When working with TypeScript, a common task encountered by developers is the need to manipulate strings, particularly splitting them into substrings based on certain delimiters. In this Typescript tutorial, I will explain how to split string by dot in Typescript.
To split a string by a dot in TypeScript, you can use the split method of the String object. Simply call myString.split(‘.’), where myString is your original string. This will return an array of substrings divided at each occurrence of the dot. If you need to handle special cases, such as ignoring multiple consecutive dots, you can use a regular expression with the split method, like myString.split(/./).
Typescript split string by dot
TypeScript, being a superset of JavaScript, provides the same string manipulation capabilities as JavaScript. We will explore different methods to achieve our goal, including the use of the split
method, regular expressions, and third-party libraries.
Here is an example of a Typescript split string by dot.
Method 1: Using the split
() Method
The most straightforward way to split a string by a dot in TypeScript is by using the built-in split
method. This method is available on any string instance and splits the string into an array of substrings based on the delimiter provided.
Here’s an example:
function splitStringByDot(input: string): string[] {
return input.split('.');
}
const myString = "www.example.com";
const parts = splitStringByDot(myString);
console.log(parts);
In this example, the splitStringByDot
() function takes a string as input and returns an array of substrings split by the dot in Typescript. When we pass “www.example.com” to this function, it returns an array with three elements: “www”, “example”, and “com”. You can see the screenshot below for the output:
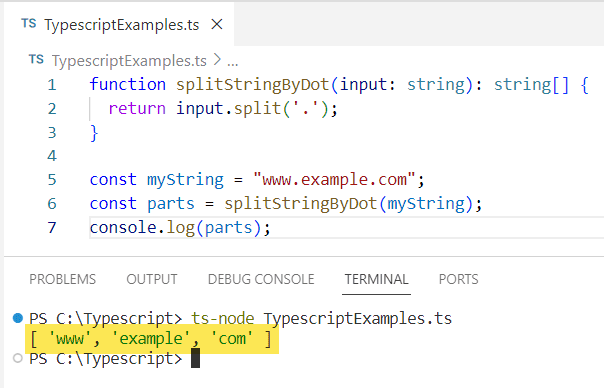
Method 2: Using Regular Expressions
Sometimes, you might need a more powerful tool for string splitting, especially if there are special cases to consider. Regular expressions come in handy for such scenarios. In TypeScript, you can use a regular expression with the split
method to split a string.
Here’s how you can do it:
function splitStringByDotUsingRegex(input: string): string[] {
// This regular expression matches a dot character in the string
const regex = /\./;
return input.split(regex);
}
const myString = "www.example.com";
const parts = splitStringByDotUsingRegex(myString);
console.log(parts);
The regular expression /\./
is used to match the dot character because in regular expressions, the dot is a special character that matches any character except new lines. To match a literal dot, we need to escape it with a backslash (\
). For the output, check the screenshot below:
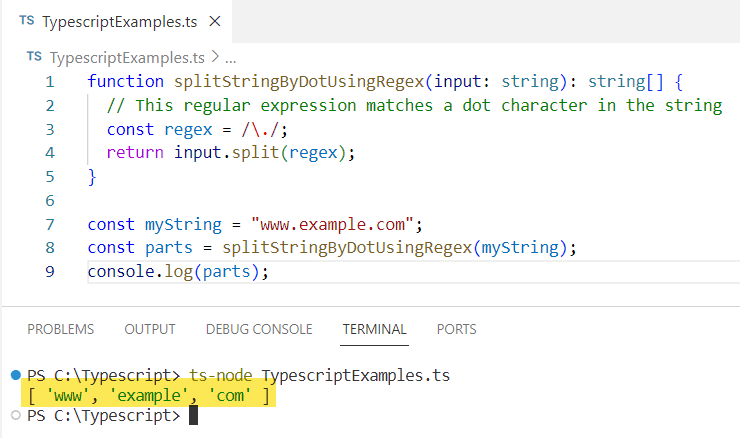
Method 3: Handling Edge Cases
When splitting strings in Typescript, we might encounter edge cases such as leading or trailing dots, or multiple consecutive dots. It’s important to decide how to handle these cases in your application. Here’s an example that deals with consecutive dots in a Typescript string:
function splitStringByDotHandlingConsecutive(input: string): string[] {
// This regular expression matches one or more consecutive dots
const regex = /\.+/;
return input.split(regex);
}
const myString = "www...example...com";
const parts = splitStringByDotHandlingConsecutive(myString);
console.log(parts);
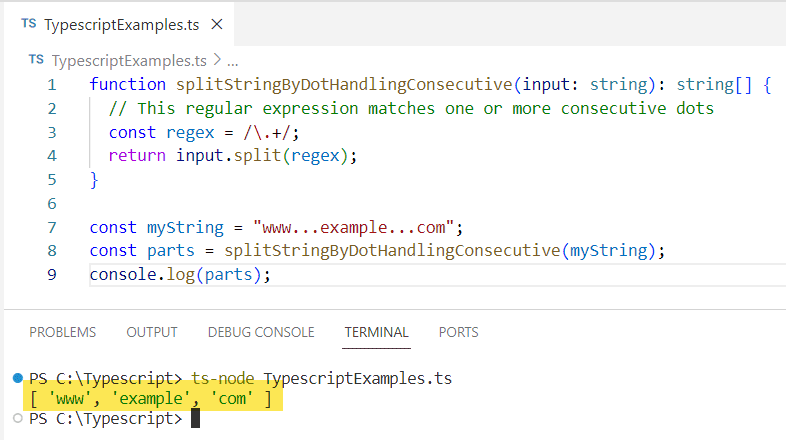
In this example, the regular expression /\./
has been modified to `/.+/’ to match one or more consecutive dots. This way, the input string “www…example…com” is split into three parts, ignoring the extra dots
Method 4: Using Third-Party Libraries
While TypeScript and JavaScript provide powerful native methods to manipulate strings, sometimes you might prefer using a third-party library for additional functionality or more readable code. One such popular library is Lodash, which provides a variety of utility functions.
Here’s an example using Lodash’s split
function:
import * as _ from 'lodash';
function splitStringByDotUsingLodash(input: string): string[] {
return _.split(input, '.');
}
const myString = "www.example.com";
const parts = splitStringByDotUsingLodash(myString);
console.log(parts);
.
Conclusion
Splitting a string by a dot in TypeScript is a common and straightforward task that can be accomplished using the native split
method, regular expressions, or third-party libraries like Lodash. In this Typescript tutorial, I have explained with examples how to split string by dot in Typescript.
You may also like:
- Typescript check if a string contains a substring
- Typescript split string multiple separators
- Typescript remove quotes from string
- How to Split String by Length in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com