Do you want to know about the Typescript replaceall() method? In this Typescript tutorial, I will explain everything about Typescript replace all with examples, especially how to replace all occurrences in string in Typescript.
The Typescript replaceAll
method allows you to replace all occurrences of a specified substring within a string. It’s used as string.replaceAll(searchValue, replaceValue)
, where searchValue
is the substring you want to replace (it can be a string or a regular expression), and replaceValue
is the new string to insert. This method is especially handy for tasks like updating multiple instances of a word or pattern in a string.
Typescript replaceall
The replaceAll
method in TypeScript (and JavaScript) is used to replace all instances of a specified substring with a new substring. It’s different from the replace
method, which only replaces the first occurrence of the substring.
Syntax of replaceall() method
Here is the syntax of replaceall() method in Typescript.
string.replaceAll(searchValue, replaceValue)
searchValue
: The substring that we want to replace. It can be a string or a regular expression.replaceValue
: The string that will replace each occurrence of thesearchValue
.
Typescript replace all examples
Now, let us check out a few examples of typescript replace all. Here is a complete example for replaceall in Typescript.
let cities = "New York, Los Angeles, Chicago, New York, Houston";
let updatedCities = cities.replaceAll("New York", "San Francisco");
console.log(updatedCities);
Output:
San Francisco, Los Angeles, Chicago, San Francisco, Houston
Once you run the code using Visual Studio code, you can check the output in the screenshot below:
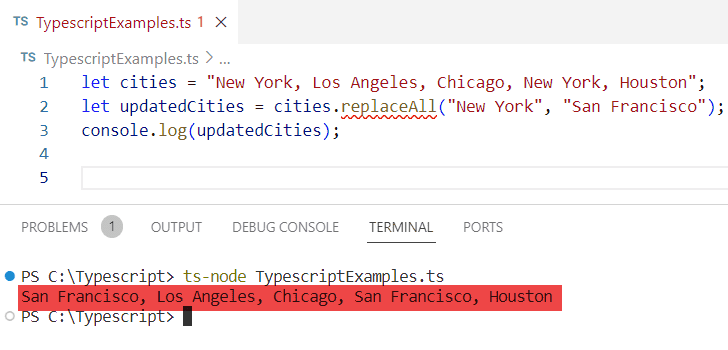
Typescript string replaceall using regular expressions
If your search string may contain special characters that have meaning in a regular expression (like .
or *
), and you want to treat them as literal characters, you should escape them before using them in replaceAll()
.
Here’s an example:
function escapeRegExp(string: string) {
// Escape special characters for use in a regular expression
return string.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
}
function replaceAllOccurrences(str: string, search: string, replacement: string): string {
// Escape special characters in 'search'
const escapedSearch = escapeRegExp(search);
// Replace all occurrences of 'escapedSearch' with 'replacement'
return str.replaceAll(escapedSearch, replacement);
}
// Example usage:
const originalString = "Visit Coeur d'Alene. Coeur d'Alene is beautiful.";
const searchString = "Coeur d'Alene";
const replacementString = "San Francisco";
const newString = replaceAllOccurrences(originalString, searchString, replacementString);
console.log(newString);
You can see the output below in the screenshot.
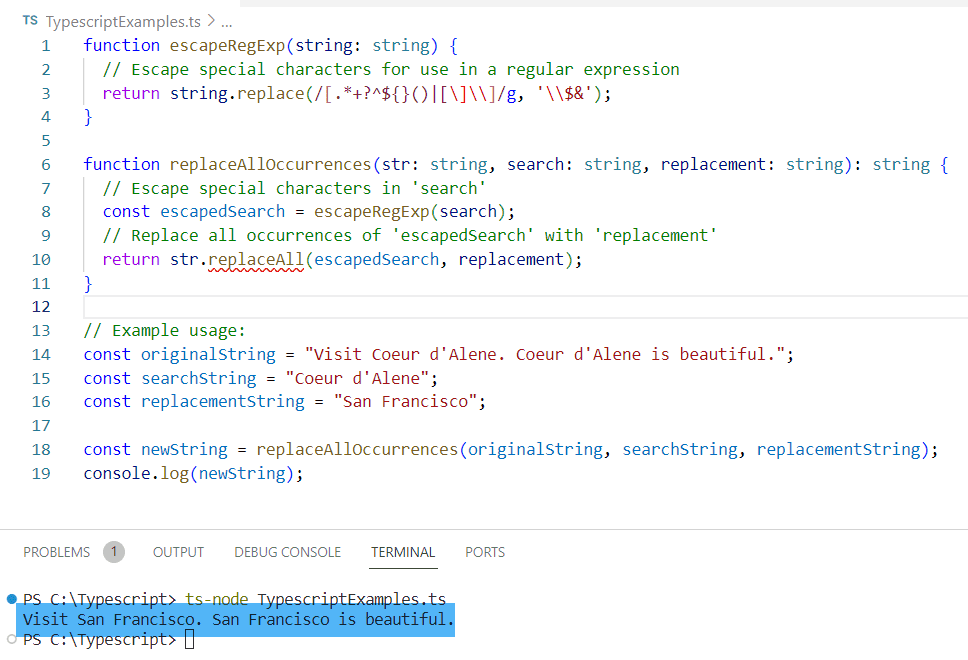
replaceall in typescript (case insensitive)
By default, replaceAll is case-sensitive. To perform a case-insensitive replacement, use a regular expression with the i flag.
let cities = "miami, Miami, MIAMI";
let updatedCities = cities.replaceAll(/miami/i, "Orlando");
console.log(updatedCities);
Typescript replace all occurrences in string
Now, let us see how to replace all occurrences in string in Typescript using various methods.
