Do you want to know about Typescript compare dates? In this Typescript tutorial, I will explain how to compare two dates in typescript.
Here, we will see an example of how to compare two date strings in typescript using various methods.
- strict equality check with getTime ()
- abstract equality check with getTime ()
- Greater than check(>)
- Less than check(<)
Typescript compare dates using Strict equality
Here we will see an example to compare to date strings by using a Strict equality check in typescript.
For example, we will see an example to compare two string dates in a typescript with strict equality check
In the ToCompareString.ts file, write the below code:
const firstDate = new Date('2021-05-10')
const secondDate = new Date('2021-05-10')
console.log(firstDate.getTime())
console.log(secondDate.getTime())
function dateComparison() {
// Strict equality check
if (firstDate.getTime() === secondDate.getTime()) {
console.log('Dates are the same')
} else {
console.log('Date are not the same')
}
}
dateComparison()
To compile the code, run the below command and you can see the result in the console.
ts-node ToCompareString
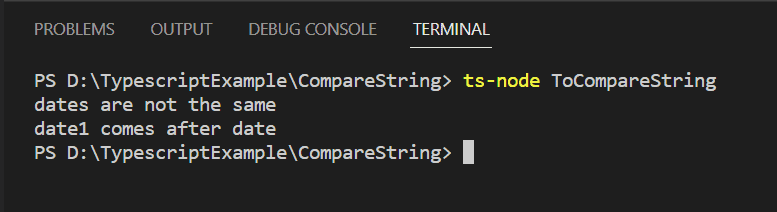
This is an example of comparing two string dates in typescript.
Compare dates in typescript using abstract equality
Here, we will compare two string dates using abstract equality check in typescript.
For example, we have two string dates, and then we will cover it to the date type by using a new Date(). Then, we will compare these two converted dates by checking the abstract equality and getTime().
Here is the getTime () will return the count of milliseconds since the timestamp, which is declared as midnight at the start of January 1, 1970, UTC. This method may be used to assist in the assignment of a date and time to another Date object
In the ToCompareString.ts file, write the below code:
const firstDate = new Date('2021-05-10')
const secondDate = new Date('2021-05-10')
console.log(firstDate.getTime())
console.log(secondDate.getTime())
function dateComparison() {
if (firstDate.getTime() == secondDate.getTime()) {
console.log('Dates are the same')
} else {
console.log('Date are not the same')
}
}
dateComparison()
To compile the code run the below command, and you can see the result in the console:
ts-node ToCompareString
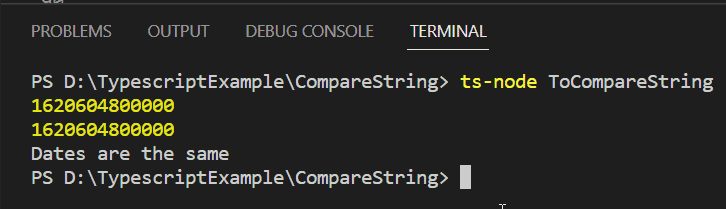
This is an example of Using an Abstract equality check to compare two string dates in typescript.
Also, check out How to remove quotes from a string in Typescript
Typescript compare dates using greater than
Here, we will compare two string dates using the greater than check method in typescript.
For example, we will take two date strings, and then we will convert the two dates of string type into date type in typescript. Then, we will check which date is greater using the greater than the operator.
In the ToCompareString.ts file, write the below code:
const firstDate = new Date('2021-05-15')
const secondDate = new Date('2021-05-12')
// console.log(firstDate.getTime())
// console.log(secondDate.getTime())
function dateComparison() {
if (firstDate> secondDate) {
console.log('firstDate is greater than SecondDate')
} else {
console.log('firstDate is not greater than SecondDate')
}
}
dateComparison()
To compile the code and run the below command and you can see the result in the console:
ts-node ToCompareString
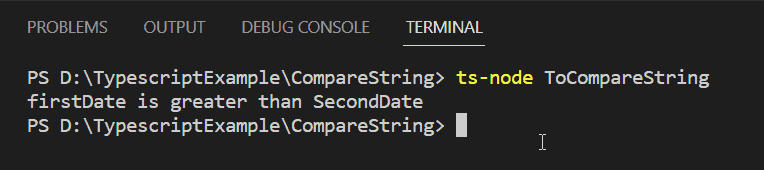
This is an example of how to compare two string dates in typescript using the greater than operator.
Typescript date comparison using less than
Here, we will compare two string dates using the less-than-check method in typescript.
For example, we will take two date strings, and then we will convert the two dates of string type into date type in typescript. Then, we will check which date1 is less than date 2 using the less than operator.
In the ToCompareString.ts file, write the below code:
const firstDate = new Date('2021-05-11')
const secondDate = new Date('2021-05-12')
// console.log(firstDate.getTime())
// console.log(secondDate.getTime())
function dateComparison() {
// 1. Abstract equality check
if (firstDate< secondDate) {
console.log('firstDate is less than SecondDate')
} else {
console.log('firstDate is not less than SecondDate')
}
}
dateComparison()
To compile the code and run the below command and you can see the result in the console:
ts-node ToCompareString
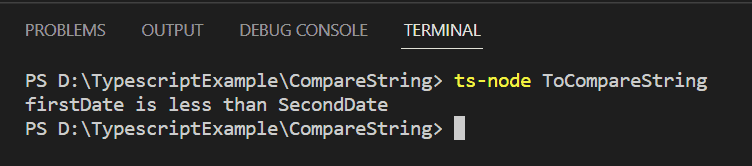
This is an example of how to compare two string dates in typescript using the less than operator.
Conclusion
In this typescript tutorial, we saw different methods to compare two date strings in typescript. Here are the methods we covered:
- strict equality check with getTime ()
- abstract equality check with getTime ()
- Greater than check(>)
- Less than check(<)
You may also like the following typescript tutorials:
- How to compare two strings in typescript
- Typescript string replaces all occurrences
- Typescript check if a string contains a substring
- How to split a string with multiple separators in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com