I was working with the Typescript array filter() method and wanted to check how to remove null values. In this tutorial, I will explain how to remove null values from an array in Typescript using different methods with examples.
Remove Null Values from an Array in TypeScript using Filter()
To remove null values from an array in Typescript, you can use the filter() method. The filter() method creates a new array filled with elements that pass a test provided by a function. To remove null values, the test will simply check if the element is not null.
Here are a few examples:
Example-1: Basic Filter
In this example, I have an array containing numbers and null values in Typescript. We will remove the null values and keep only the numbers. Below is the complete code:
const arrayWithNulls: (number | null)[] = [1, null, 2, null, 3];
const filteredArray: number[] = arrayWithNulls.filter((item): item is number => item !== null);
console.log(filteredArray);
In the example above, we use a type guard (item): item is number
to inform TypeScript that the filter function will only return an array of numbers, excluding null values.
You can check the screenshot below after I executed the Typescript code using Visual Studio code.
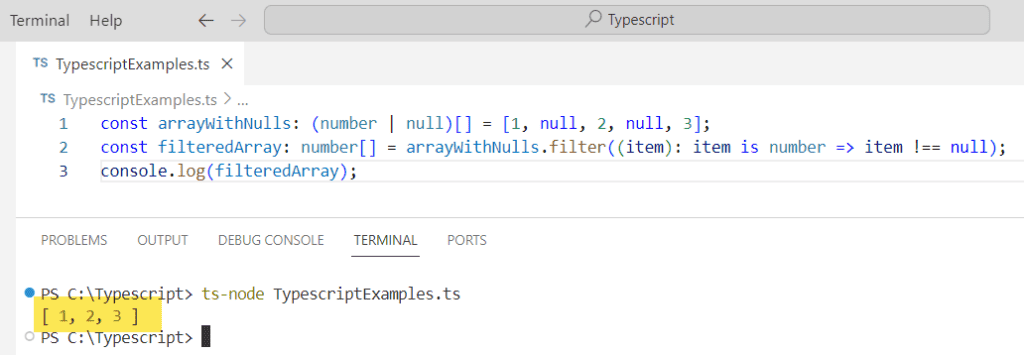
This is the simplest way in Typescript to remove null values.
Here is another example of how to filter out null values from an array of strings in TypeScript. Here is an example:
const stringArrayWithNulls: (string | null)[] = ["apple", null, "banana", "cherry", null];
const filteredStringArray: string[] = stringArrayWithNulls.filter((item): item is string => item !== null);
console.log(filteredStringArray);
In this example, we have an array stringArrayWithNulls
that contains strings mixed with null values. We use the filter
method to create a new array filteredStringArray
that contains only strings. The type guard (item): item is string
tells TypeScript that the new array will not include any null values, ensuring that the variable filteredStringArray
is of type string[]
.
Once you execute the above Typescript code, you can see the output in the screenshot below:
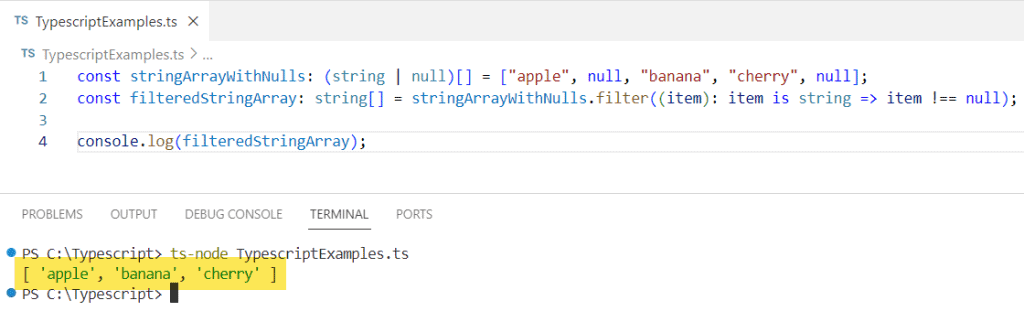
Example-2: Remove null and keep undefined values
Suppose you have a Typescript array that contains both null and undefined values, and you want to remove the null values but keep the undefined values; then you can write the code like below:
