Do you want to convert a string to a boolean in Typescript? This Typescript tutorial explains how to convert string to boolean in Typescript using different methods. With examples, we will discuss everything about “Typescript string to boolean”.
To convert a string to a boolean in TypeScript, you can use the comparison method: simply compare the string directly to "true"
or "false"
. This method is straightforward and works best when you’re certain of the input format. For example, return str === "true"
will return true
if the string is exactly "true"
and false
otherwise, making it a reliable and simple solution for controlled inputs.
Convert string to boolean in Typescript
Now, let us see how to convert a string to a boolean in Typescript using various methods.
In TypeScript, a boolean is a data type that can hold one of two values: true
or false
. This is crucial in control flow and logic.
Method 1: Using Comparison
The simplest method to convert string to bool in Typescript is to compare the string to or directly
function stringToBoolean(str: string): boolean {
return str === "true";
}
console.log(stringToBoolean("true"));
console.log(stringToBoolean("false"));
After I ran the code using Visual Studio code, you can see the output in the screenshot below:
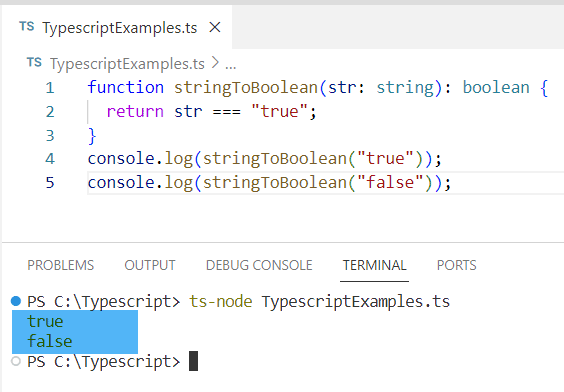
Method 2: The Boolean Constructor
TypeScript allows using the Boolean constructor, but with a caveat. It converts non-empty strings to true
and empty strings to false
. Here is a complete example of string to boolean in Typescript.
function stringToBoolean(str: string): boolean {
return Boolean(str);
}
console.log(stringToBoolean("hello"));
console.log(stringToBoolean(""));
Here is the output in the screenshot below after I ran the code using Visual Studio Code.
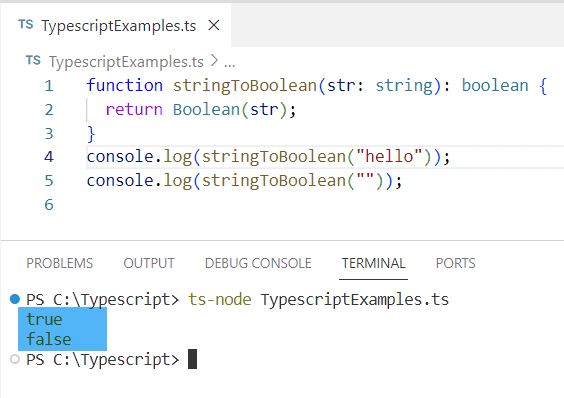
Method 3: Using a Ternary Operator
For more control over which strings convert to true
or false
, use a ternary operator in Typescript. Here is an example of “Typescript convert string to boolean” with complete code:
function stringToBoolean(str: string): boolean {
return (str.toLowerCase() === "true") ? true : false;
}
console.log(stringToBoolean("True"));
console.log(stringToBoolean("FALSE"));
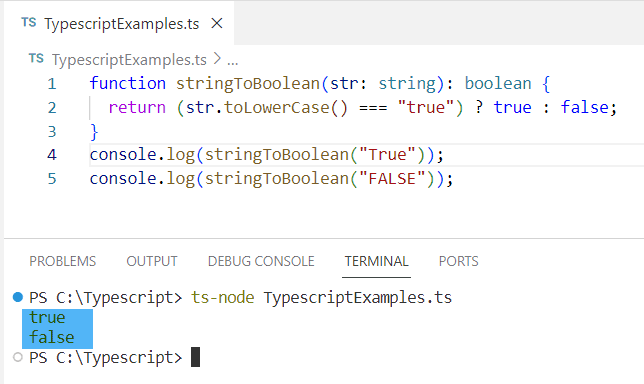
Method 4: Using a Switch Case
You can also use a switch case in Typescript to convert a string to a boolean. This method is useful when dealing with multiple true or false string representations. Here is an example.
