While working in a TypeScript application, I was required to filter an array with multiple conditions. In this tutorial, I will show you how to use the filter() method with multiple conditions in TypeScript with complete code and examples.
To filter an array with multiple conditions in TypeScript, you can use the filter method combined with logical operators. For example, to find users from “New York” who are over 30 years old, you can use users.filter(user => user.city === “New York” && user.age > 30).
Filter Array with Multiple Conditions in TypeScript
If you want to extract specific elements from an array that satisfies multiple conditions, we must filter the array with multiple conditions in TypeScript.
I will show you three methods for filtering an array with multiple methods in TypeScript. Follow the examples below.
Method 1: Using filter with Logical AND (&&)
The best method to filter an array with multiple conditions in TypeScript is to use the filter
method combined with logical operators. Here’s an example:
Suppose we have an array of user objects, and we want to filter users who are from “New York” and are over 30 years old.
interface User {
name: string;
age: number;
city: string;
}
const users: User[] = [
{ name: "John Doe", age: 28, city: "New York" },
{ name: "Jane Smith", age: 35, city: "Los Angeles" },
{ name: "Mike Johnson", age: 32, city: "New York" },
{ name: "Emily Davis", age: 22, city: "Chicago" },
{ name: "Sarah Brown", age: 40, city: "New York" }
];
const filteredUsers = users.filter(user => user.city === "New York" && user.age > 30);
console.log(filteredUsers);
In this example, the filter
method iterates over each user object and applies the conditions user.city === "New York"
and user.age > 30
. The result is an array of users who meet both criteria like below:
[
{ name: 'Mike Johnson', age: 32, city: 'New York' },
{ name: 'Sarah Brown', age: 40, city: 'New York' }
]
You can even look at the screenshot below after I executed the above code using VS code.
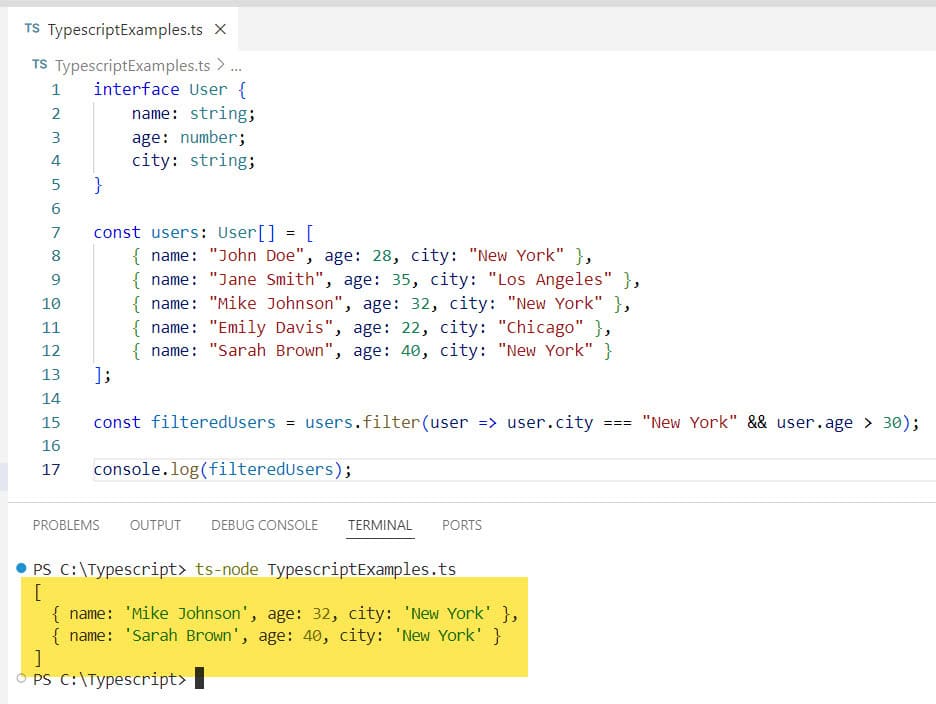
Method 2: Using filter with Logical OR (||
)
Sometimes, you may want to filter a TypeScript array where at least one of multiple conditions is met. For this, you can use the logical OR operator (||
).