const arrayWithNullsAndUndefined: (number | null | undefined)[] = [1, null, undefined, 2, 3];
const filteredArrayKeepingUndefined: (number | undefined)[] = arrayWithNullsAndUndefined.filter((item): item is number | undefined => item !== null);
console.log(filteredArrayKeepingUndefined);
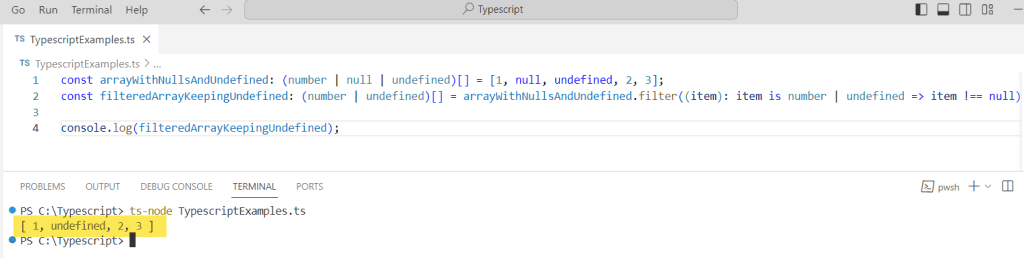
To remove null values from a Typescript array, you can also write a custom function using the filter() method that you can reuse across your application for different types of arrays.
Here is the complete code:
function removeNulls<T>(array: (T | null)[]): T[] {
return array.filter((item): item is T => item !== null);
}
const numbersWithNulls: (number | null)[] = [1, null, 2, null, 3];
const stringsWithNulls: (string | null)[] = ["a", null, "b", "c"];
const numbersWithoutNulls: number[] = removeNulls(numbersWithNulls);
const stringsWithoutNulls: string[] = removeNulls(stringsWithNulls);
console.log(numbersWithoutNulls);
console.log(stringsWithoutNulls);
Remove Null Values from an Array in TypeScript using Exclude Utility Type
There is also another way to remove null values from an array in Typescript, and that is by using the exclude utility type. The exclude utility type allows you to create a type by excluding certain properties from another type.
Here is the complete code:
type NonNullableArray<T> = Exclude<T, null>[];
const arrayWithNulls: (number | null)[] = [1, null, 2, null, 3];
const nonNullableArray: NonNullableArray<number> = arrayWithNulls.filter((item): item is number => item !== null);
console.log(nonNullableArray);
You can refer to the screenshot below, where it removed all the null values from the Typescript array and only returns the numbers.
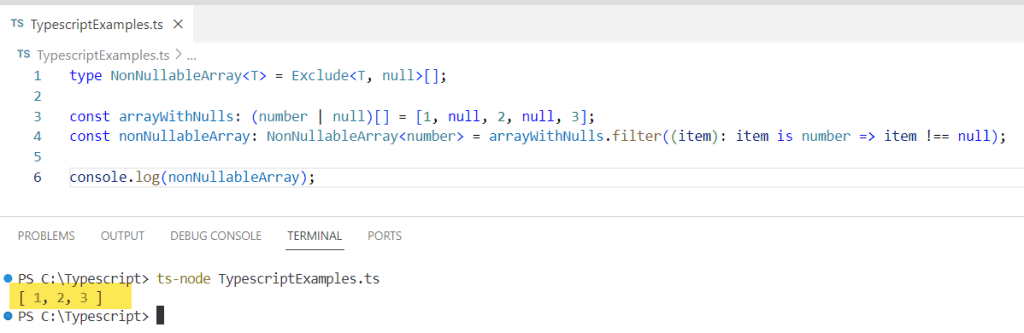
I hope now you have an idea of how to remove null values from an array in Typescript using the filter() method and by using Exclude Utility Type. All these examples I have executed, and the output are shown in the screenshots.
You may also like:
- How to Loop Through Array in Typescript?
- Typescript merge arrays
- Typescript filter array of objects
- TypeScript Array Filter with Multiple Conditions
- How to Filter Duplicates from an Array in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com