When working with arrays of objects, we often need to ensure that the merged array does not contain duplicate objects. Here, we’ll explore a method to merge object arrays without duplicates in TypeScript.
To merge object arrays without duplicates in TypeScript, you can use the Set
object in combination with the spread operator to filter out duplicate objects based on a unique property. Define a type for your objects, then merge the arrays and use a Set
to keep track of unique property values, pushing only non-duplicate objects into the final array. This method ensures that each object in the merged array is unique with respect to the specified property.
TypeScript Merge Object Arrays Without Duplicates
Consider you have two arrays of objects in Typescript, where each object has a unique identifier. The goal is to merge these arrays into one, ensuring that objects with the same identifier only appear once.
To merge arrays without duplicates in Typescript, we’ll use the power of TypeScript’s type system along with ES6 features like the spread operator and Set
.
Follow the below steps to merge object arrays without duplicates in Typescript.
Step 1: Define the Object Type
First, we need to define the type of objects we’re working with. For this example, let’s assume we have an array of User
objects in Typescript.
type User = {
id: number;
name: string;
};
Step 2: Create Two Arrays of Users
Next, we’ll create two arrays of User
objects to merge in Typescript.
const users1: User[] = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
];
const users2: User[] = [
{ id: 2, name: 'Jane Smith' },
{ id: 3, name: 'Emily Johnson' }
];
Step 3: Merge and Remove Duplicates
Now, let’s write a function to merge these arrays and remove duplicates based on the id
property.
function mergeUserArrays(array1: User[], array2: User[]): User[] {
const combinedArray: User[] = [...array1, ...array2];
const uniqueUsers: User[] = [];
const ids = new Set<number>();
for (const user of combinedArray) {
if (!ids.has(user.id)) {
uniqueUsers.push(user);
ids.add(user.id);
}
}
return uniqueUsers;
}
In this function, we first combine the two arrays using the spread operator. Then, we iterate over the combined array, checking if we’ve already encountered each user’s id
using a Set
. If not, we add the user to the uniqueUsers
array and record their id
in the Set
.
Step 4: Test the Function
Finally, we can test our function to ensure it works as expected.
const mergedUsers = mergeUserArrays(users1, users2);
console.log(mergedUsers);
Here is the complete Typescript code.
type User = {
id: number;
name: string;
};
const users1: User[] = [
{ id: 1, name: 'John Doe' },
{ id: 2, name: 'Jane Smith' }
];
const users2: User[] = [
{ id: 2, name: 'Jane Smith' },
{ id: 3, name: 'Emily Johnson' }
];
function mergeUserArrays(array1: User[], array2: User[]): User[] {
const combinedArray: User[] = [...array1, ...array2];
const uniqueUsers: User[] = [];
const ids = new Set<number>();
for (const user of combinedArray) {
if (!ids.has(user.id)) {
uniqueUsers.push(user);
ids.add(user.id);
}
}
return uniqueUsers;
}
const mergedUsers = mergeUserArrays(users1, users2);
console.log(mergedUsers);
Once you execute the Typescript code, the console.log
should output an array with three users, having removed the duplicate Jane Smith
object. You can see the output in the screenshot below:
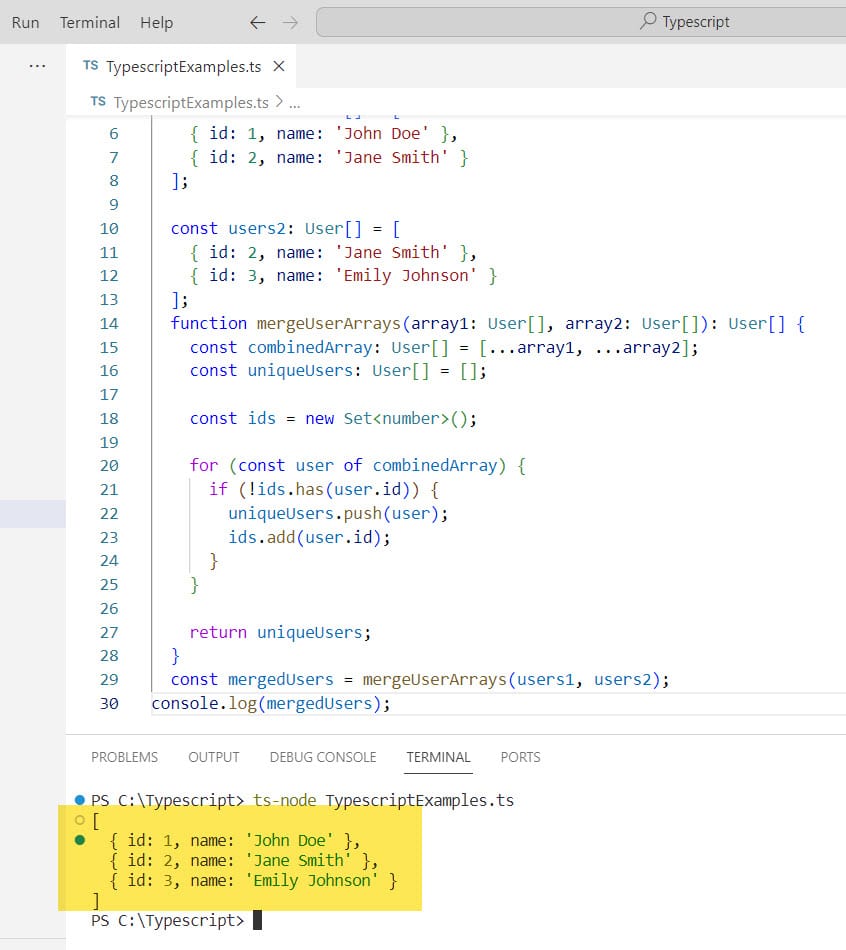
[
{ "id": 1, "name": "John Doe" },
{ "id": 2, "name": "Jane Smith" },
{ "id": 3, "name": "Emily Johnson" }
]
Conclusion
Merging object arrays without duplicates in TypeScript is straightforward when you combine ES6 features with TypeScript’s type system. By using the spread operator to combine arrays and a Set
to track unique identifiers, you can ensure that your merged array only contains unique objects. In this Typescript tutorial, I have explained in detail how to merge object arrays without duplicates in Typescript.
You may also like:
- Typescript Get Last Element of Array
- Typescript replace all occurrences in string
- Typescript merge arrays
- Typescript sort by date
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com