Let’s filter users who are either from “New York” or are over 30 years old. Here is the example with the complete code.
interface User {
name: string;
age: number;
city: string;
}
const users: User[] = [
{ name: "John Doe", age: 28, city: "New York" },
{ name: "Jane Smith", age: 35, city: "Los Angeles" },
{ name: "Mike Johnson", age: 32, city: "New York" },
{ name: "Emily Davis", age: 22, city: "Chicago" },
{ name: "Sarah Brown", age: 40, city: "New York" }
];
const filteredUsersOr = users.filter(user => user.city === "New York" || user.age > 30);
console.log(filteredUsersOr);
Here, the filter
method returns users who either live in “New York” or are older than 30, like below
[
{ name: 'John Doe', age: 28, city: 'New York' },
{ name: 'Jane Smith', age: 35, city: 'Los Angeles' },
{ name: 'Mike Johnson', age: 32, city: 'New York' },
{ name: 'Sarah Brown', age: 40, city: 'New York' }
]
You can even check the screenshot below for your reference.
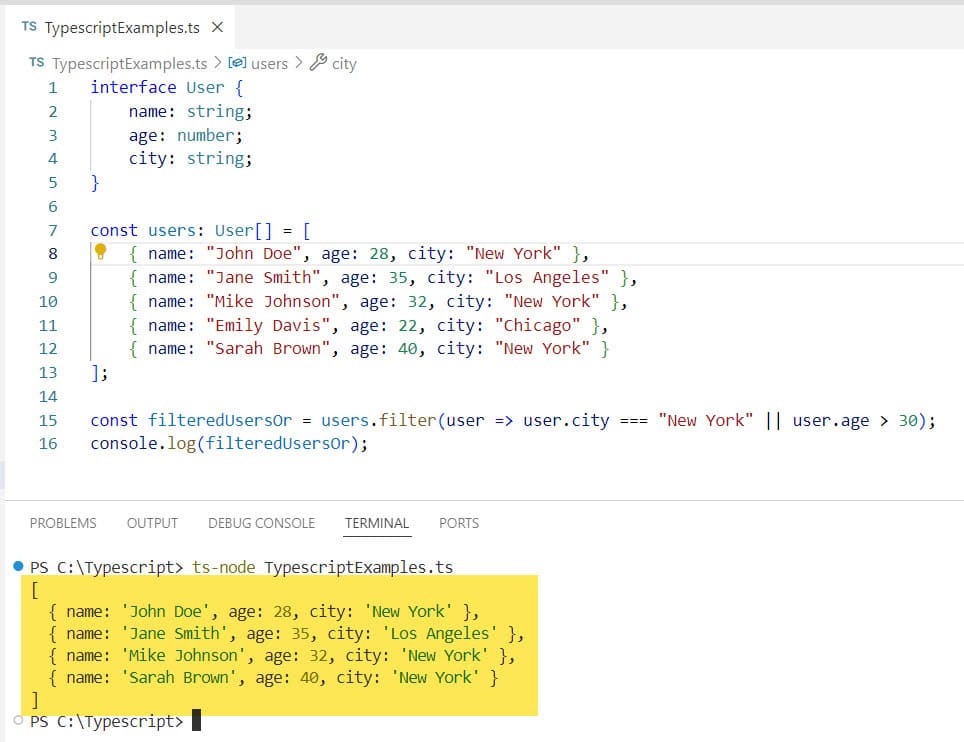
Method 3: Combine Multiple Conditions Dynamically
Sometimes, multiple conditions need to be combined dynamically. So, for this, we will create a function that evaluates all the conditions.
Suppose we want to filter users based on dynamic conditions stored in an array.
type Condition<T> = (item: T) => boolean;
function filterArray<T>(array: T[], conditions: Condition<T>[]): T[] {
return array.filter(item => conditions.every(condition => condition(item)));
}
const conditions: Condition<User>[] = [
user => user.city === "New York",
user => user.age > 30
];
const dynamicFilteredUsers = filterArray(users, conditions);
console.log(dynamicFilteredUsers);
In this example, we define a Condition
type and a filterArray
function that accepts an array and an array of conditions. The every
method checks if all conditions are met for each item in the array.
This is how to filter an array in Typescript with multiple conditions.
You may also like:
- How to Remove Null Values from an Array in TypeScript?
- How to Loop Through Array in Typescript?
- How to Split String By New Line in Typescript?
- How to Filter Duplicates from an Array in TypeScript?
- How to Filter Empty Strings from an Array in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com