In this Typescript tutorial, we will see how we can add a property to an object in Typescript. Also, we will see different examples to add a property object in typescript:
- How to add a property to an object in a typescript
- How to add a property to an object dynamically in typescript
- How to add a property to an object conditionally in typescript
- How to add a property to an object in an array in typescript
- How to add a property to object type in typescript
- How to add a property to object if not null in typescript
- How to add a property to object spread in typescript
- How to add a property to object if exists in typescript
- How to add a property to object without interface in typescript
- How to add a property to object in map in typescript
Typescript adds a property to object
Here we will see a simple example of how we can add a property to objects in typescript.
For example, we will see how we can add the age property and then both name and age property to the object in typescript.
In the code editor, create a new file objectProperties.ts, then write the below code.
interface Employee {
name: string;
age?: number; // 👈️ mark as optional so you can add later
}
const obj1: Employee = {
name: 'Henry',
};
obj1.age = 28;
// Add any property to object
const obj2: Record<string, any> = {};
// 👇️ can now add any property to the object
obj2.name = 'Tom';
obj2.age = 30;
console.log(obj1)
console.log(obj2)
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties
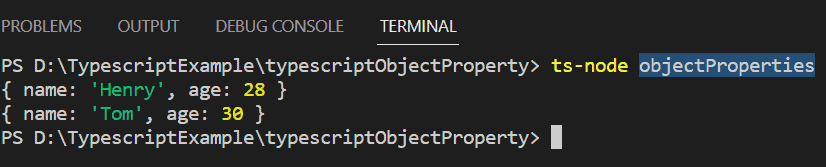
This is an example of Typescript adding a property to an object.
Read Data types in typescript with examples
Typescript adds a property to objects dynamically
Here we will see how we can add properties to objects dynamically in typescript.
By using an index signature we can dynamically add properties to an object, for example, const object: {[key: string]: any} = {}
. Index signature is used when a user doesn’t have any idea about all the names of the type’s properties and the type of their values in advance.
Let’s see an example, so, in the objectProperties.ts file write the below code:
interface Employee {
[key: string]: any;
}
const obj: Employee = {};
obj.name = 'Henry';
obj.age = 25;
console.log(obj);
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties.ts

This is an example of Typescript adds a property to objects dynamically.
Typescript add property to object conditionally
Here we will see how we can add property conditionally to an object in typescript.
For example, we will use && operator and spread operator, to add a property to an object conditionally in typescript.
Whereas the spread operator combines the properties of the destination object with the properties of the object being applied to, and if the origin object is null, no properties are added.
Let’s see the example, so, in the objectProperties.ts file write the below code:
const applyDiscount: boolean = true;
const applyTax = false;
const applyDilevery: boolean = true;
const total= {
grossAmount:100,
...(applyDiscount as boolean && {discount:'5%'}),
...(applyTax as boolean && {tax:'12%'}),
...(applyDilevery as boolean && {Dilevery:80} as object),
};
console.log(total);
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties

