In this Typescript tutorial, I have explained how to filter duplicates from an array in Typescript. There are different methods to filter duplicates from a Typescript array.
TypeScript Array Filter Duplicates
There are various methods to filter duplicates from an array in Typescript.
Using Set
One of the simplest ways to remove duplicates from an array in TypeScript is by using the Set
object. A Set
is a collection of values where each value must be unique.
function removeDuplicatesUsingSet<T>(array: T[]): T[] {
return Array.from(new Set(array));
}
// Example usage:
const numbers = [1, 2, 2, 3, 4, 4, 5];
const uniqueNumbers = removeDuplicatesUsingSet(numbers);
console.log(uniqueNumbers);
I executed the above Typescript code using VS code, and you can see the output below:
[ 1, 2, 3, 4, 5 ]
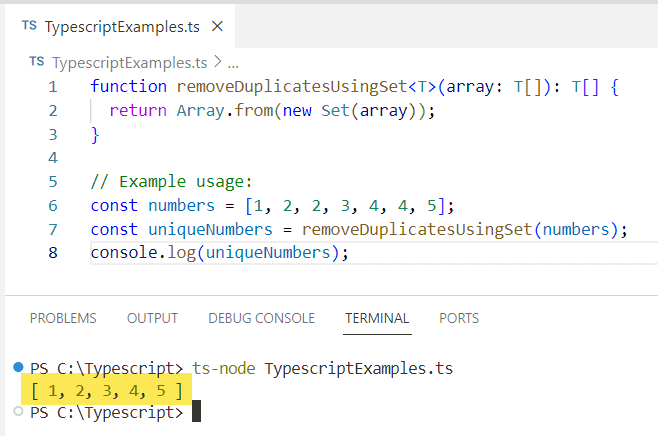
Using Filter and IndexOf
Another way to filter out duplicates from a Typescript array is to use the filter() method in combination with the indexOf
method. This method checks if the current item’s index is the first occurrence in the array.
Here is a complete code:
function removeDuplicatesUsingFilter<T>(array: T[]): T[] {
return array.filter((value, index, self) => self.indexOf(value) === index);
}
// Example usage:
const chars = ['a', 'b', 'a', 'c', 'b'];
const uniqueChars = removeDuplicatesUsingFilter(chars);
console.log(uniqueChars);
Using Reduce
The reduce() method can also be used to remove duplicates by accumulating unique items into a new array in Typescript.
function removeDuplicatesUsingReduce<T>(array: T[]): T[] {
return array.reduce((accumulator, currentValue) => {
if (!accumulator.includes(currentValue)) {
accumulator.push(currentValue);
}
return accumulator;
}, [] as T[]);
}
// Example usage:
const pets = ['dog', 'cat', 'bird', 'cat', 'dog', 'fish'];
const uniquePets = removeDuplicatesUsingReduce(pets);
console.log(uniquePets);
Here is the output in the screenshot below after executing the above Typescript code using VS code.
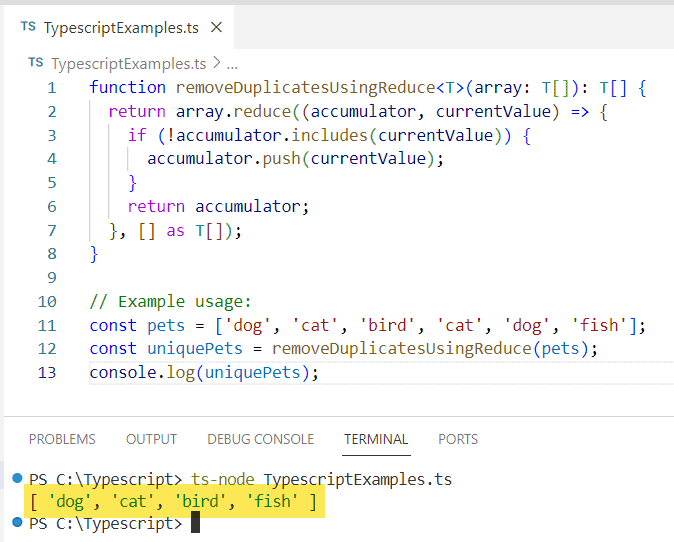
Filter Duplicate Objects
Removing duplicates becomes slightly more complex when dealing with arrays of objects in Typescript. We need to compare object properties to determine uniqueness.
interface Product {
id: number;
name: string;
}
function removeDuplicateObjects(products: Product[]): Product[] {
return products.filter((product, index, self) =>
index === self.findIndex((t) => t.id === product.id)
);
}
// Example usage:
const products: Product[] = [
{ id: 1, name: 'Book' },
{ id: 2, name: 'Pen' },
{ id: 1, name: 'Book' },
{ id: 3, name: 'Pencil' },
];
const uniqueProducts = removeDuplicateObjects(products);
console.log(uniqueProducts);
This method utilizes filter
and findIndex
to ensure that only the first occurrence of each object (based on a unique property, like id
) is included in the resulting array.
I hope now you have an idea of how to filter duplicates from an array in Typescript. Here, I have explained various methods to remove duplicates from a Typescript array like Set, filter with index of, reduce, etc.
You may also like:
- TypeScript Array Filter with Multiple Conditions
- Remove Null Values from an Array in TypeScript
- TypeScript Array filter() Method
- How to Merge Object Arrays Without Duplicates in TypeScript?
- How to Filter Empty Strings from an Array in TypeScript?
I am Bijay a Microsoft MVP (10 times – My MVP Profile) in SharePoint and have more than 17 years of expertise in SharePoint Online Office 365, SharePoint subscription edition, and SharePoint 2019/2016/2013. Currently working in my own venture TSInfo Technologies a SharePoint development, consulting, and training company. I also run the popular SharePoint website EnjoySharePoint.com