This typescript tutorial will see examples of how to compare two strings in typescript using operators like ‘==’ and ‘===’ and methods like localeCompare(). Here are the examples we are going to cover:
- Compare two strings in typescript
- How to compare two strings in typescript if a condition
- Check if two Strings are Equal in TypeScript
- TypeScript string compare not equal
Compare two strings in typescript
Here we will see how we can compare two strings in a typescript.
We will go through the different methods to compare two columns in typescript:
- Strict equality operator(===)
- Loose equality operator(==)
- localeCompare()
Use Strict equality operator(===) to compare two strings
So we will take two string let x and y, and then we will compare two strings using the ‘===’ operator, if the string is equal then it returns true, else false.
Open the code editor, and create the ToCompareString.ts file, and write the below code:
let x:string="Hey its typescript"
let y:string="Hey its typescript"
let z:string="typescript"
var result1= x===y;
console.log("Output of X===Y :" +result1);
var result2= x===z;
console.log("Output of X===Z :" +result2);
To compile the code and run the below command and you can see the result in the console.
ts-node ToCompileString
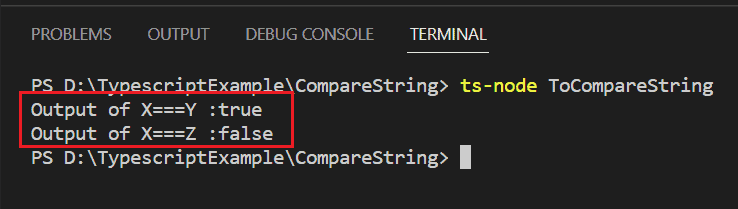
Use the loose equality operator(==) to compare two strings
We will take the same example as above, and we will use loose equality operator’==’, it will also give the same result after comparing two strings. Below you can see the code add it to the ToCompareString.ts file:
let x:string="Hey its typescript"
let y:string="Hey its typescript"
let z:string="typescript"
var result1= x==y;
console.log("Output of X==Y :" +result1);
var result2= x==z;
console.log("Output of X==Z :" +result2);
To compile the code and run the below command and you can see the result in the console.
ts-node ToCompareString
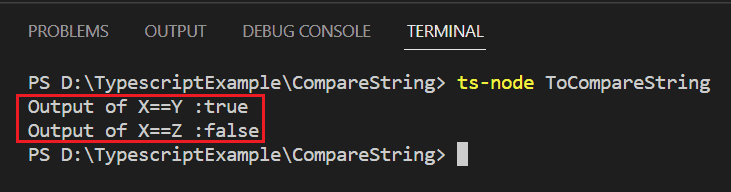
This is an example of how to Compare two strings in typescript.
Use locale compared to compare two strings
For example, we will take two strings and compare the string by using localeCompare which will returns a number showing whether a reference string occurs before, after, or is the same as the provided string, which we can get.
The syntax of localeCompare is
string.localeCompare( param )
Here param is the parameter which is a string that is used to compare with a string object.
The return value can be
- 1: There is no match, and in the locale sort order, the parameter value occurs before the string object’s value.
- 0: If the string fits 100%
- a negative value(-1): There is no match, and in the locale sort order, the parameter value occurs after the string object’s value.
In the code editor, ToCompareString.ts file, and write the below code:
var x = new String("Hey its typescript" );
var index = x.localeCompare("its typescript");
console.log("localeCompare first :" + index );
To compile the code and run the below command and you can see the result in the console:
ts-node ToCompareString
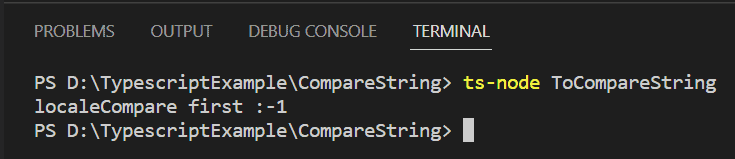
This is an example of how to compare two strings using localeCompare in typescript.
Check out, Typescript string replaces all occurrences
How to compare two strings in typescript if a condition
Here we will see an example of how to compare two strings in typescript if else.