This is an example of Typescript add property to object conditionally.
Read Typescript type alias
Typescript adds a property to objects in an array
Here we will see how we can add a property to an object in an array in typescript
For example, we will create an Employee type as an array, this array will accept properties, i.e. name and age. So we will create and add two objects to an array, one object will contain only name properties, and age property we will add later. Then 2nd object contains both properties.
The objectProperties.ts file contains the below code:
type Employee= [{
empName: string;
age?: number; // 👈️ mark as optional so you can add later
}]
const obj1: Employee = [{
empName: 'Henry',
}];
const obj2: Employee = [{
empName: 'James',
age: 21,
}];
console.log(obj1)
console.log(obj2)
obj1[0]['age']=28
console.log(obj1)
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties.ts
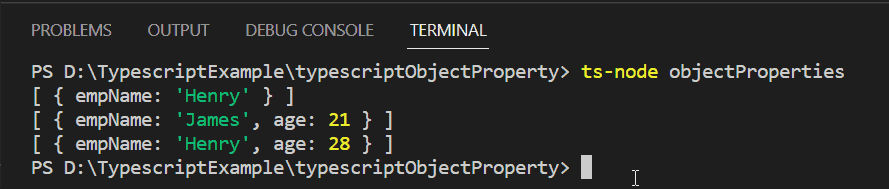
This is an example of Typescript add property to object in array.
Typescript adds a property to object type
Here we will see how to add a property to object type in typescript.
For example, we will create an object type, then based on the condition we will add a property to it in typescript.
In the objectProperties.ts file write the below code:
type PaintOptions = {
shape: string;
xPos?: number;
yPos?: number;
}
function paintShape(ops: PaintOptions) {
let xPos = ops.xPos === undefined ? 'Not defined' : ops.xPos;
let yPos = ops.yPos === undefined ? 'Not defined' : ops.yPos;
const final_result = ops.shape + ', X: ' + xPos + ', Y: ' + yPos
return final_result
}
const Square = {
shape: "Square",
xPos: 10
}
const Rectangle = {
shape: "Rectangle",
xPos: 10,
yPos: 12
}
const result1 = paintShape(Square)
const result2 = paintShape(Rectangle)
console.log(result1)
console.log(result2)
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties.ts
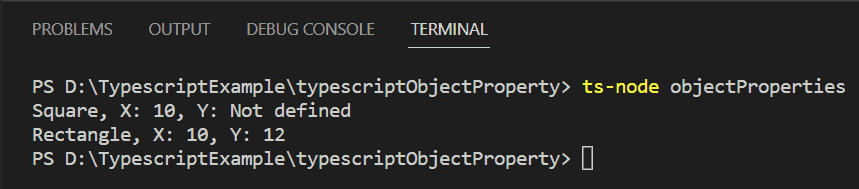
This is an example of Typescript add property to object type.
Read Typescript array concatenation
Typescript adds a property to objects in the map
Here we will see how we can add a property to objects using map() in typescript.
For example, we will map each object in an array, and then we will add the property to objects in typescript.
In the typescript.ts file, write the below code:
type Cat = { name: string; fur: "brown" | "white" };
const addOrigin = (animals: Cat[], origin: "Italy" | "France") =>
animals.map((animal) => ({ ...animal, origin }));
const Cat1: Cat = {
name: "Pussy Cat",
fur: 'white'
}
const Cat2: Cat = {
name: "Pussy Cat",
fur: 'brown'
}
const Cats = [Cat1, Cat2]
console.log(Cat1) //👉 { name: 'Pussy Cat', fur: 'white' }
const result = addOrigin(Cats, "France")
console.log(result) //👉[ { name: 'Pussy Cat', fur: 'white', origin: 'France' },{ name: 'Pussy Cat', fur: 'brown', origin: 'France' }]
const NewCats = [
{ name: "Pussy Cat", fur: 'brown' }
]
console.log(NewCats.map((NewCats) => ({ ...NewCats, origin: "Italy"}))) //👉[ { name: 'Pussy Cat', fur: 'brown', origin: 'Italy' } ]
To compile the below code and run the below command and you can see the result in the console.
ts-node objectProperties.ts
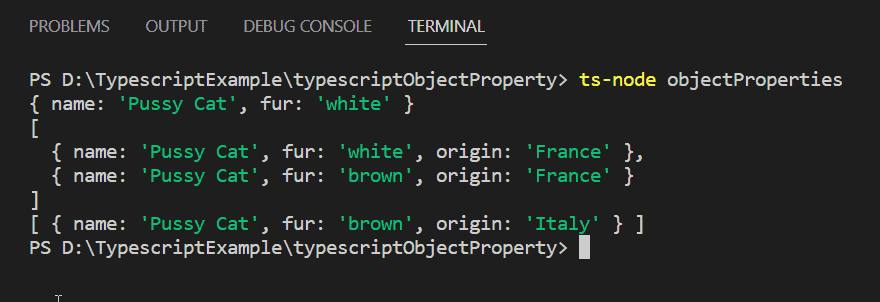
This is an example of Typescript adds a property to objects in the map.
Typescript add property to object if not null
Here we will see an example if the object is not null, then we will add the property to object.
For example, we will create an object, and we will check if the object is not null, then it will add the properties to it.
In the objectProperties.ts file write the below code:
var obj:any = { name: "Alex" };
if(obj !== null){
obj.age =14;
obj['country'] = "UK"
console.log(obj)
}
To compile the code and run the below command, and you can see the result in the console.
ts-node objectProperties.ts

