Do you want to know about the TypeScript Array filter() method? In this Typescript tutorial, I will show you how to use the filter() method in TypeScript to work with arrays. We will also cover typescript filter examples.
What is the filter() Method in Typescript?
The filter()
method in TypeScript allows you to create a new array from an existing one, containing only elements that match a condition you specify. This method does not change the original array but returns a new one.
Syntax
The basic syntax of the Typescript filter()
method is as follows:
let newArray: ArrayType[] = array.filter(callback(element[, index[, array]])[, thisArg]);
callback
: A function that tests each element of the array. Returntrue
to keep the element,false
otherwise.element
: The current element being processed.index
(optional): The index of the current element being processed.array
(optional): The arrayfilter
was called upon.thisArg
(optional): A value to use asthis
when executingcallback
.
Typescript filter examples
Now, let us see how to use filter() in Typescript with a few typescript filter examples.
Example 1: Filter an Array of Numbers
Suppose you have an array of numbers and want to filter out all numbers greater than 10. Here is the complete Typescript filter() method example.
let numbers = [1, 12, 23, 8, 5, 7, 30];
let filteredNumbers = numbers.filter(number => number > 10);
console.log(filteredNumbers);
Output:
[ 12, 23, 30 ]
You can see the output in the screenshot below after I executed the code using VS code.
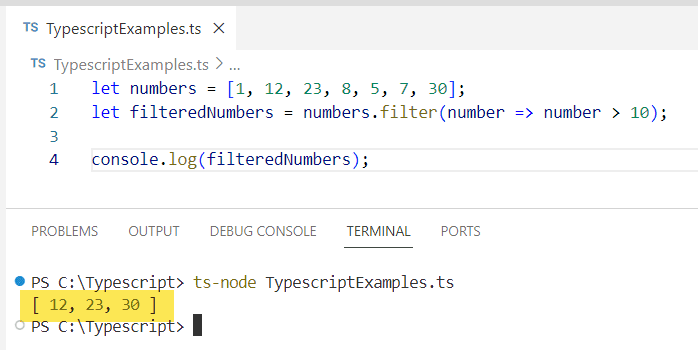
Example 2: Filter an Array of Objects
You can also use the filter() method to filter an array of objects in Typescript.
Suppose you have an array of objects representing books, and you want to find only the books published after the year 2000.
Here is the complete Typescript code.
interface Book {
title: string;
author: string;
year: number;
}
let books: Book[] = [
{ title: 'The Road Ahead', author: 'Bill Gates', year: 1995 },
{ title: 'Harry Potter and the Goblet of Fire', author: 'J.K. Rowling', year: 2000 },
{ title: 'The C Programming Language', author: 'Brian Kernighan & Dennis Ritchie', year: 1978 },
{ title: 'No Logo', author: 'Naomi Klein', year: 1999 },
{ title: 'The Code Book', author: 'Simon Singh', year: 1999 },
{ title: 'The Tipping Point', author: 'Malcolm Gladwell', year: 2000 },
{ title: 'The Da Vinci Code', author: 'Dan Brown', year: 2003 },
];
let recentBooks = books.filter(book => book.year > 2000);
console.log(recentBooks);
Output:
[ { title: 'The Da Vinci Code', author: 'Dan Brown', year: 2003 } ]
You can also see the output in the screenshot below after I executed the code using VS code.
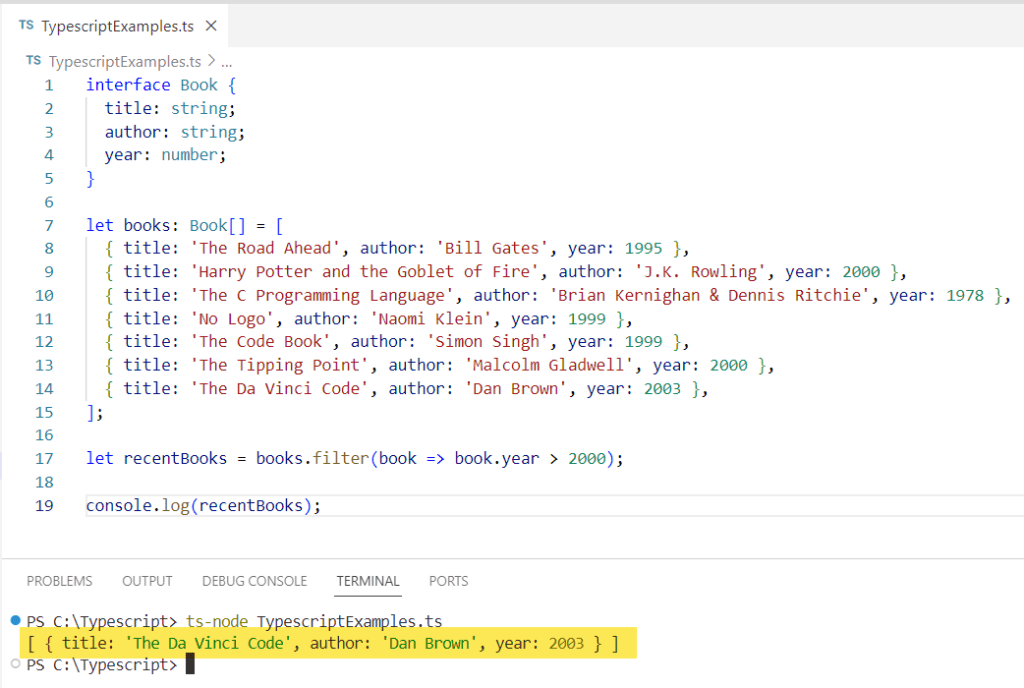
Example 3: Using Type Guards in filter()
TypeScript allows for a concept known as ‘type guards’. A type guard is an expression that performs a runtime check that guarantees the type in some scope. We can use type guards in our filter()
callback to narrow down the type of our array elements.
Here is an example:
type StringOrNumber = string | number;
let mixedArray: StringOrNumber[] = [1, 'apple', 3, 'banana', 5, 'cherry'];
let onlyNumbers: number[] = mixedArray.filter((element): element is number => typeof element === 'number');
console.log(onlyNumbers);
In the above example, the element is number
is a type predicate that ensures only numbers are included in the onlyNumbers
array.
Conclusion
In this tutorial, I have explained how to use the filter() method in Typescript to filter an array with a few examples. The Typescript filter()
does not mutate the original array.
This way, you can filter simple and complex arrays in Typescript using the filter() method.
You may also like:
- How to Loop Through Array in Typescript?
- Merge Object Arrays Without Duplicates in TypeScript
- Typescript merge two arrays of objects
- How to Remove Null Values from an Array in TypeScript?
- TypeScript Array Filter with Multiple Conditions
- How to Filter Duplicates from an Array in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com