Comparing two arrays of string in typescript is performed by reference rather than value. This implies that rather than comparing the values in the two arrays, typescript looks to see if they point to the same reference, which is frequently not the case even if the two arrays contain the same items.
This implies that when comparing two arrays in typescript using the loose or tight equality operators (== or ===), the result is frequently false.
In the ToCompareString.ts file, write the below code:
const isEquals = (x:any, y:any) => JSON.stringify(x) === JSON.stringify(y);
const x = ["Aman", "Rocky", "Alex"];
const y = ["Aman", "Rocky", "Alex"];
if (isEquals(x,y))
console.log("The arrays have the same elements.");
else
console.log("The arrays have different elements.");
To compile the code and run the below command, you can see the result in the console:
ts-node ToCompareString
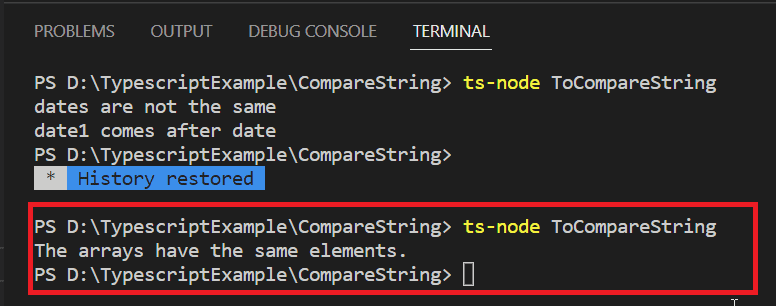
This is an example of How to compare two strings in typescript if a condition.
Check if two Strings are Equal in TypeScript
Here we will see an example to check if two strings are equal in typescript.
For example, we will take two strings, if the string is equal then it will return ‘string is equal’, else it will return ‘string is not equal’, by using the == operator in typescript.
In the ToCompareString.ts file, write the below code:
const str1:any = 'spguides';
const str2:any = 'spguides';
if (str1 == str2) {
console.log(' ✔ strings are equal');
} else {
// 👇️ this runs
console.log(' ❌strings are not equal');
}
To compile the code and run the below command, you can see the result in the console:
ts-node ToCompareString
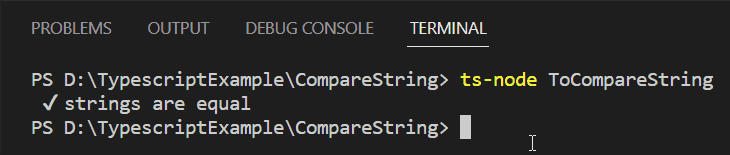
This is an example of how to check if two Strings are Equal in TypeScript.
Read How to get last element of an array in typescript
TypeScript string compare not equal
Here we will see an example of a string compares not equal in typescript.
For example, we will take two strings, and if the string is not equal then it will return ‘String is not equal’ else it returns string is equal for this we will use !== operator.
In the ToCompareString.ts file, write the below code:
const str1:any = 'spguides';
const str2:any = 'enjoysharepoint';
if (str1 !== str2) {
console.log(' ❌strings are not equal');
} else {
// 👇️ this runs
console.log(' ✔ strings are equal');
}
To compile the code and run the below command, you can see the result in the console:
ts-node ToCompareString
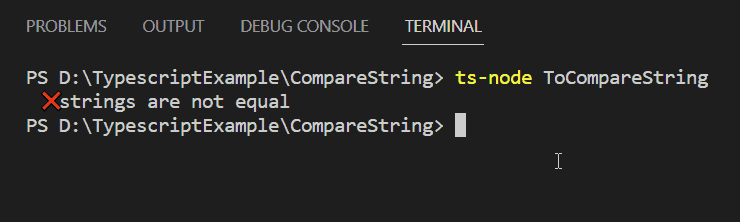
This is an example of a TypeScript string compare not equal
Conclusion
In this typescript tutorial, we saw how we can compare two strings in different methods. So here is the topic we covered :
- Compare two strings in typescript
- How to compare two strings in typescript if a condition
- Check if two Strings are Equal in TypeScript
- TypeScript string compare not equal
You may like the following typescript tutorials:
- How to check if string is empty in typescript
- Data types in typescript with examples
- How to add a property to object in Typescript
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com