If you want to work in Typescript, you should know about arrays in Typescript. In this tutorial, you will get complete information about the Typescript array.
TypeScript arrays are collections of elements of the same type, offering type safety and reducing runtime errors in web development. You can declare an array using either the syntax let arrayName: type[]; or let arrayName: Array<type>;. Arrays in TypeScript support various operations like adding, accessing, and removing elements and can also be used as multi-dimensional arrays, read-only arrays, or tuples for more complex data structures.
What is a TypeScript Array?
A TypeScript array is a data structure that allows you to store multiple values of the same type together. Unlike JavaScript, TypeScript provides the benefit of defining the type of elements that an array can store, ensuring type safety and reducing runtime errors.
How to declare an array in Typescript?
Now, let us check how to declare an array in Typescript.
You can declare an array in Typescript in two different ways:
- Using Square Brackets:
- Using Generic Array Types:
1. Using Square Brackets:
Here is the syntax to declare an array in Typescript:
let arrayName: type[];
Here is an example of a number array in Typescript.
let numbers: number[] = [1, 2, 3];
Here is another example of a Typescript string array:
let colors: string[] = ["red", "green", "blue"];
2. Using Generic Array Types:
Here is the syntax to declare a Typescript array using generic array types:
let arrayName: Array<type>;
Here is an example of a generic Typescript array.
let values: Array<number> = [1, 2, 3];
Working with Typescript Arrays
Now, let us check how to work with Typescript arrays.
To add an element to an array in Typescript, write the below code:
let colors: string[] = ["red", "green", "blue"];
colors.push("yellow");
To retrieve an element from an array in Typescript, use the below code:
let colors: string[] = ["red", "green", "blue"];
let firstColor = colors[0]; // red
To remove the last element from the array, use the below Typescript code:
let colors: string[] = ["red", "green", "blue"];
colors.pop(); // Removes "blue"
After I ran the code using Visual Studio code, you can see the output below:
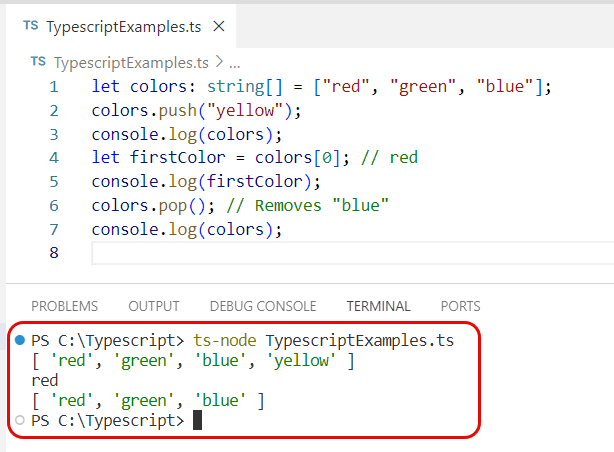
Conclusion
TypeScript arrays are a powerful feature for handling collections of data with type safety. Understanding and using TypeScript arrays effectively can significantly improve the quality and maintainability of your code in web development projects. Remember, TypeScript arrays extend JavaScript arrays, offering strong typing and additional features for robust and error-free coding.
You may also like:
- Typescript sort array
- Typescript array find
- Typescript filter array
- How to get last element of an array in typescript
- How to reverse an array in Typescript
- Typescript sort array by date
- TypeScript Split String Into Array
- How to Loop Through Array in Typescript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com