This is an example of Typescript add property to object if not null.
Read How to reverse an array in Typescript
Typescript add property to object spread
Here we will see an example how to add property to object using spread operator in typescript.
For example, we will define an object, then we define a new object, and with the help of spread operator we add the properties of the new object to the original object.
In the objectProperties.ts file, write the below code:
let getAnimals = {
dogs: 3,
cats: 5,
donkeys: 6,
horses: 2,
};
let newAnim = {
goats: 4,
};
const allAnim = {
...getAnimals,
...newAnim,
};
console.log(allAnim)
To compile the code and run the below command, and you can see the result in the console.
ts-node objectProperties.ts

This is an example of Typescript add property to object spread.
Typescript add property to object if exists
Here we will see an example of how to add properties to object if it exists using typescript.
For example, we will define an employee object type, then we will create a function to check object exist or not, if exist, then we will add properties, not, then it will return object does not exist.
In the objectProperties.ts file write the below code:
// Defining a type
type Employee = {
[key: string]: any;
}
// Function to check if object exist
function getObj(obj:Employee){
if(obj){
obj.age =14;
obj['country'] = "UK"
return obj
}
else{
return "Object does not exist"
}
}
// Defining obj of Employee type
var obj:Employee={}
// Defining any type variable
var xyz:any
// passing object in the function
console.log(getObj(obj))
// passing any type variable in the function
console.log(getObj(xyz))
To compile the code and run the below command and you can see the result in the console.
ts-node objectProperties.ts
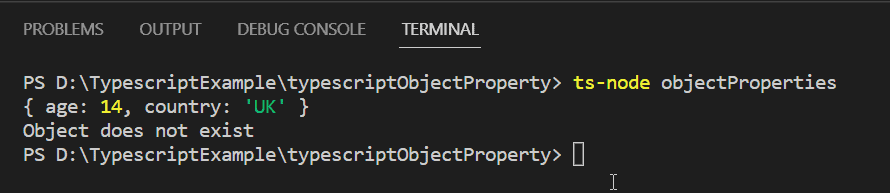
This is an example of Typescript add property to object if exists.
Typescript add property to object without interface
Here we will see how we can add property to object without interface in typescript.
For example, we will first define employee object type using type alias, then we will add properties to it.
In the objectProperties.ts, write the below code:
type Employee = {
[key: string]: any;
}
const obj1: Employee = {
empName: 'Henry',
};
obj1.age = 28;
console.log(obj1);
To compile the code and run the below command, you can see the result in the console.
ts-node objectProperties.ts

This is an example of Typescript add property to object without interface.
Conclusion
In this typescript tutorial, we saw how we can add properties to an object in different scenario. So here are the scenario we covered:
- typescript add a property to object
- typescript add property to object dynamically
- typescript add property to object conditionally
- typescript add property to object in array
- typescript add property to object type
- typescript add property to object if not null
- typescript add property to object spread
- typescript add property to object if exists
- typescript add property to object without interface
- typescript add property to object in map
You may like the following typescript tutorials:
- Typescript array
- Typescript filter array
- Typescript Identifiers and Keywords
- Typescript type annotation
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com