function stringToBoolean(str: string): boolean {
switch(str.toLowerCase()) {
case "true":
case "yes":
case "1":
return true;
case "false":
case "no":
case "0":
return false;
default:
throw new Error("Invalid input");
}
}
console.log(stringToBoolean("yes"));
console.log(stringToBoolean("0"));
You can see the output in the screenshot below after I ran the code using Visual Studio code.
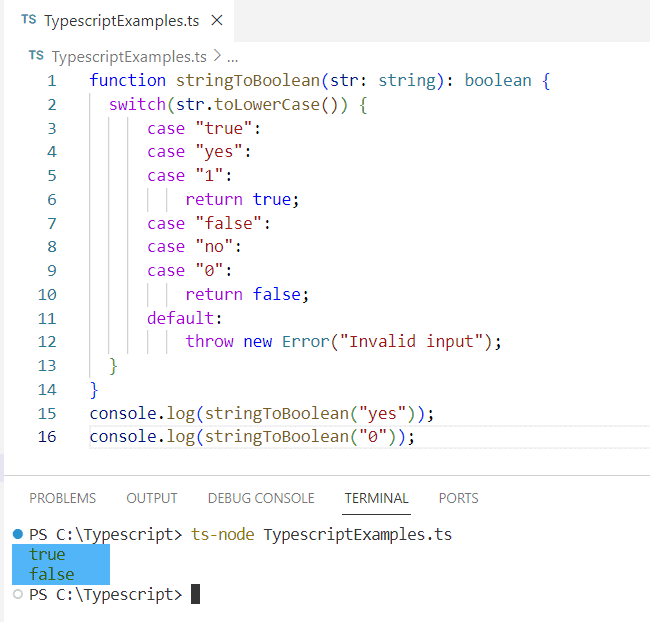
Method 5: Using JSON.parse()
To convert a string to a boolean in Typescript, you can utilize the JSON.parse()
method. This method parses a string as JSON and can interpret the text "true"
and "false"
as their respective boolean values.
let boolVal: boolean = JSON.parse("true"); // true
console.log(boolVal);
let boolVal1: boolean = JSON.parse("false"); // false
console.log(boolVal1);
After I executed the code using Visual Studio Code, you can see the output in the screenshot below:
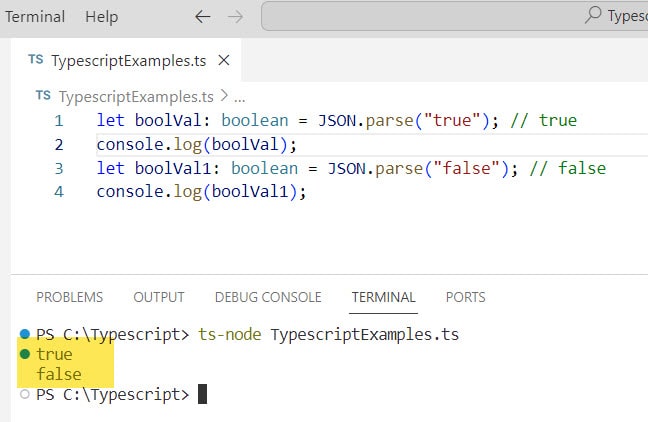
Conclusion
Converting a string to a boolean in TypeScript can be achieved through various methods, each with its own use case. In this Typescript tutorial, I have explained how to convert a string to a boolean in Typescript using various methods:
- Using Comparison
- The Boolean Constructor
- Using a Ternary Operator
- Using a Switch Case
- Using JSON.parse()
You may like the following tutorials:
- TypeScript Enum vs Union Type
- boolean Keyword in Typescript
- Typescript Split String
- Typescript split string by dot
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com