Typescript provides different ways to loop through an array in Typescript like for loop, for…of loop, foreach loop, etc. In this tutorial, I will explain how to loop through array in Typescript.
Here, I will take different arrays as examples in Typescript, and for each array, I will show you how to iterate over an array in Typescript.
iterate over an array in Typescript
Now, let us check different methods of how to iterate over an array in Typescript.
1. Using for loop
The easiest and simplest way to loop through an array in Typescript is by using the for loop. Here is a simple example:
let fruits: string[] = ['Apple', 'Banana', 'Cherry'];
for (let i = 0; i < fruits.length; i++) {
console.log(fruits[i]);
}
In the example above, we declare an array of strings fruits and use a for loop to log each element to the console. You can see the output in the screenshot below after I executed the code using VS code.
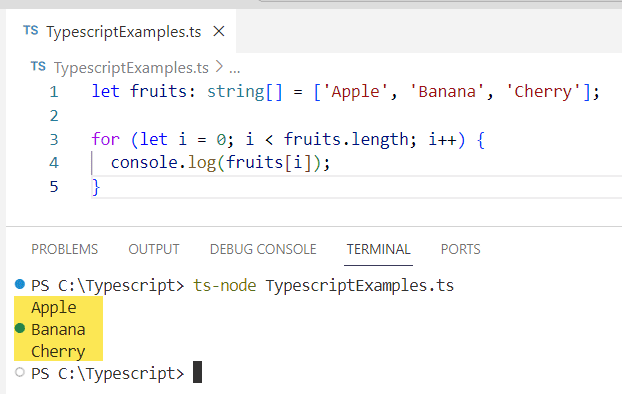
2. Using the for…of Loop
In Typescript, you can also use the the for…of loop to iterate over array elements. It directly retrieves the value of each array element, eliminating the need to use an index variable. Here is the complete code to iterate over an array in Typescript using the for…of loop.
let colors: string[] = ['Red', 'Green', 'Blue'];
for (let color of colors) {
console.log(color);
}
As shown, for…of simplifies the process of looping through the colors array in the above example. You can see the output in the screenshot below:
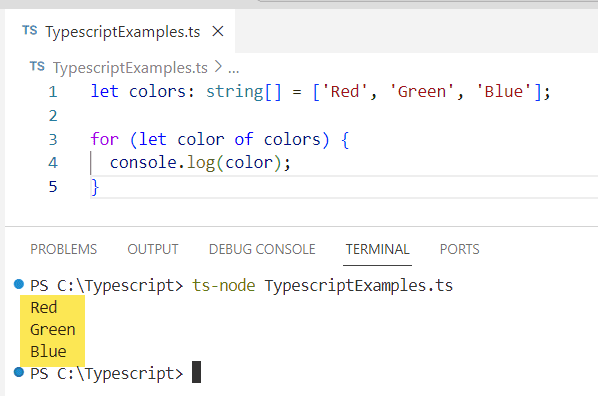
3. Using The forEach Method
You can also use the foreach method in Typescript to loop through array elements. It takes a callback function that is executed for each element in the array.
Here is a complete example of looping through an array using the foreach() method.
let numbers: number[] = [1, 2, 3, 4, 5];
numbers.forEach((number) => {
console.log(number);
});
Here is the output in the screenshot below:
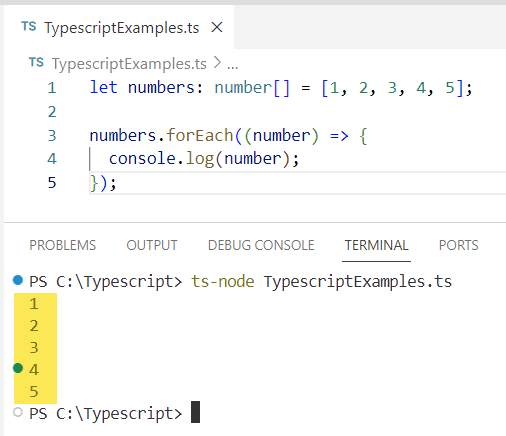
4. Using The for…in Loop
While the for…in loop is primarily used to iterate over the properties of an object, it can also be used to iterate over array elements by their indexes in Typescript. Here is a complete example.
let pets: string[] = ['Dog', 'Cat', 'Rabbit'];
for (let index in pets) {
console.log(pets[index]);
}
You can see the output in the screenshot below:
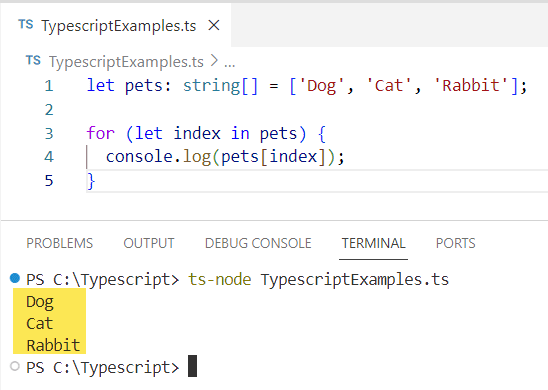
5. Using the map() Method
The map() method in Typescript is similar to forEach, but it creates a new array with the results of calling a provided function on every element in the calling array. The map method is particularly useful when you need to transform the elements of an array and create a new array with the transformed elements. Now, to understand this, follow the below example.
let numbers: number[] = [1, 2, 3, 4, 5];
let squaredNumbers: number[] = numbers.map((number) => number * number);
console.log(squaredNumbers);
Typescript loop through an array with index
Now, let us see another example of how to loop through an array with an index in Typescript.
In TypeScript, if you want to loop through an array with access to both the index and value of each element, you can use the forEach method, which provides the element value and the element index as arguments to the callback function.
Here’s a complete example:
let animals: string[] = ['Lion', 'Tiger', 'Bear'];
animals.forEach((animal, index) => {
console.log(`Index: ${index}, Animal: ${animal}`);
});
In this example, the forEach method is used to iterate over the animals array. For each element in the array, the callback function is executed, which logs the current index and the value of the element (animal) to the console.
The output is in the screenshot below; I executed the code using VS code.
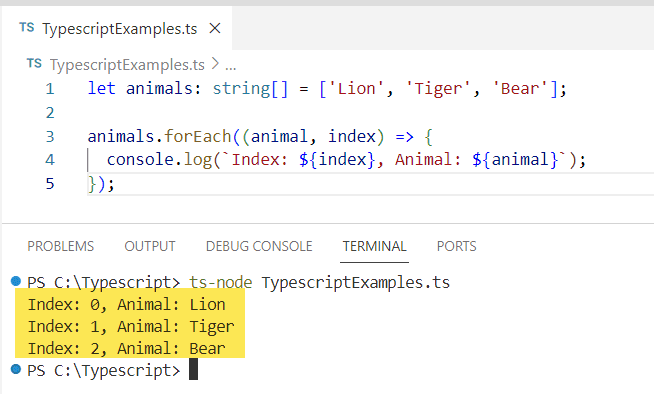
Conclusion
TypeScript provides multiple ways to loop through or iterate over arrays, like the traditional for loop for its control, for…of loop, forEach method, the for…in loop for object-like iteration, or the map method, etc.
In this tutorial, I have explained how to loop through array in Typescript using different approaches. Also, we saw how to loop through array with index in Typescript.
You may also like:
- Typescript merge arrays
- Typescript filter array of objects
- Typescript reverse array
- Typescript sort array of objects by date descending
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com