To replace all occurrences of a substring in a TypeScript string, use the replaceAll()
method for a straightforward solution, like string.replaceAll("searchString", "replaceWith")
. If you’re dealing with special characters or older versions of JavaScript, use the replace()
method with a global regular expression: string.replace(new RegExp("searchString", 'g'), "replaceWith")
. This ensures all instances of the substring are replaced efficiently.
Method-1: Using replace() method
You can use the replace() string method to replace all occurrences of a substring in a string in Typescript. By default, it replaces only the first occurrence. To replace all occurrences, you need to use a regular expression with the global (g) flag.
const originalString = "Hello World. Welcome to the World of TypeScript.";
const searchString = "World";
const replaceWith = "Universe";
const result = originalString.replace(new RegExp(searchString, 'g'), replaceWith);
console.log(result);
In this example, we create a regular expression from the searchString
and pass the 'g'
flag to replace all occurrences.
Output:
Hello Universe. Welcome to the Universe of TypeScript.
Here is the output you can see in the screenshot below:
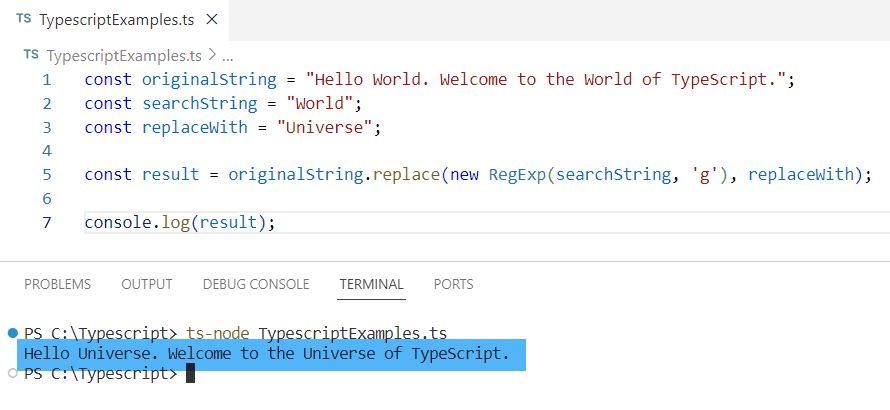
Method-2: Using String.prototype.replaceAll()
TypeScript supports the replaceAll() method, which is an easier approach to replace all occurrences without needing a regular expression.
const originalString = "Hello World. Welcome to the World of TypeScript.";
const searchString = "World";
const replaceWith = "Universe";
const result = originalString.replaceAll(searchString, replaceWith);
console.log(result);
Method-3: Handling Special Characters in Regular Expressions
If your searchString
contains special characters that are part of regex syntax (like .
or *
), you need to escape these characters. Otherwise, they might lead to unexpected behavior.
function escapeRegExp(string: string) {
return string.replace(/[.*+?^${}()|[\]\\]/g, '\\$&');
}
const originalString = "Let's learn TypeScript. TypeScript is amazing!";
const searchString = "TypeScript.";
const safeSearchString = escapeRegExp(searchString);
const replaceWith = "JavaScript.";
const result = originalString.replace(new RegExp(safeSearchString, 'g'), replaceWith);
console.log(result);
You can see the output in the screenshot below after adding in the visual studio code:
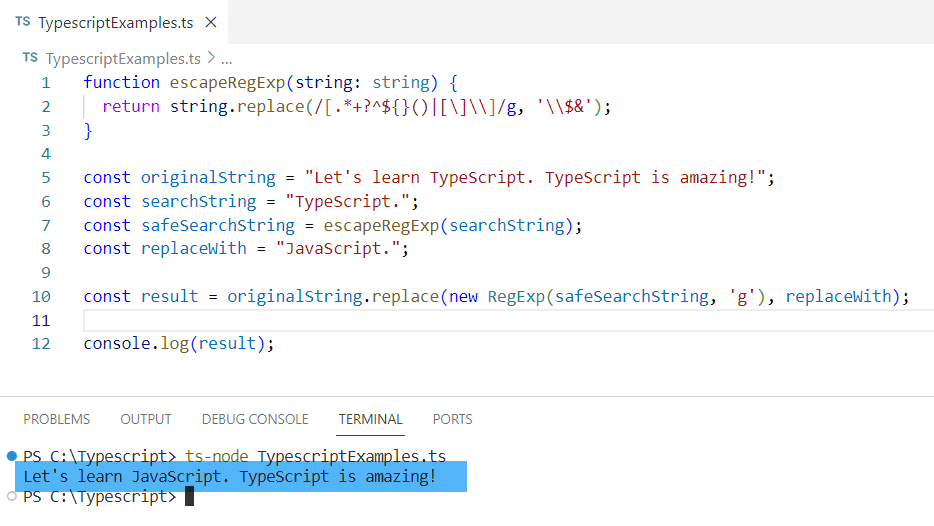
Conclusion
In this Typescript tutorial, I have explained how to use the Typescript replaceall() function with a few examples. Also, I have explained how to replace all occurrences in string in Typescript using various methods.
You may also like:
- How to check if string is empty in typescript
- How to compare two strings in typescript
- Compare two string dates in typescript
- How to remove quotes from a string in Typescript
- Typescript check if a string contains a substring
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com