to the compiler. Here are situations that qualify as appropriate usage:
- Interacting with DOM Elements: After querying a DOM element that you know exists, like an element with an
id
present on the page.
const myElement = document.getElementById('myElement')!;
- Initialization in Lifecycle Hooks: When a value is initialized in a framework’s lifecycle hook and used afterward, assuming the framework ensures initialization before usage.
class Component {
private element!: HTMLElement;
onInit() {
this.element = document.getElementById('known-element');
}
}
Common Misuses
The misuse of the non-null assertion operator can lead to runtime errors that defy TypeScript’s purpose of enhancing type safety. Below are frequent misuses:
Preemptive Assertions: Asserting non-null before any logical guarantees are established. This practice should be avoided to not undermine TypeScript’s type safety.
// Avoid doing this
let risky: string | undefined;
console.log(risky!.trim());
Ignoring Compiler Warnings: The compiler’s inability to recognize a non-null value might be due to missing type information or incorrect logic. Blindly using the non-null assertion operator without investigation can lead to errors.
// Investigate why the compiler is unsure about the type before asserting
let user = findUser();
console.log(user!.name);
TypeScript Exclamation Mark vs Question Mark
In TypeScript, the exclamation mark (!
) and question mark (?
) serve different purposes related to variable declarations and type checking. The exclamation mark is known as the non-null assertion operator while the question mark denotes optional properties or parameters.
Non-null Assertion Operator !
:
- It informs TypeScript that the developer guarantees a value is not
null
orundefined
. - Commonly used when TypeScript’s strict null checks are enabled, and the developer knows more about the presence of a value than TypeScript can infer.
- Used as a postfix (e.g.,
variable!
) to removenull
andundefined
from the type of an identifier. - It should be used with caution as it overrides TypeScript’s inherent safety checks.
Optional Property/Parameter ?
:
- Indicates that a property, parameter, or variable might be
undefined
, allowing for the absence of a value. - Used in interfaces, function declarations, and variable declarations (e.g.,
function example(arg?: number)
). - It enables safe, gradual adoption of strict types and facilitates optional chaining (
?.
) for accessing nested properties.
Exclamation Mark ! | Question Mark ? | |
---|---|---|
Use | Guarantee non-null | Denote optionality |
Type | Remove null /undefined | Allow null /undefined |
Syntax | Postfix (e.g., variable! ) | Prefix (e.g., variable?: type ) |
Employing these operators requires an understanding of TypeScript’s type system and the domain context. The exclamation mark should be used sparingly to avoid bypassing the compiler checks inadvertently. Conversely, the question mark creates a more forgiving interface for values that may or may not be present.
Conclusion
The TypeScript exclamation mark, a non-null assertion operator, is crucial in type safety. Its primary function is to inform the compiler that the developer guarantees the value is not null or undefined. It’s an explicit way to circumvent TypeScript’s strict null-checking, allowing flexibility in specific scenarios without disabling typechecks entirely.
One must handle this operator carefully, as improper use can lead to unexpected runtime errors. Here are key points to remember:
- Use Sparingly: Reserve the exclamation mark for instances where the developer has absolute certainty about the presence of a value.
- Exception, Not Norm: Treat it as an exception to the rule of strict type-checking, not as a regular coding practice.
- Safety First: Prioritize maintaining type safety whenever possible to leverage TypeScript’s full potential.
In this Typescript tutorial, I will explain everything about the Typescript exclamation mark or the TypeScript Non-null Assertion Operator.
You may also like:
- Split String by Regex in TypeScript
- TypeScript Split String Into Array
- TypeScript Enum Reverse Mapping
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com